This is for Coral. Sites like Zillow get input about house prices from a database and provide nice summaries for readers. Write a program with two inputs, current price and last month's price (both integers). Then, output a summary listing the price, the change since last month, and the estimated monthly mortgage computed as (currentPrice * 0.045) / 12. Output the estimated monthly mortgage (estMortgage) with two digits after the decimal point, which can be achieved as follows: Put estMortgage to output with 2 decimal places Ex: If the input is: 200000 210000 the output is: This house is $200000. The change is $-10000 since last month. The estimated monthly mortgage is $750.00. Note: Getting the precise spacing, punctuation, and newlines exactly right is a key point of this assignment. Such precision is an important part of programming. The code I have written is: integer currentPrice integer lastMonthsPrice integer changeSinceLastMonth integer estMortgage currentPrice = Get next input lastMonthsPrice = Get next input changeSinceLastMonth = currentPrice - lastMonthsPrice estMortgage = (currentPrice * 0.045) / 12 Put "This house is $" to output Put currentPrice to output Put ". The change is $" to output Put changeSinceLastMonth to output Put " since last month.\n" to output Put "The estimated monthly mortgage is $" to output Put estMortgage to output Put "." to output However the output does NOT have the two digits after decimal in the estMortgage variable. (IE $750.00) How do I fix this?
This is for Coral.
Sites like Zillow get input about house prices from a
Output the estimated monthly mortgage (estMortgage) with two digits after the decimal point, which can be achieved as follows:
Put estMortgage to output with 2 decimal places
Ex: If the input is:
200000 210000
the output is:
This house is $200000. The change is $-10000 since last month. The estimated monthly mortgage is $750.00.
Note: Getting the precise spacing, punctuation, and newlines exactly right is a key point of this assignment. Such precision is an important part of
The code I have written is:
integer currentPrice
integer lastMonthsPrice
integer changeSinceLastMonth
integer estMortgage
currentPrice = Get next input
lastMonthsPrice = Get next input
changeSinceLastMonth = currentPrice - lastMonthsPrice
estMortgage = (currentPrice * 0.045) / 12
Put "This house is $" to output
Put currentPrice to output
Put ". The change is $" to output
Put changeSinceLastMonth to output
Put " since last month.\n" to output
Put "The estimated monthly mortgage is $" to output
Put estMortgage to output
Put "." to output
However the output does NOT have the two digits after decimal in the estMortgage variable. (IE $750.00) How do I fix this?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

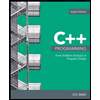
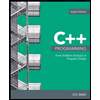