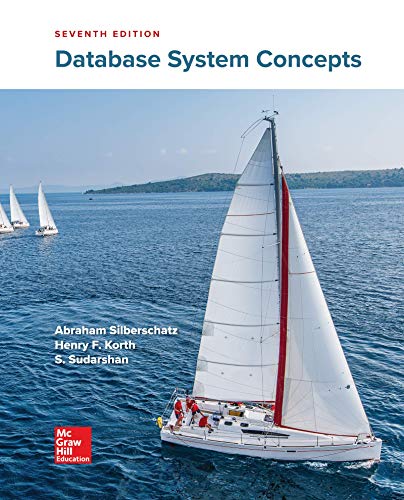
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Using the code below, answer the following questions:
1) What are each of the 3 functions responsible for?
2) How many parameters will each function have?
3) What does each function return?
4) How will the program be controlled?
5) What functions will it call?
6) How will it communicate the results with the user?
********************************************* Code starts here **************************************************
Module.py
#Defination to sort the list
def sort(listNum):
sortedList = []
#While loop will run until the listNum don't get null
while(len(listNum) != 0 ):
#Set the min as first element in list
min = listNum[0]
#iterate over the list to compare every element with num
for ele in listNum:
#If element is less than min
if ele < min:
#Then set min as element
min = ele
#append the sorted element in list
sortedList.append(min)
#Remove the sorted element from the list
listNum.remove(min)
return sortedList
#Function to find the sum of all elements in list
def SumOfList(listNum):
#Set the sum as zero
sum =0
#Iterate over the list to get every element
for ele in listNum:
#Keep adding the element to the sum variable
sum = sum + ele
#returnt the sum
return sum
#Function to find the max of the list
def listMax(listNum):
#To store the max
max =0
#Iterate over the list
for ele in listNum:
#If element is greater than max then max = element
if(ele>max):
max = ele
#Return the max
return max
***************************************************************************************
Main .py
#Import the module file having the 3 functions
import module
import random
def main():
#use the random module to create the list of random integers
#To store the random numbers in range 0 to 10
randomList = []
#Create the loop to create the list of 5 random integers
for i in range(0,5):
#Use randint to create the random integer
num = random.randint(0,10)
#Add the random integer into the list
randomList.append(num)
#Now create the menu to let user choose from what to perform on the random list
print("Random list created : ",randomList,"\nSpecify what operations you wanna perform on the list")
print("1:Sorting\n2:Sum of all elements \n3:Maximum\nChooce 1-3 : ",end = "")
#Now take input from the user
choice = int(input())
#Now use decision structures to decide which function to call from module.py
if(choice == 1 ):
#Print the sorted list
print("Sorted list as follows : " ,module.sort(randomList))
elif(choice == 2):
#Print the sum of all elements in list
print("The sum of all elements in list : ",module.SumOfList(randomList))
elif(choice == 3):
#print the maximum of list
print("The maximum of list is : ",module.listMax(randomList))
#When the __name__ will be ___main__ the main function will be called.
if __name__ == "__main__":
main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Given the code below, in your solution cell: make the necessary changes to write the code as a function. You can choose how to name your function, but try make sure it reflects what it is supposed to do. Identify which variables from the given code should be arguments to the function instead The result should be the value returned by your function Document your function using comments Provide 3 test cases with expected outputs for the function created. # the given code # initializing the variablesnumlist = [10, 5, 14, 8, 74]result = [[],[]]# for each elem in numlist insert a value into resultfor num in numlist: if num%3==0: result[0].append(num) else: result[1].append(num)# the final result print(result) # your solution: # Your test cases (3 test cases)arrow_forwardHere are three versions of a code fragment located inside a function: Version A for Row in range(10): for Column in range(5): DoSomethingInteresting(Row,Column) return Version B for Row in range(10): for Column in range(5): DoSomethingInteresting(Row,Column) return Version C for Row in range(10): for Column in range(5): DoSomethingInteresting(Row,Column) return How many times do each of the versions call the DoSomethingInteresting function? Write a sentence or two explaining which version is the "correct" versionarrow_forwardWhat is the return value of a function that doesn't specify a return statement or simply has an empty return statement? Example: def x(a,b): print(x) returnarrow_forward
- When a function calls another function in pep/9, describe the steps involved. How do they communicate information, and how does the calling software continue to run once the call is completed?arrow_forward1. Write function printGrade in void function to compute and output the course grade. The course score is passed as a parameter to the function printGrade. (The program will take 5 inputs for 5 subjects. And show the grade of each subject. And calculate the CGPA) TTTE Percentage Score Quality Point Renark Letter Grade 8e - 100 75 - 79 70- 74 65- 69 6e- 64 55- 59 se - 54 45 - 49 40 - 44 35 - 39 e. 34 Excellent Extrenely Good Very Good Good Fairly Good Satisfactory Quite Satisfactory Poor 4.00 3.67 3.33 3.00 2.67 2.33 2.e0 1.67 1.33 Very Poor Extremely Poor Fail 1.00 Expected Output: Inputs 1. Mark for the programming is: 2. Mark for the Math is: 80 85 3. Mark for the Database System is: 4. Mark for the Software Engineering is: 5. Mark for the Business Fundamental is: 74 76 65 Output Letter Grade for Programming: A Letter Grade for Math: A Letter Grade for Database System: Letter Grade for Software Engineering: Letter Grade for Business Fundamental: Remark: B+ A- в Extremely Good 2.…arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Write a function diceprob() that takes a possible result r of a roll of pair of dice (i.e. aninteger between 2 and 12) and simulates repeated rolls of a pair of dice until 100 rolls of rhave been obtained. Your function should print how many rolls it took to obtain 100 rollsof r.>>> diceprob(2)It took 4007 rolls to get 100 rolls of 2>>> diceprob(3)It took 1762 rolls to get 100 rolls of 3>>> diceprob(4)It took 1058 rolls to get 100 rolls of 4>>> diceprob(5)It took 1075 rolls to get 100 rolls of 5>>> diceprob(6)It took 760 rolls to get 100 rolls of 6>>> diceprob(7)It took 560 rolls to get 100 rolls of 7arrow_forward
- Exercise 4 A number, a, is a power of b if it is divisible by b and a/b is a power of b. Write a function called is_power that takes parameters a and b and returns True if a is a power of b. Note: you will have to think about the base case.arrow_forwardAll lines of code in your program can be reached and executed when I run your program define two functions and they have a "meaningful purpose" to your program. Each function must have more than three statements or lines of code. At least one function defines a parameter and you use this parameter in a meaningful way At least one function returns a value back to the code that is calling it and it is used in a meaningful wayarrow_forwardUnique answer onlyarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
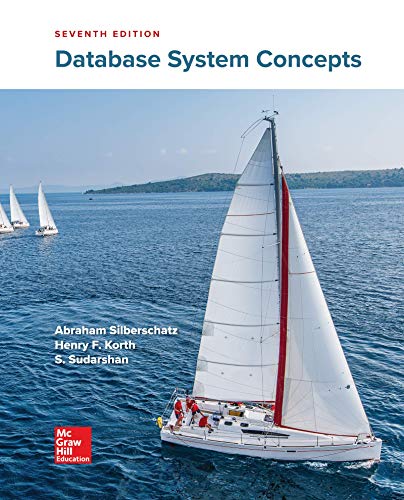
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
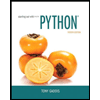
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
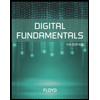
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
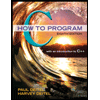
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
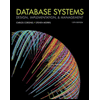
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
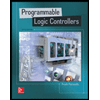
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education