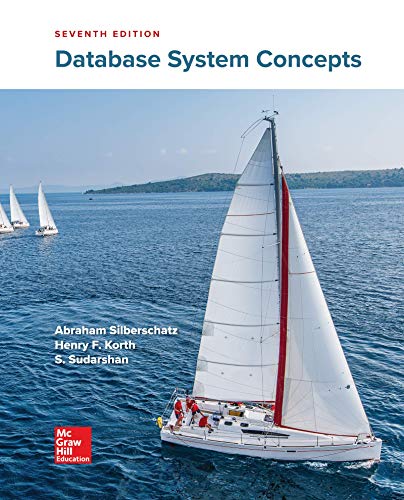
Concept explainers
C PROGRAMMING
Instructions:
Using the code template below, create a function that computes the average of all the elements in the array using a 'for' loop to sum the array. Use an array pointer to get the values from the array. The average is the return value from the function. Then, implement the function into the main() method.
/*_____________________________________________________________________________*/
#include <stdio.h>
#include <stdlib.h>
int main()
{
float data[] = {2.5, 3.33, 4.2, 8.0, 5.1};
float sum = 0;
printf("Index\tValues\n");
for(int i = 0; i < 5; i++)
{
printf("%d\t%f", i, data[i]);
printf("\n");
sum = sum + data[i];
}
sum = sum / 5;
printf("Avg\t%f", sum);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- Using c++arrow_forward2. Lottery Number Generator Design a program that generates a 7-digit lottery number. The program should have an Integer array with 7 elements. Write a loop that steps through the array, ran- domly generating a number in the range of 0 through 9 for each element. (Use the random function that was discussed in Chapter 6.) Then write another loop that displays the contents of the array.arrow_forward9arrow_forward
- Create Array of Random Dates In this task you are required to write a function that will generate a column array (a variable called dates) containing N random dates. N is an integer (whole number) entered by the user and will indicate to the function how many random dates the user requires. The dates array will be structured as follows: • The array shall have two columns; column 1 will contain numbers indicating the day of each month (1-31) and column 2 will contain numbers indicating the month (Jan 1, Feb=2, Mar = 3.... Dec = 12). . Each day must be randomly generated (using the randi() function) taking into account the maximum number of days in each month (i.e. Jan, Mar, May, Jul, Aug. Oct and Dec have 31 days, Apr. Jun, Sep and Nov have 30 days and Feb will have 28 days). You must use an if-elseif-else statement to decide this. • We are assuming no leap years (e. February can only have days between 1-28). For example, the output from calling the function: output = dategen (3) could…arrow_forwardC++ Do the following lab by an array(not vector) for 3 students find the average of a student by asking him how many grades do you have. The answer to this question, determine the size of the array(list of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Find the maximum and minimum grades for each student. Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98arrow_forwardprogramming used: javaarrow_forward
- Topic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forwardTopic: pointers, dynamic array and command line arguments Write a function named "getDynamicNumList" that accepts a count (integer) for the totalof all the numbers to obtain storage for. The function will allocate an array of integer pointers dynamically, then read in from the user that many numbers. For each number, it will allocate memory for each integer initialized it with what the userenters and save its pointer in the new array. It then returns the pointer of the array of integer pointers to the caller. The caller should print out this array based on that description.Here is an example of calling getDynamicNumList with 4. It will allocate an array of 4 pointers and read in 10, 55, 76 and 1. Each integer will have its own dynamic memory location.arrow_forwardWhen traversing an array, which of the following statements does not need to keep track of the individual array subscripts: Do...Loop, For...Next, or For Each...Next?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
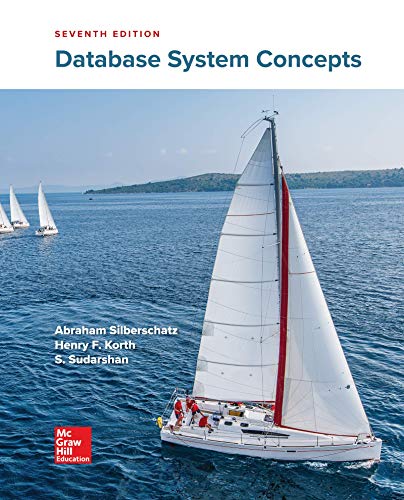
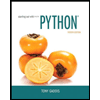
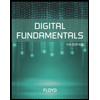
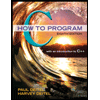
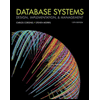
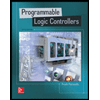