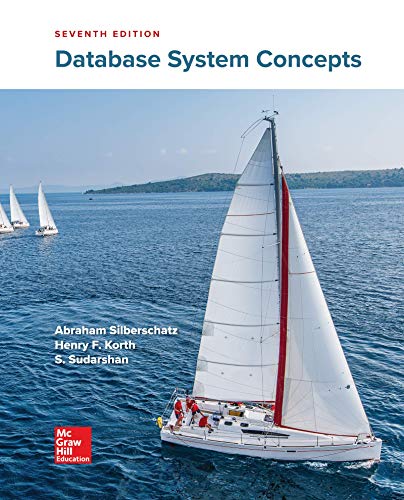
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
--- MATLAB work for Jacobi Iteration ---
--- Please answer the question in the attached image by replacing the ### with matlab script ---
--- Thank you in adavance ---
![Use element-by-element Jacobi iteration to solve the system of equations Ax = b where
6
1-51
A-12 7
0-21
10
-1-2-31
2
H
10
and b =
3
2
You will be assessed on whether you've defined A and B correctly, whether the test for diagonal dominance is correct, whether one of the intermediate steps has been computed
correctly, and whether the final step count and answer for x is correct.
Make use of Matlab's help file to understand the functions being used.
Script
1
2 %% Solving a matrix equation Ax=b using the Jacobi iterative technique.
3 % % The methods require A to be square and none of its main diagonal
4 %% elements can be zero.
5
6 A = ###
7 b = ###
%% set b as a column vector
8
9 [# # #,N] = size(A);
%%% M = number of rows, N = number of columns
10 if M~=N
11
error('A must be a square matrix')
12 end
13
14 %%% Test for diagonal dominance
15 %%% Check if the absolute value of the main diagonal elements is greater than
16 %%% the sum of the absolute value of the remaining terms in each row.
17 rowtest = abs(diag(A)) > sum(abs (A-diag(diag(A))),###);
18 index
find (rowtest == ###);
19 if isempty (index)
20
21
22
23 end
24
disp("The matrix is not diagonally dominant.")
disp("Take the results with caution.")
pause(2)
25 % % The iterative process
26 x [0; 0; 0; 0; 0];
27 xe = X
28
29 reldiff_tolerance = ###;
30 reldiff = 100;
31 k = 0;
32
% % The initial guess.
%% setup another vector to store the new data
%% set a tolerance of 10^-6 for the relative difference
%% set reldiff arbitrarily high to begin
%% to keep count of the number of iterations
33 fprintf('0, [%.6f, %.6f, %.6f, %.6f, %.6f]\n',x(1),x(2),x(3),x(4),x(5));
34 while ### > ###
x0(1) = (b(1)-A(1,2:M)*x(2:M))/A(1,1); %% all terms are to the right
for j = 2
M-1
terms] = A(j,1:j-1)*x(1:j-1); %% terms to the left of the main diagonal
termsr = ###; %% terms to the right of the main diagonal
x0(j)
(b(j)-termsl-termsr)/A(j,j);
35
36
37
38
39
40
end
41
42
43
k = k+1;
44
x = ###3
45
if k == 10
46
x10 = ###3
47
end
x0(M) = (b(M)-A(M,1:M-1)*x(1:M-1))/A(M,M); % % all terms are to the left
reldiff = abs(norm(x-x0))/norm(x0);
%% increment the iteration count
%% overwrite the old data with the new data
%% automatically store the value of x when k=10
48 fprintf("%d, [%.6f, %.6f, %.6f, %.6f, %.6f]\n',k,x(1),x(2),x(3),x(4),x(5));
49 end
50 xend ###;
%% store the final value of x
51 kend ###;3
%% store the total number of iterations
52
53
Save
CReset
MATLAB Documentation
► Run Script
?](https://content.bartleby.com/qna-images/question/2476f823-c3c8-40b1-886d-66e8e64f59f9/1271f120-8aa6-4aa9-92f2-a16fed2eae1c/sfjuksa_thumbnail.png)
Transcribed Image Text:Use element-by-element Jacobi iteration to solve the system of equations Ax = b where
6
1-51
A-12 7
0-21
10
-1-2-31
2
H
10
and b =
3
2
You will be assessed on whether you've defined A and B correctly, whether the test for diagonal dominance is correct, whether one of the intermediate steps has been computed
correctly, and whether the final step count and answer for x is correct.
Make use of Matlab's help file to understand the functions being used.
Script
1
2 %% Solving a matrix equation Ax=b using the Jacobi iterative technique.
3 % % The methods require A to be square and none of its main diagonal
4 %% elements can be zero.
5
6 A = ###
7 b = ###
%% set b as a column vector
8
9 [# # #,N] = size(A);
%%% M = number of rows, N = number of columns
10 if M~=N
11
error('A must be a square matrix')
12 end
13
14 %%% Test for diagonal dominance
15 %%% Check if the absolute value of the main diagonal elements is greater than
16 %%% the sum of the absolute value of the remaining terms in each row.
17 rowtest = abs(diag(A)) > sum(abs (A-diag(diag(A))),###);
18 index
find (rowtest == ###);
19 if isempty (index)
20
21
22
23 end
24
disp("The matrix is not diagonally dominant.")
disp("Take the results with caution.")
pause(2)
25 % % The iterative process
26 x [0; 0; 0; 0; 0];
27 xe = X
28
29 reldiff_tolerance = ###;
30 reldiff = 100;
31 k = 0;
32
% % The initial guess.
%% setup another vector to store the new data
%% set a tolerance of 10^-6 for the relative difference
%% set reldiff arbitrarily high to begin
%% to keep count of the number of iterations
33 fprintf('0, [%.6f, %.6f, %.6f, %.6f, %.6f]\n',x(1),x(2),x(3),x(4),x(5));
34 while ### > ###
x0(1) = (b(1)-A(1,2:M)*x(2:M))/A(1,1); %% all terms are to the right
for j = 2
M-1
terms] = A(j,1:j-1)*x(1:j-1); %% terms to the left of the main diagonal
termsr = ###; %% terms to the right of the main diagonal
x0(j)
(b(j)-termsl-termsr)/A(j,j);
35
36
37
38
39
40
end
41
42
43
k = k+1;
44
x = ###3
45
if k == 10
46
x10 = ###3
47
end
x0(M) = (b(M)-A(M,1:M-1)*x(1:M-1))/A(M,M); % % all terms are to the left
reldiff = abs(norm(x-x0))/norm(x0);
%% increment the iteration count
%% overwrite the old data with the new data
%% automatically store the value of x when k=10
48 fprintf("%d, [%.6f, %.6f, %.6f, %.6f, %.6f]\n',k,x(1),x(2),x(3),x(4),x(5));
49 end
50 xend ###;
%% store the final value of x
51 kend ###;3
%% store the total number of iterations
52
53
Save
CReset
MATLAB Documentation
► Run Script
?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- Explain the advantages and disadvantages of sharding, as well as the possible concerns.arrow_forwardwhat are the Differentiate between coding, documentation problemarrow_forward1. Compute the Laplace transform of the following equations via MATLAB. Tips: O First, you have to declare the symbolic variable names that you will be using via the MATLAB command syms. Use the MATLAB help to know more about this command and its syntax. o Write the function. o Perform the laplace transform on the function. Question 1: x(t) = sin(t) Question 2: x(t) = 5-et 4√3arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
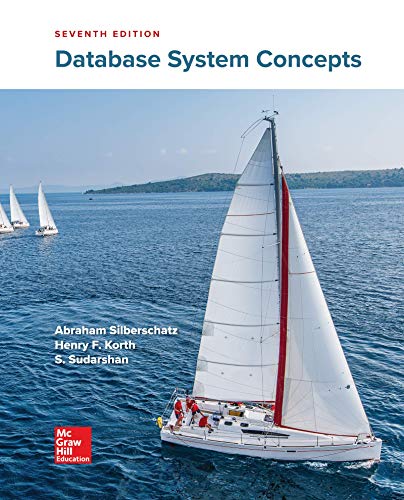
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
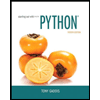
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
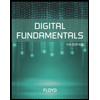
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
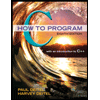
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
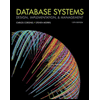
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
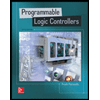
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education