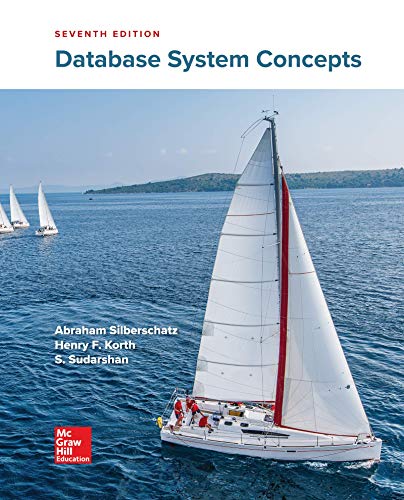
Use Clojure:
a. See attached picture.
b. In Clojure (like other functional programming languages) functions and variables are treated identically. This means a function may easily take another function as an argument, and/or return a function. Write a function swap-arg-order which takes a function (of two arguments) as an argument returns another function that does the same thing, but expects its two arguments in the opposite order.
That is, for example
• given the division function / which divides the first argument by the second (so (/ 3 6) returns the number 1/2), the following expression should evaluate to 2
((swap-arg-order /) 3 6)
• given the function list-longer-than? from above, the following expression should evaluate to true
((swap-arg-order list-longer-than?) '(1 2 3) 2)
c. Define a higher order function g so the following expression evaluates to true:
(= 100 (g (fn [n] (* n n))))


Step by stepSolved in 3 steps

- Call by value in programming means that a copy of variable value is pased from function calling part to function definition part. Call by reference means that an address of variables are passed from calling part to function definition. arrow_forward Step 2 Call by reference is used to avoid extra memory usage because in call by reference same variables addresses are passed. Any changes made will reflect back. So it doesn't require extra variable to hold data. Call by value in programming is used where memory storage is not concern. Scenario in which actual value must not be disturbed then in these type of cases, call by value is used. We can't use only one because user requirement changes. Sometimes user tella actual data should be preserved and he/she want any data processing, in such scenario programmers use call by value. Some users only want calculated result. In such cases, programmers use call by reference to preserve memory usage. So it is totally dependent on user…arrow_forwardneed help in C++ Problem: You are asked to create a program for storing the catalog of movies at a DVD store using functions, files, and user-defined structures. The program should let the user read the movie through the file, add, remove, and output movies to the file. For this assignment, you must store the information about the movies in the catalog using a single vector. The vector's data type is a user-defined structure that you must define on functions.h following these rules: Identifier for the user-define structure: movie. Member variables of the structure "movie": name (string), year (int), and genre (string). Note: you must use the identifiers presented before when defining the user-defined structure. Your solution will NOT pass the unit test cases if you do not follow the instructions presented above. The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). The following user-defined functions are…arrow_forwardChoose if each of the following is true or false:Dynamically binding data to virtual functions is limited to pointers and references.arrow_forward
- Consider the following function definition.void mystery(int* x){// function body } Inside the definition of mystery, the C++ language provides a way to distinguish if x points to a lone int value or to an int allocated as part of a larger array.A. True B. Falsearrow_forwardConsider the function definition: void GetNums(int howMany, float& alpha, float& beta) { int i; beta = 0; for (i = 0; i < howMany; i++) { beta = alpha + beta; } } Describe what happens in MEMORY when the GetNums function is called.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
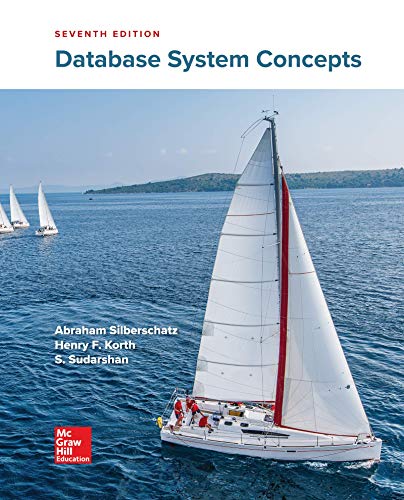
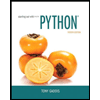
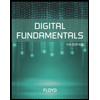
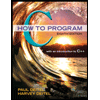
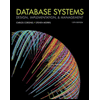
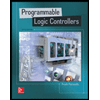