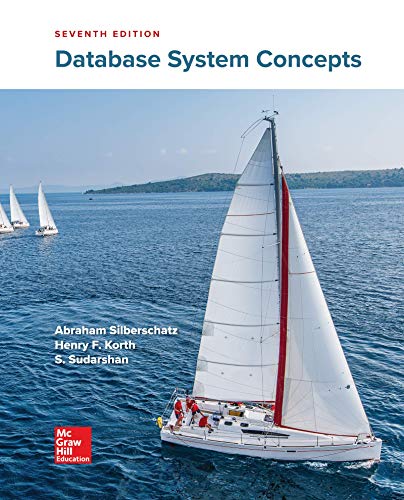
Concept explainers
Turn this C
Files: main.c, matrix.h, matrix.c
Code:
#include <stdio.h>
#define ROW 6
#define COL 6
//function prototypes
void add(int matrixResult[][COL],int matrixA[]
[COL],int matrixB[][COL],int row,int col);
void subtract(int matrixResult[][COL],int matrixA[]
[COL],int matrixB[][COL],int row,int col);
void multiply(int matrixResult[][COL],int matrixA[]
[COL],int matrixB[][COL],int row,int col);
void display(int matrix[][COL]);
//main function
int main()
{
//declare and initialize 6*6 matrix
int matrixA[][COL] = {{10,20,30,40,50,60},{7,8,9,10,11,12},
{13,14,15,16,17,18},{19,20,21,22,23,24},
{25,26,27,28,29,30},{31,32,33,34,35,36}};
//declare and initialize 6*6 matrix
intmatrixB[][COL] = {{1,2,3,4,5,6},{17,28,19,78,34,12},
{33,14,25,16,17,18},{19,22,21,22,23,24},
{19,26,11,28,29,30},{30,32,23,34,15,16}};
int matrixResult[ROW][COL];
printf("Addition of two matrices:\n");
add(matrixResult,matrixA,matrixB,ROW,COL);
printf("\n\nDifference of two matrices:\n");
subtract(matrixResult,matrixA,matrixB,ROW,COL);
printf("\n\nProduct of two matrices:\n");
multiply(matrixResult,matrixA,matrixB,ROW,COL);
return 0;
}
//function to add two 6*6 matrix
void add(int matrixResult[][COL],int matrixA[][COL],
int matrixB[][COL],int row,int col)
{
for(int i=0;i<row;i++)
for(int j=0;j<col;j++)
matrixResult[i][j] = matrixA[i][j] + matrixB[i][j];
display(matrixResult);
}
//function to subtract two 6*6 matrix
void subtract(int matrixResult[][COL],int matrixA[][COL],
int matrixB[][COL],int row,int col)
{
for(int i=0;i<row;i++)
for(int j=0;j<col;j++)
matrixResult[i][j] = matrixA[i][j] - matrixB[i][j];
display(matrixResult);
}
//function to multiply two 6*6 matrix
void multiply(int matrixResult[][COL],int matrixA[][COL],
int matrixB[][COL],int row,int col)
{
for(int i=0;i<row;i++)
{
for(int j=0;j<col;j++)
{
matrixResult[i][j] = 0; //assigning 0
//find product
for(int k=0;k<row;k++)
matrixResult[i][j]+ = matrixA[i][k] * matrixB[k][j];
}
}
display(matrixResult);
}
//function to display 6*6 matrix
void display(int matrix[][COL],
{
for(int i=0;i<ROW;i++)
{
for(int j=0;j<COL;j++)
printf("%d\t",matrix[i][j]);
printf("\n"); //new line
}
}

Step by stepSolved in 3 steps with 1 images

- Every data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardI am trying to write a rotate function in C language.arrow_forwardFunction Implementation with Data Abstraction in C Programming 1. Create a new file and SAVE as MATHV1.H Define and Implement the following functions: int sum(int x, int y); - returns the computed sum of two numbers x and y int diff(int x, int y); - returns the computed difference of two numbers x and y. Make sure that the larger number is deducted by the smaller number to avoid a negative answer long int prod(int x, int y); - returns the computed product of two number x and y float quot(int x, int y); - returns the computed quotient of two numbers x and y. Make sure that the larger number is always the numerator and divided by the smaller number to avoid division by zero error. int mod(int x, int y); - returns the computed remainder of two numbers x and y using the modulo operator %2. Create another new file and save as MYTOOLS.H void center(int row, char str[]); /*centers text across the screen -calculate here for the center col using the formula col=(80-strlen(str))/2; -then use…arrow_forward
- need help in C++ Problem: You are asked to create a program for storing the catalog of movies at a DVD store using functions, files, and user-defined structures. The program should let the user read the movie through the file, add, remove, and output movies to the file. For this assignment, you must store the information about the movies in the catalog using a single vector. The vector's data type is a user-defined structure that you must define on functions.h following these rules: Identifier for the user-define structure: movie. Member variables of the structure "movie": name (string), year (int), and genre (string). Note: you must use the identifiers presented before when defining the user-defined structure. Your solution will NOT pass the unit test cases if you do not follow the instructions presented above. The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). The following user-defined functions are…arrow_forwardStructural Verification Structural verification is, in this case, validating that a data structure is formed according to its specification. For this lab you are given an essentially arbitrary specification, but you could think of this being used to verify a data structure produced by a program that must have certain properties in order to be used correctly. For example, a list must not be circular, or an image file might require a particular header describing its contents. You must implement this function, which examines a matrix and ensures that it adheres to the following specification: bool verify_matrix(int x, int y, int **matrix); This function accepts an X dimension, a Y dimension, and a matrix of y rows and x columns; although it is declared as int **, this is the same type of matrix as returned by parse_life() in PA1, and you should access it as a two-dimensional array. Note that it is stored in Y-major orientation; that is, matrix ranges from matrix[0][0] to matrix[y 1][x -…arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
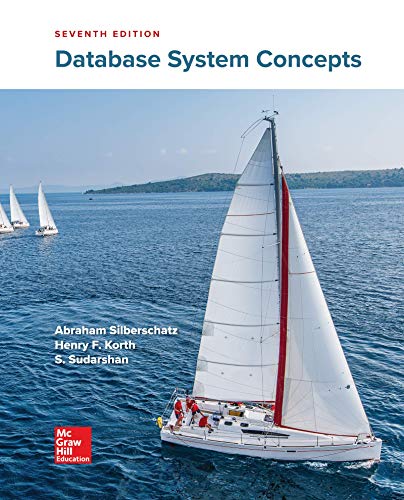
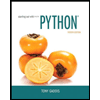
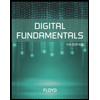
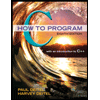
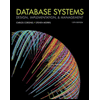
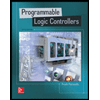