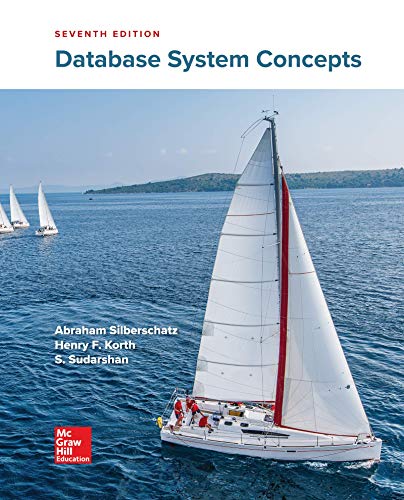
I REALLY NEED HELP!!!!!
We learnt this week that lists can be multi-dimensional. For e.g., the following is another example of 2-D multidimensional list. Each row contains student name followed by their grades in 5 subjects:
students = [ ['Anna', 98.5, 77.5, 89, 93.5, 85.5], ['Bob', 77, 66.5, 54, 90, 85.5], ['Sam', 98, 97, 89.5, 92.5, 96.5] ]
To access, a specific row, you would use students[row_number][column_number].
- students[0][0] would print 'Anna'
- students[0][1] would print 98.5
Write a program that defines a function that takes a list as an argument, adds the scores of each student, calculate average for each student (append them to a separate list) and display them.
Your program should:
-
Define a function display_average(students) that takes in a 2-D list as an argument. Display the original list using for/while loop. Calculate and display the average of each student.
-
You do not need to ask user for input. You can use your own 2-D lists with at-least 2-rows.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- We learnt this week that lists can be multi-dimensional. For e.g., the following is another example of 2-D multidimensional list. Each row contains student name followed by their grades in 5 subjects: students = [ ['Anna', 98.5, 77.5, 89, 93.5, 85.5], ['Bob', 77, 66.5, 54, 90, 85.5], ['Sam', 98, 97, 89.5, 92.5, 96.5] ] To access, a specific row, you would use students[row_number][column_number]. students[0][0] would print 'Anna' students[0][1] would print 98.5 and so on.. Write a program that defines a function that takes a list as an argument, adds the scores of each student, calculate average for each student (append them to a separate list) and display them. Your program should: Define a function display_average(students) that takes in a 2-D list as an argument. Display the original list using for/while loop. Calculate and display the average of each student. You do not need to ask user for input. You can use your own 2-D lists with at-least 2-rows.arrow_forwarddef create_category(info): li = info.split() if len(li) == 2: if is_numeric(li[1]) == True: li[2] = float(l[2]) else: return -1 else: return -2 """ Given a string `info`, which is supposed to contain the category name and its percentage, return a two-element list, which contains this information stored as a string and a float respectively. Calls is_numeric() to verify that the percentage is a numeric value (int or float). Stores the percentage as a float (not as a string). E.g., if the input is "Quiz 25", the function returns a list: ["Quiz", 25.0] If splitting the `info` string does not result in a two-element list, then return -2. If the last input value (the percentage) in `info` is not numeric (int or float), does not update the list and returns -1 instead. """ debugarrow_forwardA fibonacci series is defined as a series where the number at the current index, is the value of the summation of the index preceding it (index -1) and (index-2). Essentially, for a list fibonacci_numbers which is the fibonacci numbers series, fibonacci_numbers[i] = fibonacci_numbers[i-1] + fibonacci_numbers[i-2] The fibonacci series always begins with 0, and then a 1 follows. So an example for fibonacci series up to the first 7 values would be - 0, 1, 1, 2, 3, 5, 8, 13 Complete the fibonacci(n) function, which takes in an index, n, and returns the nth value in the sequence. Any negative index values should return -1. Ex: If the input is: 7 the output is: fibonacci(7) is 13 Important Note Use recursion and DO NOT use any loops. Review the Week 9 class recording to see a variation of this solution. def fibonacci(n): if (n < 0 ): return -1 else: return n fibonacci_numbers = (fibonacci[n - 1] + fibonacci[n - 2]) return fibonacci_numbers(n) # TODO: Write…arrow_forward
- Suppose a list contains marks earned in the courses CSE110, PHY111, and MAT110 of each student consecutively in a nested list form. Your task is to take a course name as input and sort the list based on the marks obtained in that course to finally print the names of the students in descending order of marks obtained i.e. from the student who earned the highest marks to the student who earned the lowest. For example, the list may look like stu_lst = [ ["Alan", 95, 87, 91], ["Turing", 92, 90, 83], ["Elon", 87, 92, 80], ["Musk", 85, 94, 90] ] where for each nested list, 1st index holds the name of the student, 2nd index is total marks earned in the CSE110 course, 3rd index is PHY111 marks and 4th index is MAT110 marks. [You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead] =============================================================== Sample Input 1 MAT110 Sample Output 1 ['Alan', 'Musk', 'Turing', 'Elon']…arrow_forwardWrite a program in Java to manipulate a Singly Linked List: Count the number of nodes Insert a new node before the value 5 of Singly Linked List Search an existing element in a Singly linked list (the element of search is given by the user) Suppose List contained the following Test Data: Input data for node 1: 2Input data for node 2 : 3Input data for node 3 : 5 Input data for node 4: 8arrow_forwardJavaScript Given a singly linked list of integers, determine whether or not it's a palindrome. // Singly-linked lists are already defined with this interface: // function ListNode(x) { // this.value = x; // this.next = null; // } // function isListPalindrome(head) { } Note: in examples below and tests preview linked lists are presented as arrays just for simplicity of visualization: in real data you will be given a head node l of the linked list Example For l = [0, 1, 0], the output should beisListPalindrome(l) = true; For l = [1, 2, 2, 3], the output should beisListPalindrome(l) = false.arrow_forward
- python LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardIn Python, Create 5 lists from grades = [99,87,76,54,91,65,84,69,72], one for each grade, that is, A to F. For instance, a list that holds B grades taken from the grades Panda Series would be: B = list(grades[(grades >= 80) & (grades < 90)]). Create a pie chart where the slices are the number of elements in each one of the lists of A, B, C, D, F. For colors, use r, g, b, y, m, start at 90◦, use shadow, and explode the F grades in the pie chart.arrow_forwardThe code fragment above contains a list of integers named my_list and a pair of counter-controlled loops. Once this code has been executed the variables qux and egg will have been assigned values. Let x denote the average those two values. If you were to create a new list that contains every integer in my_list that is greater than than x but also less than than x + 7 then what would be the length of that list? This question is internally recognized as variant 235. Q13) 1 2 3 4 5 6 7 8 none of the abovearrow_forward
- Question 1 5 pts A regular expression is shorthand for a set of strings. For example (b+ba)* ba (a+ab)* represents a set of strings for which the first two elements (when listed in length order with same length strings listed in alphabetical order) are: ba and baa. What are the next three strings in this sequence? Next string: Next after that: Next after that:arrow_forwardIn Python Write code that creates a LIST (data type) of only the first names of the students who are juniors. call it juniorRoster= Given the folowing data set: studentDataScores =…arrow_forwardWrite a program that implements a sorted list using dynamic allocated arrays. DataFile.txt contains the information of poker cards. C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest) 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A No Joker cards Any C cards are lower than any D cards. DataFile Content(You can write the file specification into your program.): H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10 H4, D5, HK, D2 H4, HK, SK C9,C10 For examples, DJ means J of Diamonds;H7 means 7 of hearts. Your job Create a list by dynamic allocated array and set the size to 20 Read the first 20 cards in the first line of the file, the put them one by one into the list by implementing and using putItem(). The list must be kept sorted in ascending order. Then print out all the cards in the list in one line separating by commas. Then delete the cards indicated in the second…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
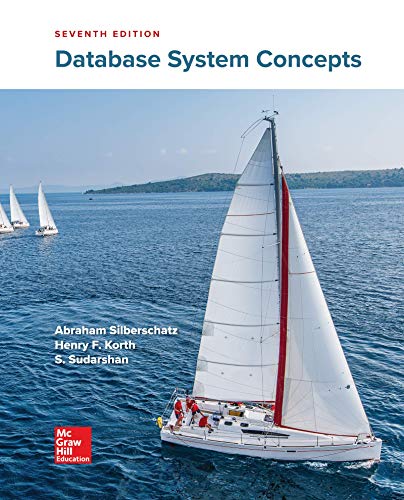
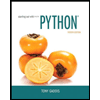
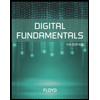
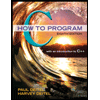
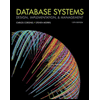
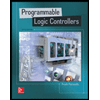