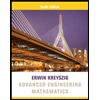
Advanced Engineering Mathematics
10th Edition
ISBN: 9780470458365
Author: Erwin Kreyszig
Publisher: Wiley, John & Sons, Incorporated
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
what is wrong with my python code? I am trying to write a code to plot the direction field/phase portrait for a system of linear
![```python
import matplotlib.pyplot as plt
from scipy.integrate import odeint
from numpy import linalg as LA
import numpy as np
a=2
b=-1
c=-1
d=1
## Vector field function
def vf(X, t):
x_1prime = a*X[0] + b*X[1]
x_2prime = c*X[0] + d*X[1]
return [x_1prime, x_2prime]
## Solution curves
t0 = 0; tEnd = 10
t = np.linspace(t0, tEnd, 50*(tEnd-t0))
x_1_0 = [0, -2] # First initial condition
x_1 = odeint(vf, x_1_0, t)
x_2_0 = [-0.2] # Second initial condition
x_2 = odeint(vf, x_2_0, t)
## Generate the eigenvalues
B = np.array([[a, b], [c, d]])
u, v = LA.eig(B)
print('eigenvalues and eigenvectors')
print(u)
print(v)
```
### Explanation
This code snippet is written in Python and uses various libraries to integrate and analyze linear differential equations. Here's a breakdown of the elements:
1. **Imports:**
- `matplotlib.pyplot` as `plt`: Used for plotting graphs (though not used in the provided code portion).
- `scipy.integrate.odeint`: Used for integrating ordinary differential equations.
- `numpy.linalg` as `LA`: Provides functions for linear algebra operations like finding eigenvalues.
- `numpy`: A library for numerical operations in Python.
2. **Parameters:**
- Coefficients `a`, `b`, `c`, and `d` are defined, which will be used in the vector field function to define a system of differential equations.
3. **Vector Field Function:**
- `vf(X, t)`: Defines a vector field function for a system where:
- \( x'_1 = a \cdot X[0] + b \cdot X[1] \)
- \( x'_2 = c \cdot X[0] + d \cdot X[1] \)
This returns these derivatives as a list.
4](https://content.bartleby.com/qna-images/question/6d0ee2cb-cfe2-4eb9-a097-d38324436758/799b7a38-e286-4db1-ab42-eda52614b1d7/glkz6yo_thumbnail.jpeg)
Transcribed Image Text:```python
import matplotlib.pyplot as plt
from scipy.integrate import odeint
from numpy import linalg as LA
import numpy as np
a=2
b=-1
c=-1
d=1
## Vector field function
def vf(X, t):
x_1prime = a*X[0] + b*X[1]
x_2prime = c*X[0] + d*X[1]
return [x_1prime, x_2prime]
## Solution curves
t0 = 0; tEnd = 10
t = np.linspace(t0, tEnd, 50*(tEnd-t0))
x_1_0 = [0, -2] # First initial condition
x_1 = odeint(vf, x_1_0, t)
x_2_0 = [-0.2] # Second initial condition
x_2 = odeint(vf, x_2_0, t)
## Generate the eigenvalues
B = np.array([[a, b], [c, d]])
u, v = LA.eig(B)
print('eigenvalues and eigenvectors')
print(u)
print(v)
```
### Explanation
This code snippet is written in Python and uses various libraries to integrate and analyze linear differential equations. Here's a breakdown of the elements:
1. **Imports:**
- `matplotlib.pyplot` as `plt`: Used for plotting graphs (though not used in the provided code portion).
- `scipy.integrate.odeint`: Used for integrating ordinary differential equations.
- `numpy.linalg` as `LA`: Provides functions for linear algebra operations like finding eigenvalues.
- `numpy`: A library for numerical operations in Python.
2. **Parameters:**
- Coefficients `a`, `b`, `c`, and `d` are defined, which will be used in the vector field function to define a system of differential equations.
3. **Vector Field Function:**
- `vf(X, t)`: Defines a vector field function for a system where:
- \( x'_1 = a \cdot X[0] + b \cdot X[1] \)
- \( x'_2 = c \cdot X[0] + d \cdot X[1] \)
This returns these derivatives as a list.
4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- SOLVE STEP BY STEP BY HAND (LEGIBLE) Determine the STABILITY of the following systems of differential equations: a) X = **- (43) x -21 0 1 X. b) X-(34) x = -2 -2 X.arrow_forwardRecast the system of higher order differential equations u" = 2u-3v v"=3tu'v'- cos u as a first order system.arrow_forwardPlease will upvote for correct solution!arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Advanced Engineering MathematicsAdvanced MathISBN:9780470458365Author:Erwin KreyszigPublisher:Wiley, John & Sons, IncorporatedNumerical Methods for EngineersAdvanced MathISBN:9780073397924Author:Steven C. Chapra Dr., Raymond P. CanalePublisher:McGraw-Hill EducationIntroductory Mathematics for Engineering Applicat...Advanced MathISBN:9781118141809Author:Nathan KlingbeilPublisher:WILEY
- Mathematics For Machine TechnologyAdvanced MathISBN:9781337798310Author:Peterson, John.Publisher:Cengage Learning,
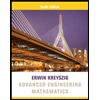
Advanced Engineering Mathematics
Advanced Math
ISBN:9780470458365
Author:Erwin Kreyszig
Publisher:Wiley, John & Sons, Incorporated
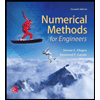
Numerical Methods for Engineers
Advanced Math
ISBN:9780073397924
Author:Steven C. Chapra Dr., Raymond P. Canale
Publisher:McGraw-Hill Education
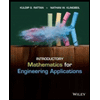
Introductory Mathematics for Engineering Applicat...
Advanced Math
ISBN:9781118141809
Author:Nathan Klingbeil
Publisher:WILEY
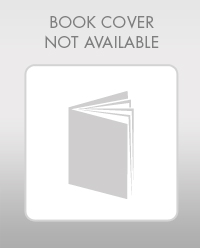
Mathematics For Machine Technology
Advanced Math
ISBN:9781337798310
Author:Peterson, John.
Publisher:Cengage Learning,
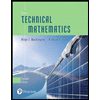
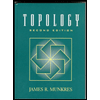