TODO 11 Using the axis_array defined below complete the following TODOs. Hint: Think about which axis is being collapsed or the direction of the arrow for each axis in the above picture. Compute the average using np.average for each row. Store the output into the variable row_avg. Find the minimum value using np.min for each column. Store the output into the variable col_min. axis_array = np.vstack([np.full((1, 5), 2), np.full((1, 5), 5)]) print(f"axis_array output: \n {axis_array}") print(f"axis_array shape: {axis_array.shape}") # TODO 11.1 row_avg = print(f"row_avg output: \n {row_avg}") print(f"row_avg shape: {row_avg.shape}") todo_check([ (row_avg.shape == (2,), 'row_avg does not have the correct shape of (2,)'), (np.all(row_avg == np.array([2, 5])), 'row_avg does not have the correct values') ]) # TODO 11.2 col_min = print(f"col_min output: \n {col_min}") print(f"col_min shape: {col_min.shape}") todo_check([ (col_min.shape == (5,), "col_min does not have the correct shape of (5,)"), (np.all(col_min == np.array([2, 2, 2, 2, 2])), 'col_min does not have the correct values') ])
TODO 11
Using the axis_array defined below complete the following TODOs.
Hint: Think about which axis is being collapsed or the direction of the arrow for each axis in the above picture.
- Compute the average using np.average for each row. Store the output into the variable row_avg.
- Find the minimum value using np.min for each column. Store the output into the variable col_min.
axis_array = np.vstack([np.full((1, 5), 2), np.full((1, 5), 5)])
print(f"axis_array output: \n {axis_array}")
print(f"axis_array shape: {axis_array.shape}")
# TODO 11.1
row_avg =
print(f"row_avg output: \n {row_avg}")
print(f"row_avg shape: {row_avg.shape}")
todo_check([
(row_avg.shape == (2,), 'row_avg does not have the correct shape of (2,)'),
(np.all(row_avg == np.array([2, 5])), 'row_avg does not have the correct values')
])
# TODO 11.2
col_min =
print(f"col_min output: \n {col_min}")
print(f"col_min shape: {col_min.shape}")
todo_check([
(col_min.shape == (5,), "col_min does not have the correct shape of (5,)"),
(np.all(col_min == np.array([2, 2, 2, 2, 2])), 'col_min does not have the correct values')
])

Introduction
In Python, the term "for each row" and "for each column" refers to the concept of looping over the elements in a 2-dimensional data structure, such as a matrix or a 2-dimensional array.
-
"For each row" means to iterate over the rows of a 2-dimensional data structure, where each iteration of the loop represents a row, and access is given to the elements in that row.
-
"For each column" means to iterate over the columns of a 2-dimensional data structure, where each iteration of the loop represents a column, and access is given to the elements in that column.
Here is an example of how you might use these terms in a for loop:
import numpy as np
# Create a 2-dimensional array
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Loop over each row
for row in matrix:
print(row)
# Loop over each column
for col in matrix.T:
print(col)
OUTPUT:
[1 2 3]
[4 5 6]
[7 8 9]
[1 4 7]
[2 5 8]
[3 6 9]
Step by step
Solved in 2 steps

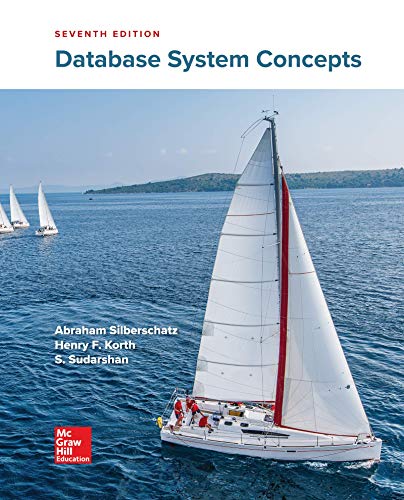
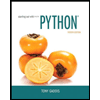
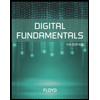
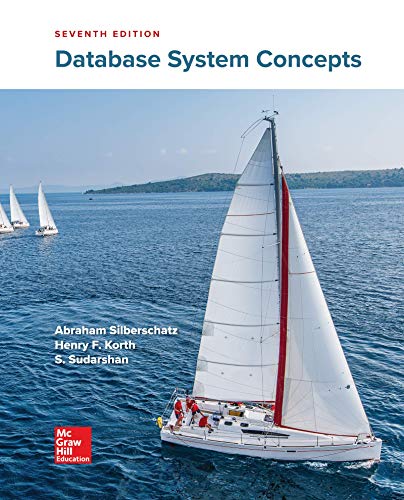
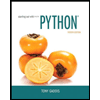
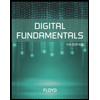
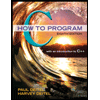
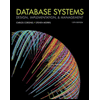
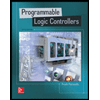