We will consider the Exam class seen in the Problem of the Lab 02. You are asked to define two more classes that inherits from the Exam class which are MidtermExam and LabExam, and, optionally, you can define Final Exam class. You have the freedom to make the design choices, but we would like to provide you some guidelines to simplify some aspects of your work. You can define a new class named DateTime that will include all members from Exam class concerning the date and time. Then, by composition, link the Exam with DateTime. Move all members specific to a subclass from the superclass to the subclass and keep the more general in the superclass. Move the specific processing from the constructor of the superclass to the constructor of the subclass. If a method performs different processing, which is related to superclass and subclass, redefine (override) the method in the subclass with the specific processing, and keep only the general processing in the superclass's method. Invoke the superclass constructor and methods whenever you need them in subclass. Keep the superclass members with their original access specifiers, unless you have wisely decided to upgrade their visibility. Test all the classes.
This is my question and this is the code in lab 2
The 1's are replaced with an E
public class 1xam
{
private int day;
private int month;
private int year;
private int hour;
private int minutes;
private double maxMarks;
private final String type;
private final int order;
private static int lab1xamCounter = 0;
private static int midterm1xamCounter = 0;
public static final String LAB_1XAM = "Lab 1xam";
public static final String MIDTERM_1XAM = "Midterm 1xam";
public static final String FINAL_1XAM = "Final 1xam";
public 1xam(String type)
{
this (type, 0, 0, 0, 0, 0, 0);
}
public 1xam(String type, double maxMarks)
{
this (type, maxMarks, 0, 0, 0, 0, 0);
}
public 1xam(String type, double maxMarks, int day, int month, int year)
{
this (type, maxMarks, day, month, year, 0, 0);
}
public 1xam(1xam other)
{
this (other.getType(), other.getMaxMarks(), other.getDay(), other.getMonth(), other.getYear(), other.getHour(), other.getMinutes());
}
public 1xam(String type, double maxMarks, int day, int month, int year, int hour, int minutes)
{
switch( type ) {
case LAB_1XAM:
this.order = ++lab1xamCounter;
this.maxMarks = (maxMarks > 5 && maxMarks <= 10 ? maxMarks : 10 );
break;
case MIDTERM_1XAM:
this.order = ++midterm1xamCounter;
this.maxMarks = (maxMarks > 15 && maxMarks <= 20 ? maxMarks : 20 );
break;
case FINAL_1XAM:
this.order = 0;
this.maxMarks = (maxMarks > 30 && maxMarks <= 40 ? maxMarks : 40 );
break;
default:
this.order = 0;
this.maxMarks = maxMarks;
}
if (day >= 1 && day <= 31)
this.day = day;
if (month >= 1 && month <= 12)
this.month = month;
if (year > 0)
this.year = year;
if (hour >= 0 && hour <= 23)
this.hour = hour;
if (minutes >= 0 && minutes <= 59)
this.minutes = minutes;
this.type = (type != null ? type : "");
}
public String getType()
{
return type;
}
public void setDay(int day)
{
if (day >= 1 && day <= 31)
this.day = day;
}
public int getDay()
{
return day;
}
public void setMonth(int month)
{
if (month >= 1 && month <= 12)
this.month = month;
}
public int getMonth()
{
return month;
}
public void setYear(int year)
{
if (year > 0)
this.year = year;
}
public int getYear()
{
return year;
}
public void setDate(int day, int month, int year)
{
this.setDay(day);
this.setMonth(month);
this.setYear(year);
}
public void setHour(int hour)
{
if (hour >= 0 && hour <= 23)
this.hour = hour;
}
public int getHour()
{
return hour;
}
public void setMinutes(int minutes)
{
if (minutes >= 0 && minutes <= 59)
this.minutes = minutes;
}
public int getMinutes()
{
return minutes;
}
public void setTime(int hour, int minutes)
{
this.setHour(hour);
this.setMinutes(minutes);
}
public void setMaxMarks(double maxMarks)
{
switch( this.type ) {
case LAB_1XAM:
this.maxMarks = (maxMarks > 5 && maxMarks <= 10 ? maxMarks : 10 );
break;
case MIDTERM_1XAM:
this.maxMarks = (maxMarks > 15 && maxMarks <= 20 ? maxMarks : 20 );
break;
case FINAL_1XAM:
this.maxMarks = (maxMarks > 30 && maxMarks <= 40 ? maxMarks : 40 );
break;
}
}
public double getMaxMarks()
{
return this.maxMarks;
}
public String toString()
{
return String.format("1xam: %s %s (%04.2f marks)\nDate: %d/%d/%d\nTime: %02d:%02d\n",
getType(), (order > 0 ? order : ""), getMaxMarks(), getDay(),
getMonth(), getYear(), getHour(), getMinutes());
}
public static String get1xamStats()
{
return LAB_1XAM + ": " + lab1xamCounter + " 1xam(s)\n" +
MIDTERM_1XAM + ": " + midterm1xamCounter + " 1xam(s)\n" ;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

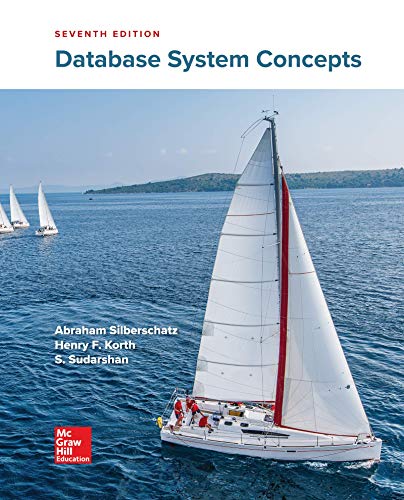
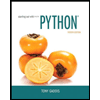
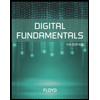
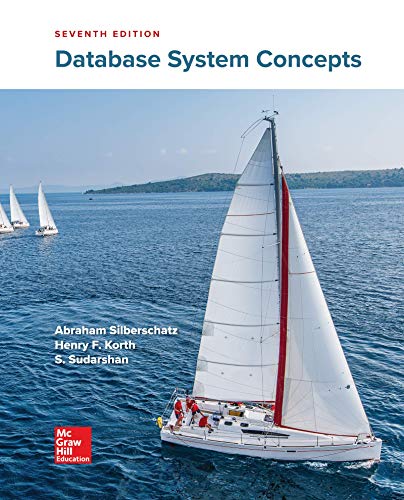
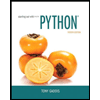
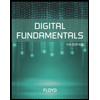
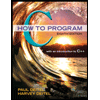
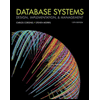
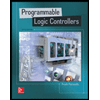