Under your module imports, paste the following definitions: (define WIDTH 300) (define HEIGHT 160) (define ES (empty-scene WIDTH HEIGHT)) (define (next-size s) (/ s 2)) Next, write a tail-call recursive function called circles. This function should take two numbers, x and size, and a Scene scn. If size is less-than or equal to 2, then your function returns the scn unchanged (base case). Otherwise, your function should place a circle in the Scene that results from a recursive call to circles with x shifted by (+ size (next-size size)), a new size of (next-size size), and using the original Scene. After evaluating (circles 100 48 ES), your function should output this image of five blue circles, in this order, with the first circle at (x,y) coordinate position (100, 80):
Under your module imports, paste the following definitions:
(define WIDTH 300)
(define HEIGHT 160)
(define ES (empty-scene WIDTH HEIGHT))
(define (next-size s) (/ s 2))
Next, write a tail-call recursive function called circles. This function should take two numbers, x and size, and a Scene scn. If size is less-than or equal to 2, then your function returns the scn unchanged (base case). Otherwise, your function should place a circle in the Scene that results from a recursive call to circles with x shifted by (+ size (next-size size)), a new size of (next-size size), and using the original Scene. After evaluating (circles 100 48 ES), your function should output this image of five blue circles, in this order, with the first circle at (x,y) coordinate position (100, 80):


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

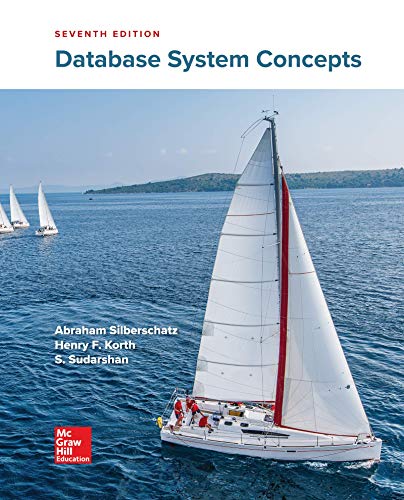
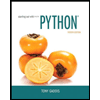
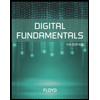
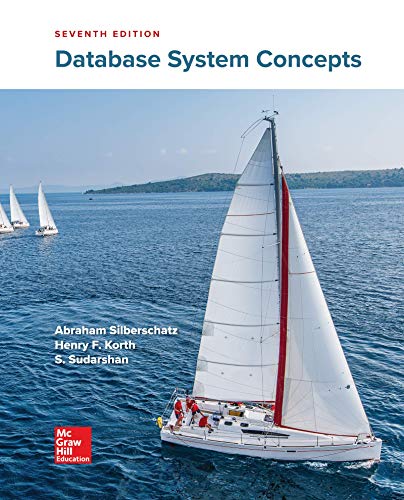
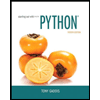
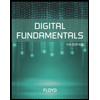
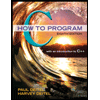
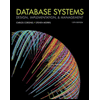
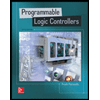