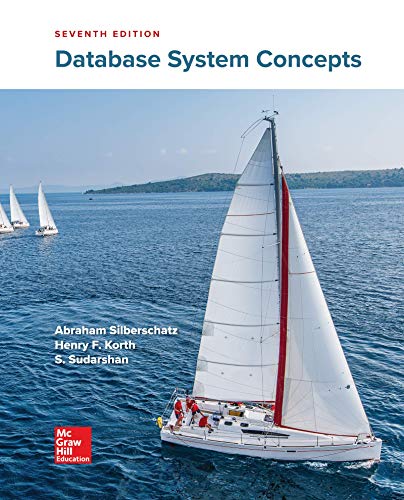
Write a python
Python ISBN Conversion Menu
1. Verify the check digit of an ISBN-10
2. Verify the check digit of an ISBN-13
3. Convert an ISBN-10 to an ISBN-13
4. Convert an ISBN-13 to an ISBN-10
5. Exit
Please remember to use what you have learned during the semester such as:
• Functions and/or Value Returning Functions
• If structures or logic structures IF/Elif/ELSE
• Data structures i.e. File input/output
• String manipulations i.e. string slicing and lists, etc.
• Modules
• Loops i.e. WHILE loops or FOR loops
I already created the program by using Python, but here is what I need help with (in bold):
• Reading and writing data to a file(s). In other words, have the program to read ISBNs from a file and store the correct results in a file as well.
Can you include codes within my current program (included below) so that my program will read ISBNs from a file and store the correct results in a file as well?
* Please make sure to use the Python program when making out the code in specific. Thanks!
My current Python program:
def main():
menu()
def menu():
print('1. Verify the check digit of an ISBN-10.')
print('2. Verify the check digit of an ISBN-13.')
print('3. Convert an ISBN-10 to an ISBN-13.')
print('4. Convert an ISBN-13 to an ISBN-10.')
print('5. Exit')
command = input('Enter the command (1-5): ')
if command == '5':
return
elif command == '1' or command == '3':
ISBN = input('Please enter the ISBN-10 number: ')
ISBN = ISBN.replace('-', '')
while len(ISBN) != 10:
print('Please make sure you have entered a number that is exactly 10 characters long.')
ISBN = input('Please enter the ISBN number: ')
ISBN = ISBN.replace('-', '')
print(format(ISBN))
if command == '1':
print(check_10_digit(ISBN))
else:
print(convert_10_to_13(ISBN))
elif command == '2' or command == '4':
ISBN = input('Please enter the 13 digit number: ')
ISBN = ISBN.replace('-','')
while len(format(ISBN)) != 13:
print('Please make sure you have entered a number that is exactly 13 characters long.')
ISBN = input('Please enter the 13 digit number: ')
ISBN = ISBN.replace('-', '')
if command == '2':
print(check_13_digit(ISBN))
else:
print(convert_13_to_10(ISBN))
menu()
def check_10_digit(ISBN):
digit = 0
for i in range(len(ISBN)):
n = ISBN[i]
num = int(n)
digit += (i+1) * num
d10 = digit % 11
print('Your ISBN-10 is: ', ISBN, 'with a remainder of ', str(d10))
if d10 == 0:
print('Your ISBN-10 is valid.')
else:
print('Your ISBN-10 is NOT valid.')
def check_13_digit(ISBN):
n = str(ISBN)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int (n[i]) * 3
digit = digit % 10
if digit == 0:
print('Your remainder is: ', digit, ', which means that your ISBN-13 is valid.')
else:
print('Your remainder is: ', digit, ', which means that your ISBN-13 is NOT valid.')
def convert_10_to_13(ISBN):
ISBN_13 = '978' + ISBN[:-1]
n = str(ISBN_13)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = digit % 10
ISBN_New = str(ISBN_13) + str(digit)
print('The ISBN-10 number ', ISBN, 'is converted to the ISBN-13 number ', ISBN_New)
def convert_13_to_10(ISBN):
if ISBN[:3] == '978':
n = str(ISBN[3:-1])
else :
raise 'ISBN is not convertible.'
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = 10 - (digit % 10)
ISBN_New = str(n) + str(digit)
print('The ISBN-13 number ', ISBN, 'is converted to the ISBN-10 number ', ISBN_New)
main()

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- C Language Title: Quests from the Queenarrow_forwardWrite in Java a procedure or function that will have four different effects, depending on whether arguments are passed by value, by reference, by value/result, or by name.arrow_forwardPlease help in guiding me in the necessary steps i should take when tackling this assignment, there is a main.cpp given Objective: The main objective of this assignment is to assess students’ ability to apply the stepwise refinement process to develop a new algorithm and carry that through to the implementation of the program. Implementation must follow the top-down design approach, where the solution starts by describing the general functionality of a game. Next, more details are provided in successive steps to refine the implementation. Problem Description: Assume you are hired by a game developing company to write a new computer game for kids. This company has decided to create a version of tricky triangles with a few different rules hoping that this new game will be more entertaining. If you are unfamiliar with the original game of tricky triangle, please learn how to play. This modified version of tricky triangles that you required to create is very much like the original game.…arrow_forward
- Using C# program explain step by step using your code how can we reverse the string. Dont forgot to put the comments with proper explainationarrow_forwardPYTHON Q9: Assignment statements, such as x = 3, define variables in programs. To execute one in an environment diagram, record the variable name and the value: Evaluate the expression on the right side of the = sign Write the variable name and the expression's value in the current frame. Use these rules to draw a simple diagram for the assignment statements below. x = 10 % 4 y = x x **= 2arrow_forwardA(n) on its own but can be used with other software products. _is a small application that cannot runarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
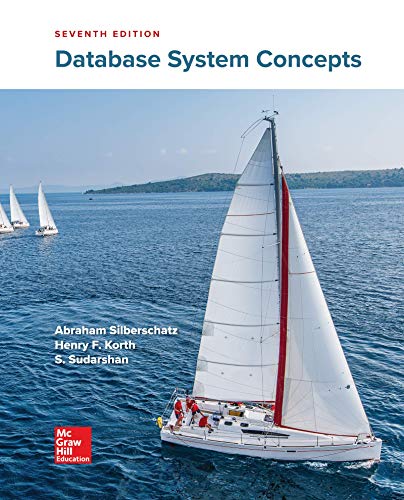
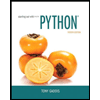
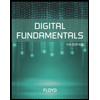
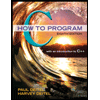
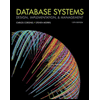
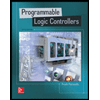