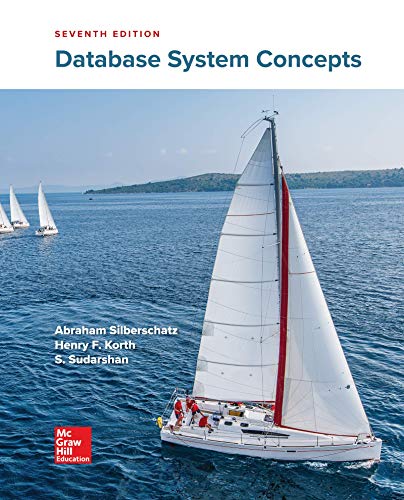
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Use C++
Using dynamicarrays, implement a polynomial class with polynomial addition, subtraction, and multiplication.
Implement addition and subtraction functions first.
Multiplication function –extra point
![## Polynomial Class Implementation
The image provides a code snippet for implementing a Polynomial class in C++ and explains its structure with diagrams illustrating how to handle polynomial expressions dynamically.
### Code Explanation:
```cpp
class Polynomial
{
public:
Polynomial();
Polynomial(int d);
Polynomial(int* c, int d);
int getDegree();
int* getCoef();
friend Polynomial add(Polynomial x, Polynomial y);
friend Polynomial subtract(Polynomial x, Polynomial y);
// friend Polynomial multiply(Polynomial x, Polynomial y); // extra point
private:
int* coef;
int degree;
};
```
- **Constructors:**
- `Polynomial()`: Default constructor.
- `Polynomial(int d)`: Initializes a polynomial with a specific degree `d`.
- `Polynomial(int* c, int d)`: Initializes with coefficients `c` and degree `d`.
- **Methods:**
- `int getDegree()`: Returns the degree of the polynomial.
- `int* getCoef()`: Returns the coefficients array.
- **Friend Functions:**
- `add(Polynomial x, Polynomial y)`: Adds two polynomials.
- `subtract(Polynomial x, Polynomial y)`: Subtracts one polynomial from another.
- `multiply(Polynomial x, Polynomial y)`: (Commented out) Multiplies two polynomials.
### Note:
> *If needed, you can add additional member functions.*
### Diagram Explanation:
- **Object A:**
- Polynomial: \( A = 4x^7 + 5x^6 + 3x^3 + 2x^1 + 8x^0 \)
- Degree: 7
- Coefficients Array: `[8, 0, 2, 0, 0, 3, 0, 5, 4]`
- **Object B:**
- Polynomial: \( B = 6x^8 + 3x^6 + 4x^5 + 8x^3 + 3x^1 + 2x^0 \)
- Degree: 8
- Coefficients Array: `[2, 0, 3, 0, 0, 0, 3, 0, 6]`
### Graphs Explanation:
- **Dynamic Array:**
- Both objects use a dynamic array](https://content.bartleby.com/qna-images/question/cdbdf4c8-5459-45de-99f8-cd8d71031beb/07b52563-37af-444b-b3f9-8e1bb7705e03/kwj2758_thumbnail.png)
Transcribed Image Text:## Polynomial Class Implementation
The image provides a code snippet for implementing a Polynomial class in C++ and explains its structure with diagrams illustrating how to handle polynomial expressions dynamically.
### Code Explanation:
```cpp
class Polynomial
{
public:
Polynomial();
Polynomial(int d);
Polynomial(int* c, int d);
int getDegree();
int* getCoef();
friend Polynomial add(Polynomial x, Polynomial y);
friend Polynomial subtract(Polynomial x, Polynomial y);
// friend Polynomial multiply(Polynomial x, Polynomial y); // extra point
private:
int* coef;
int degree;
};
```
- **Constructors:**
- `Polynomial()`: Default constructor.
- `Polynomial(int d)`: Initializes a polynomial with a specific degree `d`.
- `Polynomial(int* c, int d)`: Initializes with coefficients `c` and degree `d`.
- **Methods:**
- `int getDegree()`: Returns the degree of the polynomial.
- `int* getCoef()`: Returns the coefficients array.
- **Friend Functions:**
- `add(Polynomial x, Polynomial y)`: Adds two polynomials.
- `subtract(Polynomial x, Polynomial y)`: Subtracts one polynomial from another.
- `multiply(Polynomial x, Polynomial y)`: (Commented out) Multiplies two polynomials.
### Note:
> *If needed, you can add additional member functions.*
### Diagram Explanation:
- **Object A:**
- Polynomial: \( A = 4x^7 + 5x^6 + 3x^3 + 2x^1 + 8x^0 \)
- Degree: 7
- Coefficients Array: `[8, 0, 2, 0, 0, 3, 0, 5, 4]`
- **Object B:**
- Polynomial: \( B = 6x^8 + 3x^6 + 4x^5 + 8x^3 + 3x^1 + 2x^0 \)
- Degree: 8
- Coefficients Array: `[2, 0, 3, 0, 0, 0, 3, 0, 6]`
### Graphs Explanation:
- **Dynamic Array:**
- Both objects use a dynamic array
![The image contains information about polynomial expressions and their operations. Here's the transcription suitable for an educational website:
---
**Polynomial Operations Example**
We are given two polynomials, \( A \) and \( B \), and we will explore their addition and subtraction.
**Polynomial A:**
\[ A = 4x^7 + 5x^6 + 3x^3 + 2x^1 + 8x^0 \]
- **Object A:**
- Coefficients: \( [0, 0, 0, 3, 0, 0, 5, 4] \)
- Degree: 7
**Polynomial B:**
\[ B = 6x^8 + 3x^6 + 4x^5 + 8x^3 + 3x^1 + 2x^0 \]
- **Object B:**
- Coefficients: \( [2, 3, 0, 8, 0, 4, 3, 0, 6] \)
- Degree: 8
**Addition of Polynomials (A + B):**
The result of adding polynomials \( A \) and \( B \) is:
\[ 6x^8 + 4x^7 + 8x^6 + 4x^5 + 11x^3 + 5x^1 + 10x^0 \]
- Combined Coefficients: \( [10, 5, 0, 11, 0, 4, 8, 4, 6] \)
- Resulting Degree: 8
**Subtraction of Polynomials (A - B):**
The result of subtracting polynomial \( B \) from \( A \) is:
\[ 6x^8 + 4x^7 + 2x^6 - 4x^5 - 5x^3 - x^1 + 6x^0 \]
- Combined Coefficients: \( [6, -1, 0, -5, 0, -4, 2, 4, 6] \)
- Resulting Degree: 8
**Explanation of the Tables:**
The tables display the coefficients for each power of x, starting from \( x^0 \) up to \( x^8 \), where applicable. The degree](https://content.bartleby.com/qna-images/question/cdbdf4c8-5459-45de-99f8-cd8d71031beb/07b52563-37af-444b-b3f9-8e1bb7705e03/olds88_thumbnail.png)
Transcribed Image Text:The image contains information about polynomial expressions and their operations. Here's the transcription suitable for an educational website:
---
**Polynomial Operations Example**
We are given two polynomials, \( A \) and \( B \), and we will explore their addition and subtraction.
**Polynomial A:**
\[ A = 4x^7 + 5x^6 + 3x^3 + 2x^1 + 8x^0 \]
- **Object A:**
- Coefficients: \( [0, 0, 0, 3, 0, 0, 5, 4] \)
- Degree: 7
**Polynomial B:**
\[ B = 6x^8 + 3x^6 + 4x^5 + 8x^3 + 3x^1 + 2x^0 \]
- **Object B:**
- Coefficients: \( [2, 3, 0, 8, 0, 4, 3, 0, 6] \)
- Degree: 8
**Addition of Polynomials (A + B):**
The result of adding polynomials \( A \) and \( B \) is:
\[ 6x^8 + 4x^7 + 8x^6 + 4x^5 + 11x^3 + 5x^1 + 10x^0 \]
- Combined Coefficients: \( [10, 5, 0, 11, 0, 4, 8, 4, 6] \)
- Resulting Degree: 8
**Subtraction of Polynomials (A - B):**
The result of subtracting polynomial \( B \) from \( A \) is:
\[ 6x^8 + 4x^7 + 2x^6 - 4x^5 - 5x^3 - x^1 + 6x^0 \]
- Combined Coefficients: \( [6, -1, 0, -5, 0, -4, 2, 4, 6] \)
- Resulting Degree: 8
**Explanation of the Tables:**
The tables display the coefficients for each power of x, starting from \( x^0 \) up to \( x^8 \), where applicable. The degree
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In c++ please help me answer this question I will give you a good rating :) implement the three versions of the addupto20() function: iterative, recursive, and divide-and-conquer approach test these functions with a few inputs from your main() Iterative solution * check if there exsits two numbers from vector data that add up to 20 e.g., if data=[2,5,3,15], the function returns true, as data [0] +data [3]==20 e.g., if data=[3,4,0,8], the function return false precondition: vector data has been initialized postcondition: if there are two numbers from list add up to 20, return true; otherwise, return false */ bool AddupTo20 (const vector‹int> & data){ } Come up with a recursive solution to the problem, following the hints given below: * check if there exsits two numbers from vector data[first...right] add up to 20 e.g., if data=[2,5,3,15], first=0, last=3, the function returns true, as data [0] +data [3]==20 e.g., if data=[2,5,3,15], first=2, last=3, the function returns…arrow_forwardIn C++, what is the difference between emplace_back and push_back, when using vectors? I looked at other sources, and still find it confusing as to what s the difference between the two of these.arrow_forwardExplain the pointer soting with help of pseudo code?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
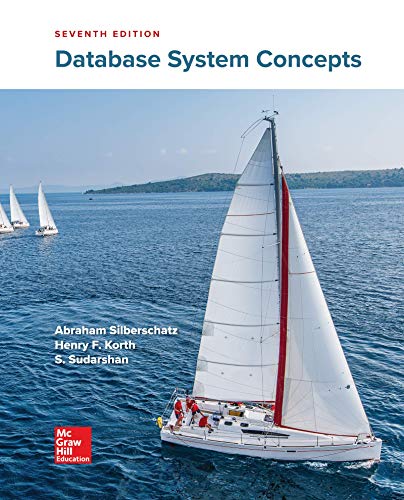
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
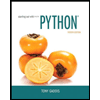
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
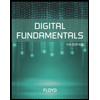
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
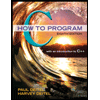
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
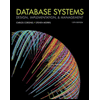
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
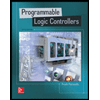
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education