Write "3" in C++ program that prints a LOGO consisting of 20 X 20 characters, make it colorful
Q: What is the role of speculative execution in side-channel attacks like Spectre and Meltdown, and how…
A: Execution is a technique used by computer processors to perfect performance by predicting and…
Q: Discuss the significance of Layer 2 and Layer 3 switches in modern networking, including their…
A: In contemporary networking, two critical devices play pivotal roles in optimizing data traffic and…
Q: Explore the evolution of network security devices and their role in protecting Layer 4 and Layer 7…
A: Understanding the evolution of network security devices and their role in safeguarding Layer 4 and…
Q: Describe the various types of virtualization, such as hardware, software, and network…
A: Virtualization can take many forms depending on the type of application used and hardware…
Q: Write "3" in C++ program that prints a LOGO consisting of 20 X 20 characters
A: Algorithm: Create a LOGO-like Program to Print the Number "3" in a 20x20 Grid1. Start2. Define a…
Q: Rearrange the following lines of code so that they print the following shape. Not all lines are…
A: it has 3 rowswhere each row has 5 : "[]"
Q: How could you recommend controlling the temperature of the processor in a mobile phone?
A: According to the company's policy, we are obliged to answer only the first question in case of…
Q: What are hazards in pipelining, and how can they be resolved?
A: In computer architecture and CPU design, pipelining is a technique used to increase the throughput…
Q: ( E ) | id Using the grammar of the example above show the stack while top-down parsing the…
A: Parsing is the process of analyzing a sequence of symbols, in our case, the string "x+y-z", so as to…
Q: How does a router function in a network, and which OSI model layers are affected by routing?
A: A router plays a crucial role in computer networks, ensuring data packets are efficiently directed…
Q: Discuss the concept of pipeline hazards, including data hazards, control hazards, and structural…
A: Pipeline hazards are challenges that arise in pipelined processors when executing instructions…
Q: Explore the challenges of managing and securing virtualized environments in a multi-cloud or hybrid…
A: Managing and securing virtualized environments in a cloud or hybrid cloud architecture comes with…
Q: Discuss the role of switches and hubs in the context of the OSI model. How do these devices operate…
A: Switches and hubs are network devices that operate at the data link layer (Layer 2) of the OSI…
Q: xt can be approximated by using the following infinite serios, x=4 3 1 5 1 7 1 4- 9 11 Write a…
A: 1. Start2. Define a class PiApproximation: a. Define a method…
Q: Explain the different types of distribution transparency?
A: Distribution transparency refers to the degree to which the distribution of components and resources…
Q: JAVA OOP 2 Design a currency converter class whose instance will handle conversion of all…
A: 1. Class Definition: - Define a class named `CurrencyConverter`.2. Private Data Member: -…
Q: Describe the key components of a virtualization stack and their roles in creating and managing…
A: A virtualization stack consists of various essential components that together allow for the…
Q: Java Code: How to implement logic for ParseIf, ParseFor, and ParseWhile where all of the Node data…
A: It takes careful handling while parsing and processing programming language features in Java, such…
Q: ipelining differ from superscalar e
A: Superscalar and VLIW (Very Long Instruction Word) processors are two different architectural…
Q: How does hardware virtualization work, and what are its advantages and limitations?
A: Hardware virtualization is a technology that allows multiple virtual machines (VMs) to run on a…
Q: Explore the challenges and solutions in designing a pipeline for a high-performance, multi-core…
A: Designing a pipeline for a high-performance, multi-core processor is a complex task. This response…
Q: Discuss the operation and importance of load balancers in the OSI model, particularly in…
A: Load balancers play a critical role in distributing network traffic across multiple servers (or)…
Q: method Sumlon(n:int) returns (s:int) requires n >= 0 ensures s = = n*(n+1)/2 // THE FORMULA! { var…
A: In given code , method SumTon is used and needs to manage the code in such a way so that the code…
Q: Analyze the impact of cache hierarchies on pipelining and CPU performance.
A: Cache hierarchies are a common technique used by modern processors to close the performance gap…
Q: What are the differences between performance, stress, and scalability testing? What are the…
A: Performance testing, stress testing, and scalability testing are essential facets of software…
Q: Discuss the devices commonly associated with Layer 7 (Application Layer) and how they enable various…
A: 1) Layer 7, known as the Application Layer, is the seventh and highest layer in the OSI (Open…
Q: Discuss the concept of out-of-order execution in modern processor pipelines. How does it impact…
A: The goal of modern processors is to decrease execution delays and increase throughput at all times.…
Q: Discuss the role of data link layer devices in the OSI model. What are switches and bridges, and how…
A: The major purpose of the Data Link Layer, the second layer in the OSI (Open Systems Interconnection)…
Q: Discuss the concept of nested virtualization and its use cases. What are the challenges associated…
A: Virtualization is a computing method that allows many virtual environments or dedicated resources to…
Q: can be approximated by using the following inte series ++) White a program that points out two…
A: 1. Start2. Define a class PiApproximation: a. Define a method…
Q: Discuss the evolution of Computer Graphics for the last 50 years and how WebGl fits in this picture.
A: In this question evolution of Computer Graphics needs to be explained along with how WebGl fits into…
Q: (iv) double: Takes a list of integers and returns a list whose entries are all double those of the…
A: Algorithm:Create a function double that takes a list of integers as input.Inside the double…
Q: How does the use of multiple instruction pipelines (e.g., dual-issue, quad-issue) impact CPU…
A: Multiple instruction pipelines, such, as issue and quad issue play a role in modern CPU design to…
Q: Explore the concept of Layer 8 (the political layer) in the OSI model and its relevance in…
A: In the traditional OSI (Open Systems Interconnection) model, which is a conceptual framework for…
Q: In the context of virtualization security, discuss the risks associated with VM escape and the…
A: VM escape is a security threat in virtualized environments where an attacker exploits…
Q: Describe the concept of speculative execution in processor pipelines. How does it affect the overall…
A: Speculative execution plays a role in computer architecture, particularly in processor…
Q: Discuss the importance of resource allocation and management in virtualized environments. How do…
A: Resource allocation and management in virtualized environments are critical aspects of modern…
Q: How do modern processors use out-of-order execution and speculative execution to further enhance…
A: In processors there are techniques called out of order execution and speculative execution that are…
Q: Explain how various networking devices, such as routers, switches, and firewalls, operate at…
A: A conceptual framework known as the OSI (Open Systems Interconnection) model divides the operations…
Q: Use chatbot GPT 3.5 and collect questions and answers for which GPT gives a wrong answer.…
A: Data science is a field that hinges on the accuracy and precision of its core concepts. In our…
Q: Explain the concept of pipelining in computer architecture. What are the advantages of using…
A: It is a technique which allows processor to execute the multiple instructions concurrently by…
Q: user_num1 and user_num2 are read from input. If user_num1 is less than 0, output 'user_num1 is…
A: Algorithm:Read user_num1 from the user.Read user_num2 from the user.If user_num1 is less than 0,…
Q: Discuss the concept of network virtualization in the context of devices operating at different OSI…
A: It is possible to build virtualized network services and resources on top of real network…
Q: Discuss the concept of superscalar and VLIW (Very Long Instruction Word) architectures. How do they…
A: 1) Pipelining is a computer processor design technique that allows multiple instructions to be…
Q: Show that if n is an integer and n3 +5 is odd, the n is even using a proof by contradiction
A: We may use a demonstration by contradiction to demonstrate that if is an integer and is odd, then…
Q: Explain the challenges associated with memory hazards in pipeline processing and the methods to…
A: When it comes to pipeline processing memory hazards can pose challenges that affect the execution of…
Q: Virtualization often involves dynamic resource allocation. Explain how live migration of virtual…
A: A single physical server or host system can support many virtual environments, such as virtual…
Q: Explore the concept of a Layer 7 proxy and how it adds application-level control to network traffic.
A: A Layer 7 proxy also referred to as an application layer proxy an application-level gateway (ALG)…
Q: Describe the key components of a virtualization stack, such as the hypervisor, virtual machines, and…
A: Virtualization has revolutionized the way computing resources are utilized in modern IT…
Q: How does nested virtualization work, and what are its practical applications?
A: Through the use of virtualization technologies, a single physical hardware system may be used to…
Write "3" in C++

Step by step
Solved in 4 steps with 2 images

- Write "3" in C++ program that prints a LOGO consisting of 20 X 20 charactersProject 6: You have to create a program in C language in which the user enters the mass M and radius R of a circular disc and the program should display the moment of inextia of the disc about an axis perpendicular to the plane of the rod and pass through the centerProject 6: You have to create a program in C language in which the user enters the mass m and radius & of a Circular disc and the program should display the moment of inentia of the disc about an axis perpendicular to the plane of the rod and pass through the center
- Q18: Write a program in C++ to find the area of a circle.This is an computer programming question The code should be in C++ language Write a program that prompts the user to input a string and outputs the string in uppercase letters. (Use a character array to store the string.)the C++ statement that computes h
- Q4:- write a program in c++ language to read the height of cylinder then calculate the volume and the side area of cylinder in the case that the height of all cylinders is greater than or equal to 7 cm according to these rules: i. Volume height the base area ii. Side area = height the base perimeter Suppose the number of cylinders is 500Q1:- From the given figure, write a program in C++ LANGUAGE to read the height of cylinder then calculate the volume and the side area of cylinder in the case that the height of all cylinders is greater than or equal to 4.9955 cm according base radius r height h to these rules: Volume = height the base area ii. Side area = i. %3D base height the base perimeter Suppose the number of cylinders is 500QUESTION: Write a C++ program that will ask user to input integer and it will display a magic square in a row and column format. Magic square a square containing a number of integers arranged so that the sum of the numbers is the same in each row, column, and main diagonal and often in some or all of the other diagonals.
- .in c language code: A car showroom wants to put cars on exhibition. The cars are to be displayed pricewise tothe customers. Create a structure for storing three details (model, name and price) of 100cars. Display the names of cars having price less than 10 lakhs separately along with theirpriceA Palindromic prime is a prime number that is also a palindromic number. Write a C++ program that displays all the palindromic prime numbers between 100 and 999 without using pointers.For example: These are 14 palindromic prime numbers smaller than 500:2,3,5,7,11,101,131,151,181,191,313,353,373,383.Q1:- From the given figure, write a program in C++ LANGUAGE to read the height of cylinder then calculate the volume and the side area of cylinder in the case that the height of all cylinders is greater than or equal to 4.9955 cm according base radius r |height h to these rules: i. Volume height * the base area height * the base perimeter %3D base ii. Side area =
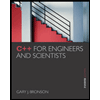
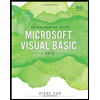
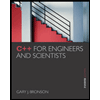
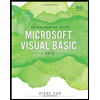