using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace LibraryManagment { class Program {// private static int userInput;// need varaible to capture user input = int public static string Title { get; private set; } public static bool IsChecked { get; private set; } //Implement a menu system that allows users to add books, check out books, //and check in books using the console. Use Console.ReadLine() ///to capture user input and Console.WriteLine() for output. static void Main(string[] args) {// provides a cosole interaction for a user while (IsChecked) { Console.WriteLine("\nMenu\n" + "1)Add book\n" + "2)Check Out Book\n" + "3)Check In Book\n" + "4)Exit\n"); Console.Write("Choose your option from menu :"); int userInput = int.Parse(Console.ReadLine); if (userInput == 1) { Books.AddBook(); // need to create a variable } else if (userInput == 2) { CheckOut(); } else if (userInput == 3) { CheckIn(); } else if (userInput == 4) { Console.WriteLine("Thank you"); Exit; } } } } //Create a base class Book public abstract class Book // create a base cass Book {//with properties for Title (string), Author (string), and IsCheckedOut (bool). //Include a constructor to set the title and author, and initialize IsCheckedOut to false. public string Title { get; set; } public string Author { get; set; } public bool IsChecked = false; // and initialize IsCheckedOut to false. // construtor , always has the same name as the class. , no return type, this creates the object with default vales Book(string Title, string Author, bool IsChecked) { Title = this.Title; Author = this.Author; IsChecked = this.IsChecked; } virtual public void CheckOut() {// this refers to the current instance of the class if (!this.IsChecked)// not equal { Console.WriteLine($"The book { Title } has been checked out, "); this.IsChecked = true;// the book is checked out } } virtual public void CheckIn() { if (this.IsChecked)// if the book is checked in { Console.WriteLine($"The book { Title } has been checked in. "); this.IsChecked = false;// the book is checked out } } // static class LibraryCatalog that manages a collection of books. Use a static list to store instances of Book. public static class LibraryCatalog { //Use a static list to store instances of Book - to store a list of the books public static List booksList = new List(); public static List booksListB = new List(); // methods to manage the books public static void AddBook(Book book) { booksList.Add(book); } // How are we using the String as the title public static void CheckOutBook(string title) { booksListB.Remove(title); Console.WriteLine("Book checked out successfully "); // else or Console.WriteLine("Book not found"); // "Book not found". } public static void checkInBook(string title) { booksListB.Add(title); Console.WriteLine("Book checked in successfully. "); } // Ensure methods provide feedback, e.g., // "Book checked out successfully" or "Book not found". } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace LibraryManagment { class Program {// private static int userInput;// need varaible to capture user input = int public static string Title { get; private set; } public static bool IsChecked { get; private set; } //Implement a menu system that allows users to add books, check out books, //and check in books using the console. Use Console.ReadLine() ///to capture user input and Console.WriteLine() for output. static void Main(string[] args) {// provides a cosole interaction for a user while (IsChecked) { Console.WriteLine("\nMenu\n" + "1)Add book\n" + "2)Check Out Book\n" + "3)Check In Book\n" + "4)Exit\n"); Console.Write("Choose your option from menu :"); int userInput = int.Parse(Console.ReadLine); if (userInput == 1) { Books.AddBook(); // need to create a variable } else if (userInput == 2) { CheckOut(); } else if (userInput == 3) { CheckIn(); } else if (userInput == 4) { Console.WriteLine("Thank you"); Exit; } } } } //Create a base class Book public abstract class Book // create a base cass Book {//with properties for Title (string), Author (string), and IsCheckedOut (bool). //Include a constructor to set the title and author, and initialize IsCheckedOut to false. public string Title { get; set; } public string Author { get; set; } public bool IsChecked = false; // and initialize IsCheckedOut to false. // construtor , always has the same name as the class. , no return type, this creates the object with default vales Book(string Title, string Author, bool IsChecked) { Title = this.Title; Author = this.Author; IsChecked = this.IsChecked; } virtual public void CheckOut() {// this refers to the current instance of the class if (!this.IsChecked)// not equal { Console.WriteLine($"The book { Title } has been checked out, "); this.IsChecked = true;// the book is checked out } } virtual public void CheckIn() { if (this.IsChecked)// if the book is checked in { Console.WriteLine($"The book { Title } has been checked in. "); this.IsChecked = false;// the book is checked out } } // static class LibraryCatalog that manages a collection of books. Use a static list to store instances of Book. public static class LibraryCatalog { //Use a static list to store instances of Book - to store a list of the books public static List booksList = new List(); public static List booksListB = new List(); // methods to manage the books public static void AddBook(Book book) { booksList.Add(book); } // How are we using the String as the title public static void CheckOutBook(string title) { booksListB.Remove(title); Console.WriteLine("Book checked out successfully "); // else or Console.WriteLine("Book not found"); // "Book not found". } public static void checkInBook(string title) { booksListB.Add(title); Console.WriteLine("Book checked in successfully. "); } // Ensure methods provide feedback, e.g., // "Book checked out successfully" or "Book not found". } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LibraryManagment
{
class Program
{//
private static int userInput;// need varaible to capture user input = int
public static string Title { get; private set; }
public static bool IsChecked { get; private set; }
//Implement a menu system that allows users to add books, check out books,
//and check in books using the console. Use Console.ReadLine()
///to capture user input and Console.WriteLine() for output.
static void Main(string[] args)
{// provides a cosole interaction for a user
while (IsChecked) {
Console.WriteLine("\nMenu\n" +
"1)Add book\n" +
"2)Check Out Book\n" +
"3)Check In Book\n" +
"4)Exit\n");
Console.Write("Choose your option from menu :");
int userInput = int.Parse(Console.ReadLine);
if (userInput == 1)
{
Books.AddBook(); // need to create a variable
}
else if (userInput == 2)
{
CheckOut();
}
else if (userInput == 3)
{
CheckIn();
}
else if (userInput == 4)
{
Console.WriteLine("Thank you");
Exit;
}
}
}
}
//Create a base class Book
public abstract class Book // create a base cass Book
{//with properties for Title (string), Author (string), and IsCheckedOut (bool).
//Include a constructor to set the title and author, and initialize IsCheckedOut to false.
public string Title { get; set; }
public string Author { get; set; }
public bool IsChecked = false; // and initialize IsCheckedOut to false.
// construtor , always has the same name as the class. , no return type, this creates the object with default vales
Book(string Title, string Author, bool IsChecked)
{
Title = this.Title;
Author = this.Author;
IsChecked = this.IsChecked;
}
virtual public void CheckOut()
{// this refers to the current instance of the class
if (!this.IsChecked)// not equal
{
Console.WriteLine($"The book { Title } has been checked out, ");
this.IsChecked = true;// the book is checked out
}
}
virtual public void CheckIn()
{
if (this.IsChecked)// if the book is checked in
{
Console.WriteLine($"The book { Title } has been checked in. ");
this.IsChecked = false;// the book is checked out
}
}
// static class LibraryCatalog that manages a collection of books. Use a static list to store instances of Book.
public static class LibraryCatalog
{ //Use a static list to store instances of Book - to store a list of the books
public static List booksList = new List();
public static List booksListB = new List();
// methods to manage the books
public static void AddBook(Book book)
{
booksList.Add(book);
}
// How are we using the String as the title
public static void CheckOutBook(string title)
{
booksListB.Remove(title);
Console.WriteLine("Book checked out successfully ");
// else or
Console.WriteLine("Book not found");
// "Book not found".
}
public static void checkInBook(string title)
{
booksListB.Add(title);
Console.WriteLine("Book checked in successfully. ");
}
// Ensure methods provide feedback, e.g.,
// "Book checked out successfully" or "Book not found".
} }
}
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
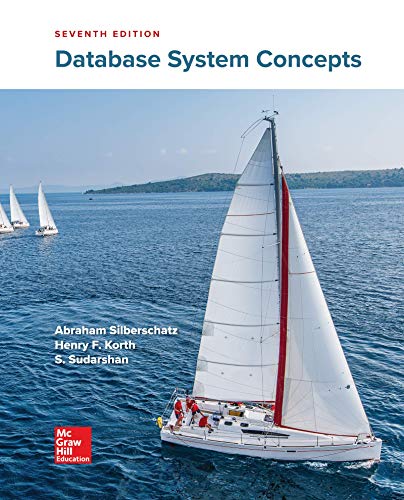
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
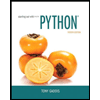
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
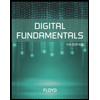
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
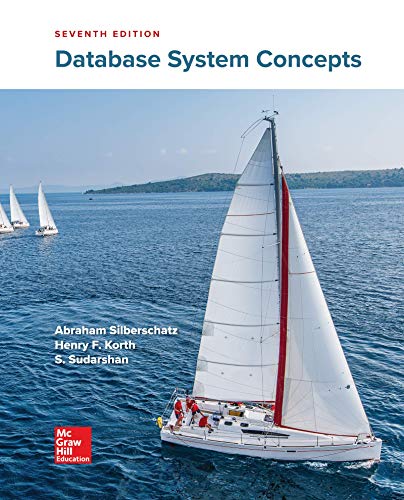
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
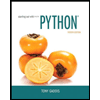
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
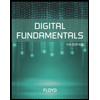
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
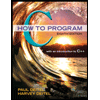
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
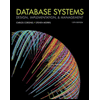
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
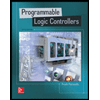
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education