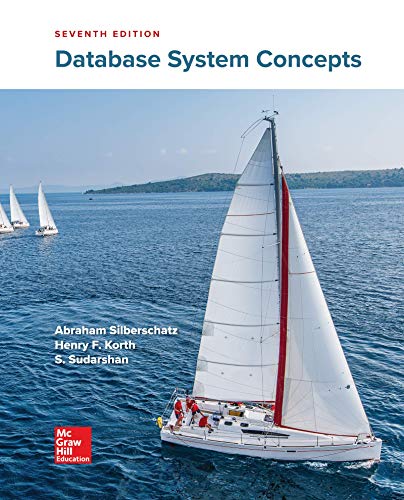
Two two-dimensional arrays m1 and m2 are strictly identical if their corresponding elements are equal. Write a function that returns true if m1 and m2 are strictly identical, using the following header:
const int SIZE = 3;
bool equals(int m1[][SIZE], int m2[][SIZE], int rowSize)
Write a test program that prompts the user to enter two 3x3 arrays of integers and displays whether the two are strictly identical. Here are the sample runs.
<Output>
Enter list1: 51 22 25 6 1 4 24 54 6
Enter list2: 51 22 25 6 1 4 24 54 6
Two arrays are strictly identical
<End Output>
<Output>
Enter list1: 51 25 22 6 1 4 24 54 6
Enter list2: 51 22 25 6 1 4 24 54 6
Two arrays are not strictly identical
C++

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- 5. Write a function which will find the average of all elements of an array of integers. Pass an array and a dimension (the number of elements), and return the value of the average as a float ( the return value). Test this function in the main program with the array: 5, 7, 3, 5, 6, 4.arrow_forwardComplete the code.arrow_forwardIn computational geometry, often you need to find the rightmost lowest point in a set of points. Write the following function that returns the rightmost lowest point in a set of points. const int SIZE = 2;void getRightmostLowestPoint(const double points[][SIZE], int numberOfPoints, double rightMostPoint[]); Write a test program that prompts the user to enter the coordinates of six points and displays the rightmost lowest point.arrow_forward
- Write a program that uses a two-dimensional array to store the highest and lowest temperatures for each month of the year. The program should output the average high, average low, and the highest and lowest temperatures for the year. Your program must consist of the following functions: Part a: Function getData: This function reads and stores data in the two- dimensional array. Part b: Function averageHigh: This function calculates and returns the aver- age high temperature for the year. Part c: Function averageLow: This function calculates and returns the average low temperature for the year. Part d: Function indexHighTemp: This function returns the index of the highest high temperature in the array. Part e: Function indexLowTemp: This function returns the index of the lowest low temperature in the array. (These functions must all have the appropriate parameters.) #include <iostream> using namespace std; const int NO_OF_MONTHS = 12; void getData(int twoDim[][2], int…arrow_forwardWrite a program that uses a two-dimensional array to store the highest andlowest temperatures for each month of the year. The program shouldoutput the average high, average low, and the highest and lowest temperaturesfor the year. Your program must consist of the following functions:a. Function getData: This function reads and stores data in the twodimensionalarray.b. Function averageHigh: This function calculates and returns theaverage high temperature for the year.c. Function averageLow: This function calculates and returns the averagelow temperature for the year.d. Function indexHighTemp: This function returns the index of thehighest high temperature in the array.e. Function indexLowTemp: This function returns the index of thelowest low temperature in the array.arrow_forwardHello, can you please help me do this Java program using the two attached pics, here are the instructions: Problem Description:Use a one-dimensional array to solve the following problem:Request five unique numbers, each of which is between 10 and 100, inclusively. As each number is read,display it only if it is not a duplicate of a number already read, providing for the “worst case”, which is allfive numbers are different.Use the smallest possible array to solve this problem. Display the complete set of unique values inputafter the user inputs each new value.Programming Steps1. Download the provided programs Project7_UniqueNumbers.java andProject7_UniqueNumbersTest.java2. Complete the programs to use arrays in your compilera. There are nine (9) TODOsi. Seven (7) in Project7_UniqueNumbers.javaii. Two (2) in Project7_UniqueNumbersTest.javab. You should add necessary statements/structures to the given programs as instructed inthe comments3. Debug and run your applicationarrow_forward
- The following program is supposed to let the end-user enters 10 numbers in a one dimensional array. The program must then check the input numbers in the following order and way: 1f the input number is a negative one, the program should multiply that number by (5), and return the number of negative elements in the array. 2. if the input number is a positive one, the program should multiply that number by (2), and return the number of positive elements in the array. 3. if the input number is a zero, the program should return the number of zeros in the array The program code is given below with some missing code. You are required to write the missing code ONLY in order to make the program complete. Sample Input/Output Enter 10 numbers in the array -8 -16 16 80 2 12 -12 -2 0 -40 -80 32 16 0 4 24 -60 -10 0 The number of negative elements is: 4 The number of positive elements is: 4 The number of zeros is: 2 dinclude using namespace std; int main() int input[10], i, negative-0, positive-0,…arrow_forwardProgramming in C language.arrow_forwardDebugging challenge: Consider the code example below. This program is designed to compare the contents of two arrays: if they are equal, the program should display "TRUE," if the arrays are not equal, the program should display "FALSE". However, even when the arrays have the same value, the program always prints "FALSE", regardless of the values in arrays a and b. What is going on here? What about the program causes the comparison to always fail? int a[4] {1, 2, 3, 4}; int b[4] = {1, 2, 3, 4}; if (a == b) { display ("TRUE"); else { display ("FALSE");arrow_forward
- In C++ language Write a function that takes a 1 Demensional array and an integer n and reutrns the number of times 'n' appears in the array. If 'n' does not appear in the array, return -1.arrow_forwardC++arrow_forwardLo Shu Magic Square C++ The Lo Shu Magic Square is a grid with 3 rows and 3 columns. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 through 9, each cell is unique. The sum of each row, each column, and each diagonal all add up to the same number. Write a program that creates a 2D array of size 3x3, and fills each cell withn random, unique values betweem 1 and 9. Print the values. Determine if the 2D array is a Lo Shu Magic Square and print the results. Sample Output Run the program when the 2D array is a lo shu square. A couple runs of the program where it is NOT a Lo Shu Magic Square. The program declares and initializes a 2D array of size 3,3 and fills it with random, unique values. The program prints the values correctly. The program determines whether or not the 2D array is a Lo Shu Magic Square and prints the results. The program must use at least one function besides main for credit.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
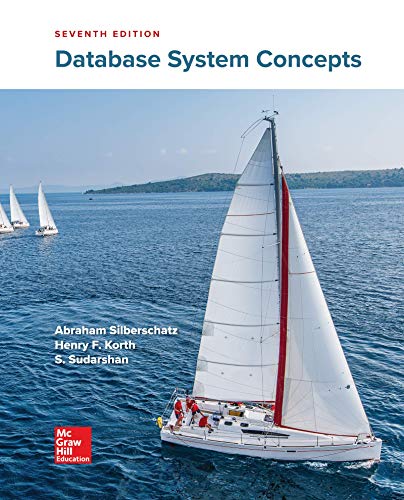
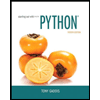
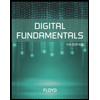
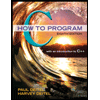
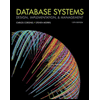
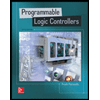