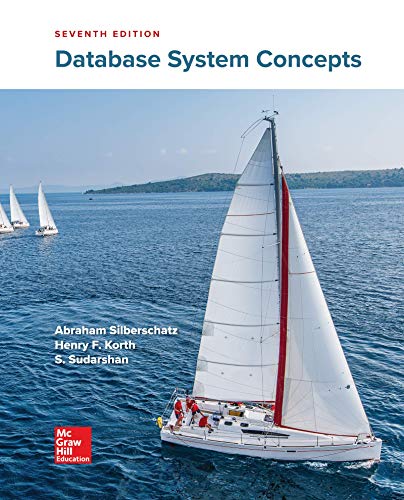
use only C#
-
Write a method call CubeIt(int x, ref int cube) that takes two arguments and does not return a value. The method will cube the first argument and assign it to the second argument.
In your main, call this method twice and print the value of the parameters after each method call. -
Write a method with the following header: static void CalculateTuitionFee(int numberOfCourses, double costPerCourse, ref double fees). This method will calculate and assign the required fees amount to the third argument. [Fees = number of courses * cost per course + 15.25].
From your program Main() method, call the CalculateTuitionFee () method four times supplying different arguments each time and display the value of the third argument after each method call. -
Write a method that takes four parameter of type int. The method will assign the sum of the first two arguments to the third and the difference of the first two to the fourth. This method should be coded so that the calling method will use the value of the third and fourth parameters.
Call this method about three times and print out the value of the four parameters after each method call.The argument is taken as degrees
-
Write a method with header static void CalculateTrigValues(double degrees, out double sine, out double cosine, out double tangent). The method will use the first argument to compute the values of the other three arguments. Used the method Math.Sin, Math.Cos and Math.Tan to compute the second to fourth arguments respectively. [radians = degrees * Math.Pi /180].
In the Main() method, invoke this method 20 times with the first argument taking the values 0, 5, 10, … 95 and display the four arguments in a professional tabular format.The argument is taken as radians
-
Write a method that takes three parameters of type double: the first represents an angle, the other two represents the sine and cosine of the angle respectively. The method must be able to change the actual value of the second and third argument.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Java Programarrow_forwardIN JAVA "New Employee Gross Salary Calculator" Write a method that accepts an employee's yearly salary and start date and calculates their gross salary from the provided start date up through the end of the year Employees always start on the first of the month Throw an IllegalArgumentException if the provided start date is not the first of the month Employees qualify for a 3% pay raise 3 months after their start date and another 6% 3 months after that Use appropriate data typearrow_forwardIn Java, a global variable is declared inside a method and is accessible only in that method. -True -Falsearrow_forward
- Financial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardWrite java code that recursively calculates the Sum of the array cells. Program requirements: Create an array named MyArr and initialize it as follow: 19 27 81 243 88 1- Write a method named ArrSum that recursively calculates the Sum of the numbers in the Array and returns the Sum of the numbers. Hint: Method with 1 parameter (the Array) that returns 1 value (the Array's Sum). 2- Write a method named Display that displays the Array contents and Sum of the numbers. Hint: Method with 2 parameters (the Array) and Sum with no returns (void) 3- Your program output format must be the same as the following: =>> Array Summation Array: 31 19 27 81 243 88 100 6 10 5 Sum: 610 =>> End of the Program Documentation / Comments 31 100 6 10 5arrow_forwardIn this program, create a method with the following signature: public static double getAverage(double d1, double d2, double d3, double d4, double d5) This method will accept five doubles and return the average. Also in program, create a method with the following signature: public static char determineGrade(double avg) This method will take an average, then return the corresponding letter grade. Write in java and use test case# as an example.arrow_forward
- C# Question Write a statement that declares an array of integers called lotteryPicks and assigns it to the value returned from calling a method named FillArray. The method takes a 3 value parameters, a string called prompt and 2 integers called low and high. Use the following literal values, “lottery numbers”, 1 and 69 as actual parameters in the callarrow_forwardNeed help with this java program.arrow_forward2please write in C#arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
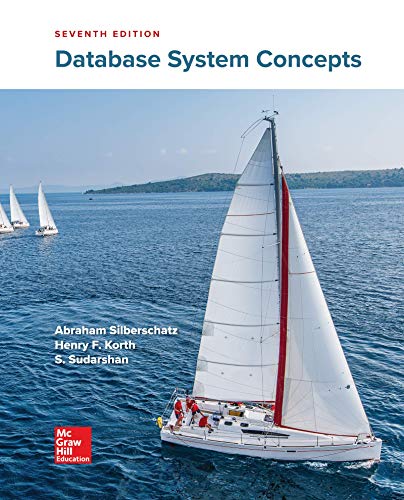
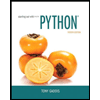
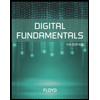
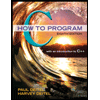
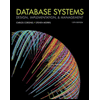
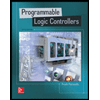