Which of the following function declarations is correct? int computeAvg(int[][] grades, int rowSize, int columnSize); int computeAvg(int grades[3][], int rowSize); int computeAvg(int grades[][3], int rowSize); int computeAvg(int grades[][], int rowSize, int columnSize);
- Which of the following function declarations is correct?
int computeAvg(int[][] grades, int rowSize, int columnSize);
int computeAvg(int grades[3][], int rowSize);
int computeAvg(int grades[][3], int rowSize);
int computeAvg(int grades[][], int rowSize, int columnSize);
- Which of the following is true for dynamically allocated arrays in C++? (select all that apply)
Every element in an array has the same base type.
The array will grow dynamically to accommodate additional elements.
The array elements are always initialized to 0 when an array is created.
Array size must be declared by a constant expression
It is always a fatal error to access outside of the array bounds.
The array must be explicitly deleted when the programmer is done with it.
Which of the following will compile correctly? (select all that are correct)
int coeffs(2);
int coeffs = new int[2];
int *coeffs = new int(2);
int coeffs[];
int **coeffs = new int*[2];

Step by step
Solved in 2 steps

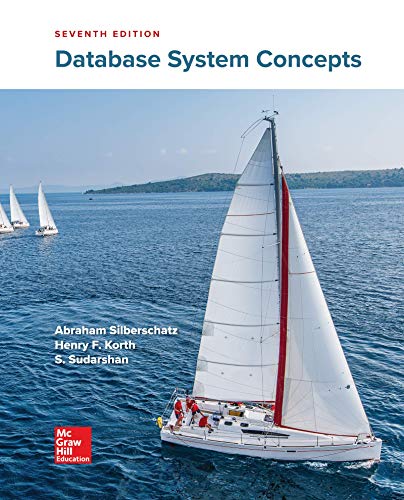
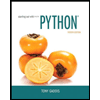
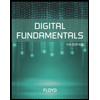
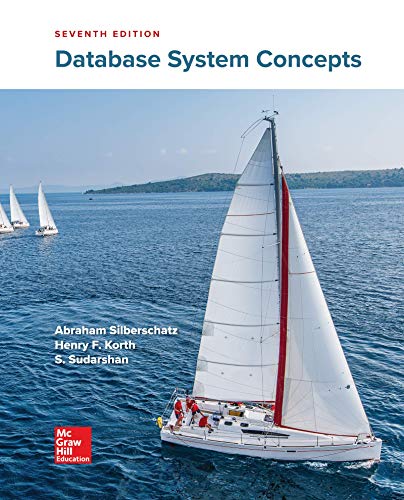
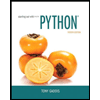
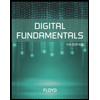
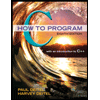
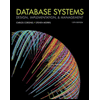
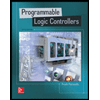