USING PYTHON: Create a function that takes a filename("string") of a PGM image file as input and loads in the image in the file, and returns it as an image matrix
USING PYTHON: Create a function that takes a filename("string") of a PGM image file as input and loads in the image in the file, and returns it as an image matrix
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
USING PYTHON: Create a function that takes a filename("string") of a PGM image file as input and loads in the image in the file, and returns it as an image matrix.
Rules: Cannot make any input calls in the main body, use basic python (nothing like .shape or .width)
I have attached an example of what the function should do, as well as what the PGM image file looks like.

Transcribed Image Text:P2
24 7
255
О
0
0
0
0
0 51 51 51 51
0 51 О О
О
0 51
О
0
О
0 51 О
О
О
0 51 51 51 51
О
0 0
0000
О О
О
О 0 0 0 0 0
119 119 119 119 119 0
О О О 0 0 О 0 О 0
187 187
0 187 187 187 187 187 0
187 187 0 255 255 255 255
0 0
119
0
О
0 119 0
187
0 0 119
0
О
0 119 0 187
187
0 187
0 187
0 187
0 187
0 187 0 255 0 0 255
0 187 0 255 255 255 255
0 187 0 255
0 187 0 255
О
0
О
О
О
0 0 119
51 0 119 119 119 119
0 119 0 187
119 0 187
О
О
0 0
0
0 0
О 00
О
0
О
0
0 0 0
О
0 0
0
51
О
О
О
0
О
О
О
О
О
О
![• load_regular_image: takes a filename (string) of a PGM image file as input, and loads in the image
contained in the file and returns it as an image matrix. If, during or after loading, the resulting
image matrix is found to not be in PGM format, then a AssertionError with an appropriate error
message should be raised instead. (The file could have anything inside it, not necessarily a valid
image matrix.)
Note: For this and further examples, we put each sublist on its own line for readability purposes.
When you write your examples in the docstring, however, the output must be all on one line for
the doctest to work properly.
>>> load_regular_image("comp.pgm")
[[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 51, 51, 51, 51, 51, 0, 119, 119, 119, 119, 119, 0, 187, 187, 187, 187, 187, 0, 255,
9, 12⁹, 0,
255, 255, 255, 0],
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0,
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0,
0],
119, 0, 187, 0, 187, 0, 187, 0, 255, 0, 0, 255, 0],
119, 0, 187, 0, 187, 0, 187, 0, 255, 255, 255, 255,
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0, 119, 0, 187, 0, 187, 0, 187, 0, 255, 0, 0, 0, 0],
[0, 51, 51, 51, 51, 51, 0, 119, 119, 119, 119, 119, 0, 187, 0, 187, 0, 187, 0, 255, 0,
0, 0, 0],
[0, 0, 0, 0, 0, 0,
0, 0, 0,
0,
0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2e9adce6-7ce4-405a-96b4-36bd26f752aa%2Ffe2ce92a-d5ec-4ef9-ab7d-9b2b4b799220%2Fvm8tvhb_processed.png&w=3840&q=75)
Transcribed Image Text:• load_regular_image: takes a filename (string) of a PGM image file as input, and loads in the image
contained in the file and returns it as an image matrix. If, during or after loading, the resulting
image matrix is found to not be in PGM format, then a AssertionError with an appropriate error
message should be raised instead. (The file could have anything inside it, not necessarily a valid
image matrix.)
Note: For this and further examples, we put each sublist on its own line for readability purposes.
When you write your examples in the docstring, however, the output must be all on one line for
the doctest to work properly.
>>> load_regular_image("comp.pgm")
[[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 51, 51, 51, 51, 51, 0, 119, 119, 119, 119, 119, 0, 187, 187, 187, 187, 187, 0, 255,
9, 12⁹, 0,
255, 255, 255, 0],
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0,
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0,
0],
119, 0, 187, 0, 187, 0, 187, 0, 255, 0, 0, 255, 0],
119, 0, 187, 0, 187, 0, 187, 0, 255, 255, 255, 255,
[0, 51, 0, 0, 0, 0, 0, 119, 0, 0, 0, 119, 0, 187, 0, 187, 0, 187, 0, 255, 0, 0, 0, 0],
[0, 51, 51, 51, 51, 51, 0, 119, 119, 119, 119, 119, 0, 187, 0, 187, 0, 187, 0, 255, 0,
0, 0, 0],
[0, 0, 0, 0, 0, 0,
0, 0, 0,
0,
0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
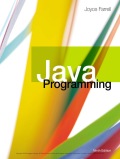
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
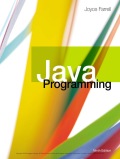
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT