The recursion base case is when the submatrices degenerate into 1x1 matrices (single numbers) at which point you multiply 2 numbers and return them. Addition and Subtraction of two matrices takes O(N²) time. So the recurrence relation can be written as: T(N) 7T(N/2) + = O(N²) Explain in your solution documentation (in the Java file header) or if you prefer in a separate .txt (text) or .doc/.docx (Word) file: - - Which case of the Master's Theorem applies (CLRS case 1, 2 or 3). - What is the time complexity of the above method in terms of an upper bound O(.)? Do you expect this to run faster than the naive method, which has cubic complexity? This question asks you to implement Strassen's square matrix multiplication method in Java. This is a naive way to multiply two matrices, with a cubic time complexity of O(n³): void multiply(int A☐] [N], int B[] [N], int C[] [N]) { for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { C[i][j] = 0; for (int k = 0; k < N; k++) { C[i][j] += A[i][k]*B[k][j]; } } } } Strassen's matrix multiplication method makes 7 recursive calls. Strassen's method is a divide and conquer method in the sense that this method divides square matrices into sub-matrices of size N/2 x N/2 (similar to the way MergeSort divides arrays as we saw in class). In Strassen's method, the four sub-matrices of the result are calculated as follows. As you can see, there are 7 submatrices with 7 multiplications done (one for each Mk). M1 := (A1,1 A2,2)(B1,1 + B2,2) M2 = (A2,1 + A2,2)B1,1 A1,1 (B1,2 B2,2) - M3 := M₁ == A2,2 (B2,1 B1,1) - M5 M6 := := M7 := = (A1,1 + A1,2) B2,2 (A2,1 A1,1)(B1,1 + B1,2) - (A1,2 A2,2)(B2,1 + B2,2) Now express Ci,j in terms of Mk and join them in the overall matrix C: C1,1 = M1 C1,2 M4 - M5 + M7 M3 + M5 C2,1 M2 M4 = C2.2 M₁ M2 + M3 + M6 =
The recursion base case is when the submatrices degenerate into 1x1 matrices (single numbers) at which point you multiply 2 numbers and return them. Addition and Subtraction of two matrices takes O(N²) time. So the recurrence relation can be written as: T(N) 7T(N/2) + = O(N²) Explain in your solution documentation (in the Java file header) or if you prefer in a separate .txt (text) or .doc/.docx (Word) file: - - Which case of the Master's Theorem applies (CLRS case 1, 2 or 3). - What is the time complexity of the above method in terms of an upper bound O(.)? Do you expect this to run faster than the naive method, which has cubic complexity? This question asks you to implement Strassen's square matrix multiplication method in Java. This is a naive way to multiply two matrices, with a cubic time complexity of O(n³): void multiply(int A☐] [N], int B[] [N], int C[] [N]) { for (int i = 0; i < N; i++) { for (int j = 0; j < N; j++) { C[i][j] = 0; for (int k = 0; k < N; k++) { C[i][j] += A[i][k]*B[k][j]; } } } } Strassen's matrix multiplication method makes 7 recursive calls. Strassen's method is a divide and conquer method in the sense that this method divides square matrices into sub-matrices of size N/2 x N/2 (similar to the way MergeSort divides arrays as we saw in class). In Strassen's method, the four sub-matrices of the result are calculated as follows. As you can see, there are 7 submatrices with 7 multiplications done (one for each Mk). M1 := (A1,1 A2,2)(B1,1 + B2,2) M2 = (A2,1 + A2,2)B1,1 A1,1 (B1,2 B2,2) - M3 := M₁ == A2,2 (B2,1 B1,1) - M5 M6 := := M7 := = (A1,1 + A1,2) B2,2 (A2,1 A1,1)(B1,1 + B1,2) - (A1,2 A2,2)(B2,1 + B2,2) Now express Ci,j in terms of Mk and join them in the overall matrix C: C1,1 = M1 C1,2 M4 - M5 + M7 M3 + M5 C2,1 M2 M4 = C2.2 M₁ M2 + M3 + M6 =
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please answer this question using java

Transcribed Image Text:The recursion base case is when the submatrices degenerate into 1x1 matrices (single numbers) at
which point you multiply 2 numbers and return them.
Addition and Subtraction of two matrices takes O(N²) time. So the recurrence relation can be
written as:
T(N) 7T(N/2) +
=
O(N²)
Explain in your solution documentation (in the Java file header) or if you prefer in a separate .txt (text)
or .doc/.docx (Word) file:
-
- Which case of the Master's Theorem applies (CLRS case 1, 2 or 3).
- What is the time complexity of the above method in terms of an upper bound O(.)?
Do you expect this to run faster than the naive method, which has cubic complexity?
![This question asks you to implement Strassen's square matrix multiplication method in Java.
This is a naive way to multiply two matrices, with a cubic time complexity of O(n³):
void multiply(int A☐] [N], int B[] [N], int C[] [N])
{
for (int i
=
0; i < N; i++)
{
for (int j = 0; j < N; j++)
{
C[i][j] = 0;
for (int k
=
0; k < N; k++)
{
C[i][j] += A[i][k]*B[k][j];
}
}
}
}
Strassen's matrix multiplication method makes 7 recursive calls. Strassen's method is a divide and
conquer method in the sense that this method divides square matrices into sub-matrices of size N/2
x N/2 (similar to the way MergeSort divides arrays as we saw in class).
In Strassen's method, the four sub-matrices of the result are calculated as follows. As you can see,
there are 7 submatrices with 7 multiplications done (one for each Mk).
M1 :=
(A1,1
A2,2)(B1,1 + B2,2)
M2 = (A2,1 + A2,2)B1,1
A1,1 (B1,2 B2,2)
-
M3
:=
M₁ ==
A2,2 (B2,1 B1,1)
-
M5
M6
:=
:=
M7 :=
=
(A1,1 + A1,2) B2,2
(A2,1 A1,1)(B1,1 + B1,2)
-
(A1,2 A2,2)(B2,1 + B2,2)
Now express Ci,j in terms of Mk and join them in the overall matrix C:
C1,1 = M1
C1,2
M4 - M5 + M7
M3 + M5
C2,1 M2 M4
=
C2.2 M₁ M2 + M3 + M6
=](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6cdd3667-54b4-4e0a-a8bb-b3ac526afae3%2Fce8fa6d2-6140-4e98-8694-23c730fb8767%2Fyydglni_processed.png&w=3840&q=75)
Transcribed Image Text:This question asks you to implement Strassen's square matrix multiplication method in Java.
This is a naive way to multiply two matrices, with a cubic time complexity of O(n³):
void multiply(int A☐] [N], int B[] [N], int C[] [N])
{
for (int i
=
0; i < N; i++)
{
for (int j = 0; j < N; j++)
{
C[i][j] = 0;
for (int k
=
0; k < N; k++)
{
C[i][j] += A[i][k]*B[k][j];
}
}
}
}
Strassen's matrix multiplication method makes 7 recursive calls. Strassen's method is a divide and
conquer method in the sense that this method divides square matrices into sub-matrices of size N/2
x N/2 (similar to the way MergeSort divides arrays as we saw in class).
In Strassen's method, the four sub-matrices of the result are calculated as follows. As you can see,
there are 7 submatrices with 7 multiplications done (one for each Mk).
M1 :=
(A1,1
A2,2)(B1,1 + B2,2)
M2 = (A2,1 + A2,2)B1,1
A1,1 (B1,2 B2,2)
-
M3
:=
M₁ ==
A2,2 (B2,1 B1,1)
-
M5
M6
:=
:=
M7 :=
=
(A1,1 + A1,2) B2,2
(A2,1 A1,1)(B1,1 + B1,2)
-
(A1,2 A2,2)(B2,1 + B2,2)
Now express Ci,j in terms of Mk and join them in the overall matrix C:
C1,1 = M1
C1,2
M4 - M5 + M7
M3 + M5
C2,1 M2 M4
=
C2.2 M₁ M2 + M3 + M6
=
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
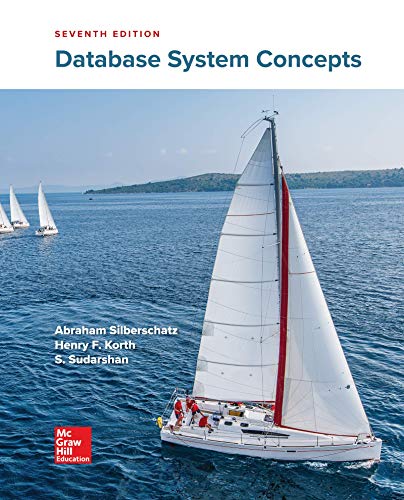
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
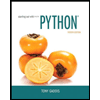
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
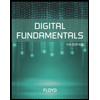
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
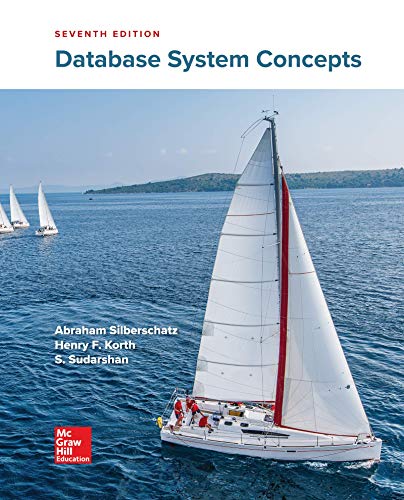
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
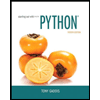
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
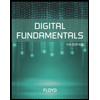
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
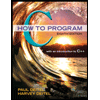
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
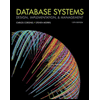
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
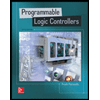
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education