Use C++ code and draw a flow chart. I only want the FLOWCHART, not the code. Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Each one of the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Project Specifications Input for this project: Values of the grid (row by row) Output for this project: Whether or not the grid is a magic square Programmer’s full name Project number Project due date
Use C++ code and draw a flow chart. I only want the FLOWCHART, not the code.
Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type.
Each one of the arrays corresponds to a row of the magic square.
The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not.
Project Specifications
Input for this project:
- Values of the grid (row by row)
Output for this project:
- Whether or not the grid is a magic square
- Programmer’s full name
- Project number
- Project due date
Processing Requirements
Use the following template to start your project:
#include<iostream>
using namespace std;
// Global constants
const int ROWS = 3; // The number of rows in the array
const int COLS = 3; // The number of columns in the array
const int MIN = 1; // The value of the smallest number
const int MAX = 9; // The value of the largest number
// Function prototypes
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void showArray(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main()
{
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding to each row of the grid */
int magicArrayRow1[COLS], magicArrayRow2[COLS], magicArrayRow3[COLS];
// Your code goes here
return 0;
}
// Function definitions go here
Create and use the following functions:
- void fillArray(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size) - Accepts 3 int arrays and size as arguments, and fills the arrays out with values entered by the user. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square
- void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and displays their content.
Example:
1 3 5 (arrayRow1)
6 7 9 (arrayRow2)
8 2 4 (arrayRow3)
- bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and a size as arguments and returns true if all the requirements of a magic square are met. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max) - accepts 3 int arrays, a size, and a min and max value as arguments and returns true if the values in the arrays are within the specified range min and max. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments, and returns true if the values in the arrays are unique (only one occurrence of numbers between 1-9). Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the rows are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the columns are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.
- bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size) - accepts 3 int arrays and size as arguments and returns true if the sum of the values in each of the array's diagonals are equal. Otherwise, it returns false. The first argument corresponds to the first row of the magic square, the second argument to the second row, and the third argument to the third row of the magic square.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

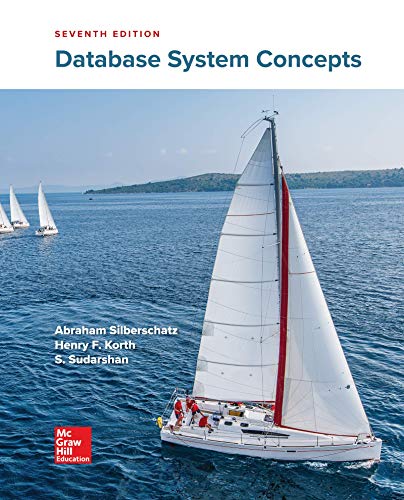
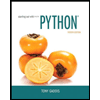
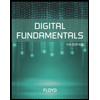
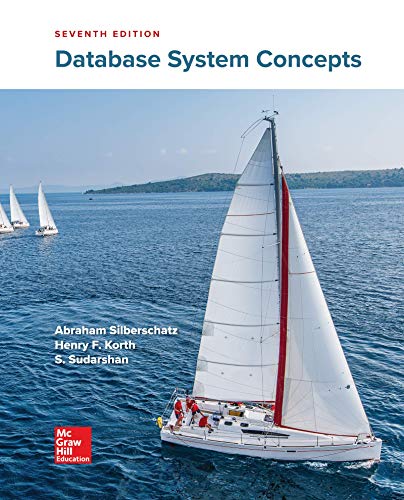
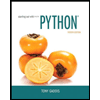
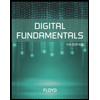
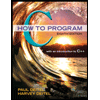
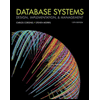
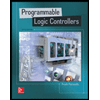