USE C++ Add your solution to the provided code template. Use descriptive variable names. Avoid single letter names when possible. DO NOT use == true or == false in your boolean expressions (see lecture notes). DO NOT use explicit type casting. Instead use coercion (see lecture notes). DO NOT unnecessarily use parenthesis in an expression, e.g. an equation or formula. Parenthesis should only be used for grouping portions of an expression to change operator precedence order. For example, parenthesis are unnecessary in the expression (a + b + c). Instead use a + b + c. Parenthesis are necessary in the expression (a + b + c) / 3. Your program must be readable including indenting, spaces, and avoid lines that are too long. Use the the sample programs in the lecture notes as a guide. Comment your program. Read the document at the "Lecture" link on Carmen under Modules->Commenting Your Program-> Commenting your program. DO NOT comment every line. Only use C++ statements presented in the course. I.e. statements and notation presented in the lecture notes and assigned readings. Important!: Write your code incrementally. This means implement your solution one portion at a time where you compile, run, and test the code portion before moving on to the next portion. Use the provided test cases to help you arrive at your final solution. All values are type double with the exception that the length and height of the rectangle are type int values. Assume that the user will enter positive values for the rectangle length and height. TASK 1: Study the lecture notes (Powerpoint slides and pre-recorded lectures) and assigned readings on Selection before you start. TASK 2: Replace "??" with your name, creation date, and a description of the program (synopsis). NOTE: DO NOT delete nor change the code already given to you in the code template, except for the comment /* INSERT YOUR CODE HERE */. You will insert your solution by replacing /* INSERT YOUR CODE HERE */. TASK 3: Write C++ code to prompt and read in the x and y coordinates of the first circle. Compile, run, and test this solution before continuing to the next task. TASK 4: Write C++ code to prompt and read in the radius of the first circle. Compile, run, and test this solution before continuing to the next task. TASK 5: Write C++ code to prompt and read in the x and y coordinates of the bottom left corner of the rectangle. Compile, run, and test this solution before continuing to the next task. Continue to do this after EACH of the following tasks. TASK 6: Write C++ code to prompt and read in the length and height of the rectangle. TASK 7: Write C++ code to prompt and read in the x and y coordinates of the second circle. TASK 8: Write C++ code to prompt and read in the radius of the second circle. TASK 9: Write C++ code to prompt and read in the x and y coordinates of the query point. Task 10: Write C++ code to determine which shapes contain the query point and output the correct message. i. Use the Pythagorean Theorem (see examples in the lecture notes) to determine whether a circle contains the query point with the equation square root{(x - cx)^2 + (y - cy)^2} <= r, where (x, y) is the query point, (cx, cy) is the circle center, r is the circle radius, and ^ means to take a value to a power. Though, the ^ is NOT a valid operator in C++. ii. Use two checks to determine whether the rectangle contains the query point. Check that the x coordinate of the query point is between the x locations of the left and right sides of the rectangle. Also, check that the y coordinate of the query point is between the y locations of the bottom and top sides of the rectangle. Let (rx, ry) be the coordinates for the bottom left corner of the rectangle, l be the length, and h be the height. The x location of the left side is rx. The x location of the right side is rx + l. The y location of the bottom side is ry. The y location of the top side is ry + h. iii. Output one of eight possible messages: All shapes contain point, Both circles contain point, 1st circle and rectangle contain point, 1st circle contains point, 2nd circle and rectangle contain point, 2nd circle contains point, Rectangle contains point, or No shape contains point. Be sure that there is a comment documenting each variable (see the document on Carmen under Modules on how to comment your code). Do not start a variable name with a capital letter. Be sure that your code is properly indented, readable, and use good descriptive names.
- USE C++
- Add your solution to the provided code template.
- Use descriptive variable names. Avoid single letter names when possible.
- DO NOT use == true or == false in your boolean expressions (see lecture notes).
- DO NOT use explicit type casting. Instead use coercion (see lecture notes).
- DO NOT unnecessarily use parenthesis in an expression, e.g. an equation or formula. Parenthesis should only be used for grouping portions of an expression to change operator precedence order. For example, parenthesis are unnecessary in the expression (a + b + c). Instead use a + b + c. Parenthesis are necessary in the expression (a + b + c) / 3.
- Your program must be readable including indenting, spaces, and avoid lines that are too long. Use the the sample programs in the lecture notes as a guide.
- Comment your program. Read the document at the "Lecture" link on Carmen under Modules->Commenting Your Program-> Commenting your program. DO NOT comment every line.
- Only use C++ statements presented in the course. I.e. statements and notation presented in the lecture notes and assigned readings.
Important!: Write your code incrementally. This means implement your solution one portion at a time where you compile, run, and test the code portion before moving on to the next portion. Use the provided test cases to help you arrive at your final solution.
All values are type double with the exception that the length and height of the rectangle are type int values. Assume that the user will enter positive values for the rectangle length and height.
TASK 1: Study the lecture notes (Powerpoint slides and pre-recorded lectures) and assigned readings on Selection before you start.
TASK 2: Replace "??" with your name, creation date, and a description of the program (synopsis).
NOTE: DO NOT delete nor change the code already given to you in the code template, except for the comment /* INSERT YOUR CODE HERE */. You will insert your solution by replacing /* INSERT YOUR CODE HERE */.
TASK 3: Write C++ code to prompt and read in the x and y coordinates of the first circle. Compile, run, and test this solution before continuing to the next task.
TASK 4: Write C++ code to prompt and read in the radius of the first circle. Compile, run, and test this solution before continuing to the next task.
TASK 5: Write C++ code to prompt and read in the x and y coordinates of the bottom left corner of the rectangle. Compile, run, and test this solution before continuing to the next task. Continue to do this after EACH of the following tasks.
TASK 6: Write C++ code to prompt and read in the length and height of the rectangle.
TASK 7: Write C++ code to prompt and read in the x and y coordinates of the second circle.
TASK 8: Write C++ code to prompt and read in the radius of the second circle.
TASK 9: Write C++ code to prompt and read in the x and y coordinates of the query point.
Task 10: Write C++ code to determine which shapes contain the query point and output the correct message.
i. Use the Pythagorean Theorem (see examples in the lecture notes) to determine whether a circle contains the query point with the equation square root{(x - cx)^2 + (y - cy)^2} <= r, where (x, y) is the query point, (cx, cy) is the circle center, r is the circle radius, and ^ means to take a value to a power. Though, the ^ is NOT a valid operator in C++.
ii. Use two checks to determine whether the rectangle contains the query point. Check that the x coordinate of the query point is between the x locations of the left and right sides of the rectangle. Also, check that the y coordinate of the query point is between the y locations of the bottom and top sides of the rectangle. Let (rx, ry) be the coordinates for the bottom left corner of the rectangle, l be the length, and h be the height. The x location of the left side is rx. The x location of the right side is rx + l. The y location of the bottom side is ry. The y location of the top side is ry + h.
iii. Output one of eight possible messages: All shapes contain point, Both circles contain point, 1st circle and rectangle contain point, 1st circle contains point, 2nd circle and rectangle contain point, 2nd circle contains point, Rectangle contains point, or No shape contains point.
Be sure that there is a comment documenting each variable (see the document on Carmen under Modules on how to comment your code).
Do not start a variable name with a capital letter. Be sure that your code is properly indented, readable, and use good descriptive names.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

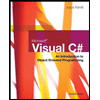
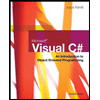