Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible origin/destinations possible combinations from the airport codes provided. Load the origin/destination string onto a stack for processing. Do not load same/same values, such as DALDAL, or LAXLAX. See comments in the program from more details. After loading the values, Create a loop to display all the values in the container. This loop should inherently remove items as they are displayed. Deliverable is a working CPP program
Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic.
Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible origin/destinations possible combinations from the airport codes provided. Load the origin/destination string onto a stack for processing. Do not load same/same values, such as DALDAL, or LAXLAX. See comments in the program from more details.
After loading the values, Create a loop to display all the values in the container. This loop should inherently remove items as they are displayed.
Deliverable is a working CPP program.
![code.cpp ) No Selection
C++
Create all the possible origin/destinations from the airport codes provided.
Load the origin/destination string onto a stack or queue for processing
1
3
4 */|
7 // MS Visual Studio 2015 uses "stdafx.h"
2017 uses "pch.h"
8
//#include "stdafx.h"
9 |/#include "pch.h"
10
11
12
#include <iostream>
13
#include <string>
14
15
using namespace std;
16
const int AIRPORT_COUNT =
string airports[AIRPORT_COUNT]
{"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"};
17
12;
18
%3D
19
20
int main()
21
{
22
// define stack (or queue ) here
23
string origin;
string dest;
string citypair;
24
25
26
27
cout << "Loading the CONTAINER
// LOAD THE STACK ( or queue) HERE
// Create all the possible Airport combinations that could exist from the list provided.
// i.e DALABQ, DALDEN,
// DO NOT Load SameSame
28
<« endl;
29
30
...., ABQDAL, ABQDEN
DALDAL, ABQABQ, etc
31
32
33
34
<< endl;
cout << "Getting data from the CONTAINER
// Retrieve data from the STACK/QUEUE here
35
•. I"
36
37
38
39
}
40](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F98856484-cfe4-4b99-b613-d8917fb53fcb%2Fb02655fe-2275-4220-8ac8-85c414e571a5%2F8y1j0h_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

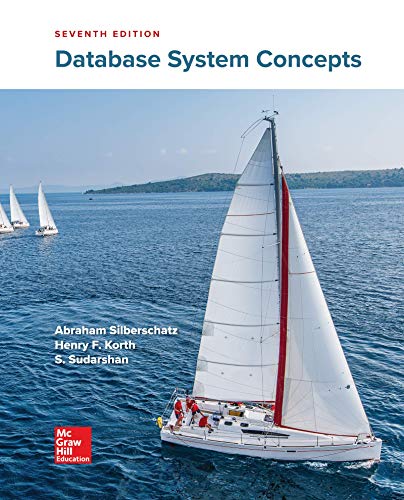
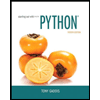
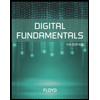
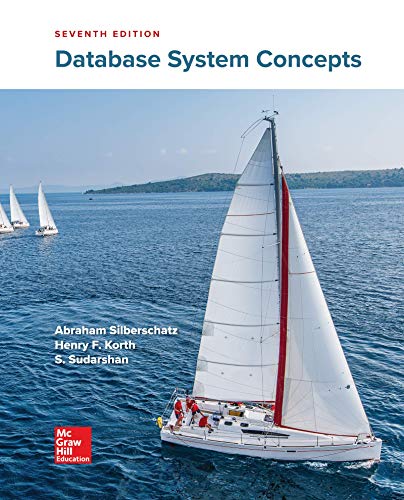
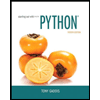
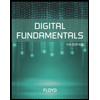
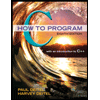
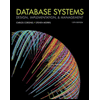
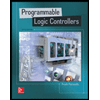