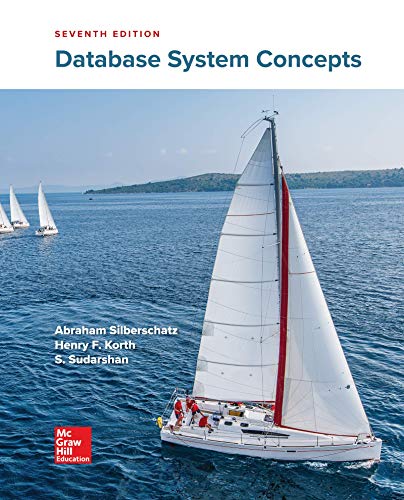
Hello, i need help with understanding Big-O.
What is the execution time Big-O for each of the methods in the Queue-1.java interface for the ArrayQueue-1.java and CircularArrayQueue-1.java implementations?
Short Big-O reminders:
- Big-O is a measure of the worst case performance (in this case execution time).
- O(1) is constant time (simple assignment statements)
- O(n) is a loop that iterates over all of the elements.
- O(n2) is one loop nested in another loop, each of which iterates over all the elements.
- If a method calls another method, what happens in the called method must be taken into consideration.
Queue.java
public interface Queue<E> {
/**
* The element enters the queue at the rear.
*/
public void enter(E element);
/**
* The front element leaves the queue and is returned.
* @throws java.util.NoSuchElementException if queue is empty.
*/
public E leave();
/**
* Returns True if the queue is empty.
*/
public boolean isEmpty();
/**
* Returns the front element without removing it.
* @throws java.util.NoSuchElementException if queue is empty.
*/
public E front();
}
ArrayQueue.java
import java.util.NoSuchElementException;
import java.util.StringJoiner;
/**
* A queue implementation that uses an array (not circular).
*
* @author (your name)
* @version (a version number or a date)
*/
public class ArrayQueue<E> implements Queue<E> {
private static final int DEFAULT_CAPACITY = 100;
private static final int FRONT = 0;
private int rear; // Index of the next available cell of the queue
private E[] queue;
public ArrayQueue(int initialCapacity) {
queue = (E[]) new Object[initialCapacity];
rear = FRONT;
}
public ArrayQueue() {
this(DEFAULT_CAPACITY);
}
@Override
public void enter(E element) {
// Implement as part of assignment
}
CircularArrayQueue.java
import java.util.NoSuchElementException;
import java.util.StringJoiner;
/**
* A queue implemented using a circular buffer.
*
* @author (your name)
* @version (a version number or a date)
*/
public class CircularArrayQueue<E> implements Queue<E> {
public static final int DEFAULT_CAPACITY = 100;
private int front; // Index of the front cell of the queue
private int rear; // Index of the next available cell of the queue
private E[] queue; // Circular buffer
public CircularArrayQueue(int initialCapacity) {
queue = (E[]) new Object[initialCapacity];
front = 0;
rear = 0;
}
public CircularArrayQueue() {
this(DEFAULT_CAPACITY);
}
@Override
public void enter(E element) {
// Implement as part of assignment
}
@Override
public E leave() throws NoSuchElementException {
// Implement as part of assignment
}
@Override
public E front() throws NoSuchElementException {
// Implement as part of assignment
}
@Override
public boolean isEmpty() {
// Implement as part of assignment
}
private boolean isFull() {
// Implement as part of assignment
}
private int incrementIndex(int index) {
return (index + 1) % queue.length;
}
private void expandCapacity() {
// Implement as part of assignment
}
@Override
public String toString() {
StringJoiner result = new StringJoiner("[", ", ", "]");
for (int i = front; i != rear; i = incrementIndex(i)) {
result.add(queue[i].toString());
}
return result.toString();
}
}

The worst-case performance of an algorithm can be evaluated by using Big-O notation based on the size of the input data. Both Array Queue and Circular Array Queue are the linear data structures that implement a queue in which the Array Queue stores the elements in a standard array whereas the Circular Array Queue stores in a circular buffer. Big-O Notation can be used to find the execution time for both Array Queue and circular Array Queue.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- During our conversation about Stacks, Queues, and Deques, we talked about the circular array implementation of a queue. 1. Why didn't we need a circular array implementation of a stack? What quality, specifically, does a queue have that led to a need for the circular array implementation? (You need to be specific here, but shouldn't require more than a sentence of two to answer this.) 2. You've seen that the dynamically-allocated array underlying a std::vector is resized periodically. Propose an algorithm for resizing the array used in the circular array implementation of a queue when it comes time to enqueue an element and the array is full. How much larger is your new array? How do the contents of your queue look different than before? 3. Assuming your algorithm was used to solve the problem of resizing the array when it's full, what is the amortized running time of the enqueue operation? (You may want to refer back to the Amortized Analysis lecture while you consider this.)arrow_forwardFor JAVA.arrow_forwardWrite a java class named First_Last_Recursive_Merge_Sort that implements the recursive algorithm for Merge Sort. You can use the structure below for your implementation. public class First_Last_Recursive_Merge_Sort { //This can be used to test your implementation. public static void main(String[] args) { final String[] items = {"Zeke", "Bob", "Ali", "John", "Jody", "Jamie", "Bill", "Rob", "Zeke", "Clayton"}; display(items, items.length - 1); mergeSort(items, 0, items.length - 1); display(items, items.length - 1); } private static <T extends Comparable<? super T>> void mergeSort(T[] a, int first, int last) { //<Your implementation of the recursive algorithm for Merge Sort should go here> } // end mergeSort private static <T extends Comparable<? super T>> void merge(T[] a, T[] tempArray, int first, int mid, int last) { //<Your implementation of the merge algorithm should go here> } // end merge //Just a quick method to display the whole array. public…arrow_forward
- What is the implementation of each instruction located in the main function of the following code? This is a sample program, i am trying to teach myself linked list and am having trouble ------------------------------------------------------------------------------- #include <iostream>#include <string> /*** node* * A node class used to make a linked list structure*/ template<typename type>struct node {node() : data(), next(nullptr) {}node(const type & value, node * link = nullptr) : data(value), next(link) {}type data;node * next;}; /*** print_list* @param list - the linked-list to print out* * Free function that prints out a linked-list space separated.* Templated so works with any node.* Use this for testing your code.*/ template<typename type>void print_list(node<type> * list) { for(node<type> * current = list; current != nullptr; current = current->next) {std::cout << current->data << ' ';}std::cout << '\n';} /***…arrow_forwardwrite a java code: Consider the following expression BNF: ::= * | / | :== + | - | ::= { }| :: 0|1|2|3|4|5|6|7|8|9 Using recursive descent, and only recursive descent, scan expressions that adhere to this BNF to build their expression tree; write an integer valued function that scans the tree to evaluate the expression represented by the tree. Input: A numeric expression adhering to this BNF. Output: Some representation of the expression tree. The result of evaluating the expression. write a java code for it.arrow_forwardWrite a program for Stack (Array-based or linked list-based) in Python. Test the scenario below with the implementation and with the reasoning of the answer. Make comments with a short description of what is implemented. Include source codes and screen-captured outputs. Stack: Given an empty stack in which the values A, B, C, D, E, F are pushed on the stack in that order but can be popped at any time, give a sequence of push and pop operations which results in pop()ed order of BADECFarrow_forward
- Modify the hash.java program to use quadratic probing instead of linear probing. // hash.java// demonstrates hash table with linear probingimport java.io.*; //////////////////////////////////////////////////////////////// class DataItem { // (could have more data) private int iData; // data item (key) //-------------------------------------------------------------- public DataItem(int ii) // constructor { iData = ii; } //-------------------------------------------------------------- public int getKey() { return iData; }//-------------------------------------------------------------- } // end class DataItem //////////////////////////////////////////////////////////////// class HashTable { private DataItem[] hashArray; // array holds hash table private int arraySize; private DataItem nonItem; // for deleted items // -------------------------------------------------------------…arrow_forwardWrite a java class named RecursiveMergeSort that implements the recursive algorithm for Merge Sort.You can use the structure below for your implementation.arrow_forwardOCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction -Command mul refers to integer multiplication -Command div refers to integer division -Command rem refers to the remainder of integer division -Command neg is to calculate the negation of an integer -Command swap interchanges the top two elements in the stack, meaning that the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
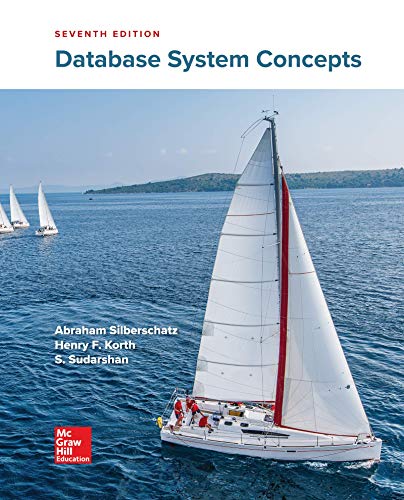
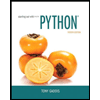
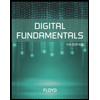
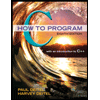
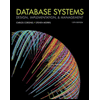
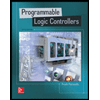