This will be a menu-driven program. The menu should look like this: Menu ==== 1. Addition of non-negative numbers 2. Alphabetize strings 3. Exit Enter your choice: If the user chooses #1 in the menu, your program should ask the user for 2 integers, then print them and their total. Keep doing this entire process until at least one of the numbers is negative. At that point, the program should print a message that at least one of the numbers is negative. Ex: if the user enters 13 and 2, the output would look like: You entered 13 and 2 Neither is negative Their total is 15 (and the loop should execute again) Ex: if the user enters 100 and -7, the output would look like: You entered 100 and -7 At least one is negative (notice no total is printed and the loop should NOT execute again) If the user chooses #2 in the menu, your program should ask the user for 2 lowercase strings (assume the user will enter lowercase strings) and tell which would come first alphabetically in the form shown in the examples. Keep doing this entire process until one of the strings is "stop". Ex: if the user enters "help" and “hello", the output would look like: 'hello' comes before 'help' alphabetically (and the loop should execute again) Ex: if the user enters "abcdefg" and "abcdefh", the output would look like: 'abcdefg' comes before 'abcdefh' alphabetically (and the loop should execute again) Ex: if the user enters "aaaaa" and "stop", the output would look like: Program is over (notice no comparison is printed and the loop should NOT execute again)
Hi, I need to solve this problem with C++


#include<iostream>
#include<string.h>
using namespace std;
int main()
{
int n1,n2,ch;
string s1,s2;
char choice;
while(true){
cout<<endl<<"Menu";
cout<<endl<<"=====";
cout<<endl<<"1. Addition of non-nrgative numbers";
cout<<endl<<"2. Alphabetize strings";
cout<<endl<<"3. Exit";
cout<<endl<<endl<<"Enter your choice:";
cin>>ch;
switch(ch)
{
case 1:
while(true){
cout<<endl<<"Enter two integer numbers: ";
cin>>n1>>n2;
cout<<endl<<"You entered "<<n1<<" and "<<n2;
if(n1>0 && n2>0)
{
cout<<endl<<"Neither is negative";
cout<<endl<<"Their total is: "<<n1+n2;
}
if(n1<0||n2<0)
{
cout<<endl<<"At least one is negative";
break;
}
}
break;
case 2:
while(true)
{
cout<<endl<<"Enter two lowercase strings: ";
cin>>s1>>s2;
if(s1=="stop"||s2=="stop")
{
cout<<endl<<"Program is over";
break;
}
if(s1>s2)
cout<<endl<<s2<<" comes before "<<s1<<" alphabetically";
else
cout<<endl<<s1<<" comes before "<<s2<<" alphabetically";
}
break;
case 3:
cout<<"Program is over";
exit(0);
default:
cout<<"Wrong choice!";
}
}
}
Step by step
Solved in 2 steps with 2 images

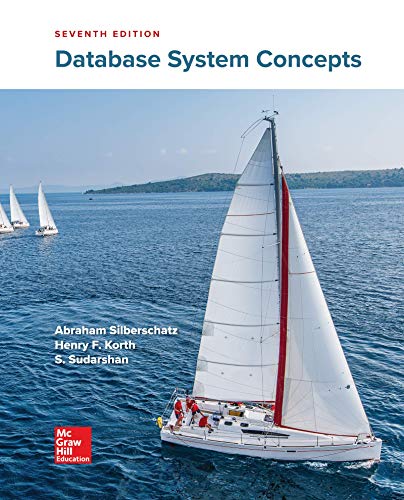
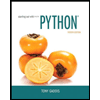
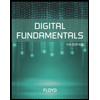
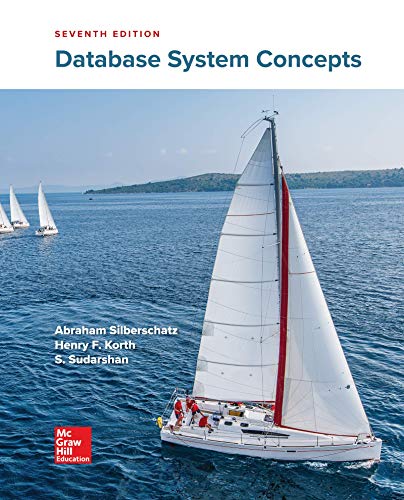
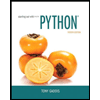
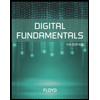
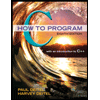
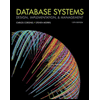
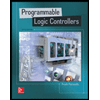