This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex. The bitwise operations are also available as __dunders__._. In question #6, I asked you to write a decimal to hex function: Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024. Most students submitted versions using this algorithm and the primary algorithm calculates list[index] using the // and % operators. def integerToHexa (integer): hexaDict = {'10': 'A', '11': 'B', '12': 'C', '13': 'D', '14': 'E', '15': 'D'} hexalist = [] while True: integer1 integer integer integer // 16 remainder = integer1 % 16 if integer <= 0: hexalist.append(remainder) break hexalist.append(remainder) hexalist.reverse() for i in hexalist: if i > 9: index = hexalist.index(i) hexaList[index] = str(hexaDict[f'{i}']) hexa = **.join(map(str, (hexalist))) print(f'0x{hexa}') The identifying feature of this algorithm is to use the 'integer' and 'integer1' variables as Indices into a list or map. This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a List, Dictionary or Tuple for your Hex_Map as shown above, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex.
This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex. The bitwise operations are also available as __dunders__._. In question #6, I asked you to write a decimal to hex function: Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024. Most students submitted versions using this algorithm and the primary algorithm calculates list[index] using the // and % operators. def integerToHexa (integer): hexaDict = {'10': 'A', '11': 'B', '12': 'C', '13': 'D', '14': 'E', '15': 'D'} hexalist = [] while True: integer1 integer integer integer // 16 remainder = integer1 % 16 if integer <= 0: hexalist.append(remainder) break hexalist.append(remainder) hexalist.reverse() for i in hexalist: if i > 9: index = hexalist.index(i) hexaList[index] = str(hexaDict[f'{i}']) hexa = **.join(map(str, (hexalist))) print(f'0x{hexa}') The identifying feature of this algorithm is to use the 'integer' and 'integer1' variables as Indices into a list or map. This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a List, Dictionary or Tuple for your Hex_Map as shown above, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use BITWISE operators
![This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a
List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to
hex. The bitwise operations are also available as __dunders___.
In question #6, I asked you to write a decimal to hex function:
Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write
your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other
Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024.
Most students submitted versions using this algorithm and the primary algorithm calculates list[index] using the // and % operators.
def integerToHexa (integer):
hexaDict = {'10': 'A', '11': 'B', '12': 'C', '13': 'D', '14': 'E', '15': 'D'}
hexaList = []
while True:
integer1 = integer
integer = integer // 16
remainder = integer1 % 16
if integer <= 0:
hexaList.append(remainder)
break
hexaList.append(remainder)
hexaList.reverse()
for i in hexaList:
if i > 9:
index = hexalist.index(i)
hexaList[index] = str(hexaDict[f'{i}'])
hexa = **.join(map(str, (hexaList)))
print(f'0x{hexa}')
The identifying feature of this algorithm is to use the 'integer' and 'integer1' variables as Indices into a list or map.
This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a
List, Dictionary or Tuple for your Hex_Map as shown above, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to
hex.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5483afab-7850-40f6-959f-e698dea1419a%2F434aef8f-eada-4db7-9a69-1095af874a18%2Fyimuym5_processed.png&w=3840&q=75)
Transcribed Image Text:This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a
List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to
hex. The bitwise operations are also available as __dunders___.
In question #6, I asked you to write a decimal to hex function:
Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write
your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other
Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024.
Most students submitted versions using this algorithm and the primary algorithm calculates list[index] using the // and % operators.
def integerToHexa (integer):
hexaDict = {'10': 'A', '11': 'B', '12': 'C', '13': 'D', '14': 'E', '15': 'D'}
hexaList = []
while True:
integer1 = integer
integer = integer // 16
remainder = integer1 % 16
if integer <= 0:
hexaList.append(remainder)
break
hexaList.append(remainder)
hexaList.reverse()
for i in hexaList:
if i > 9:
index = hexalist.index(i)
hexaList[index] = str(hexaDict[f'{i}'])
hexa = **.join(map(str, (hexaList)))
print(f'0x{hexa}')
The identifying feature of this algorithm is to use the 'integer' and 'integer1' variables as Indices into a list or map.
This exercise is to write a Decimal to Hex function using BYTE operations. See the Bitstreams.pdf on Canvas for a Bit/Byte Operation review. You can use a
List, Dictionary or Tuple for your Hex_Map as shown above, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to
hex.
Expert Solution

Step 1
Given an decimal which we have to convert in a hexadecimal type. a function is a block of code by which we can perform any specific task for which the function is made.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
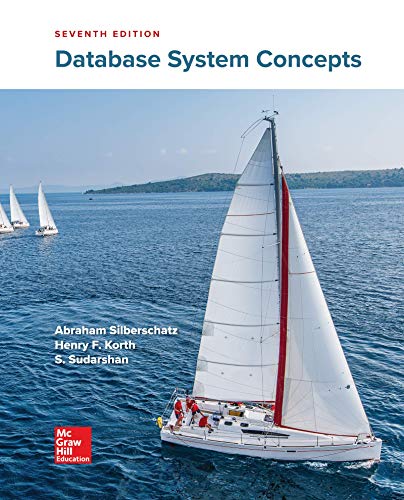
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
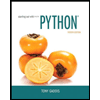
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
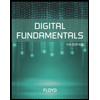
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
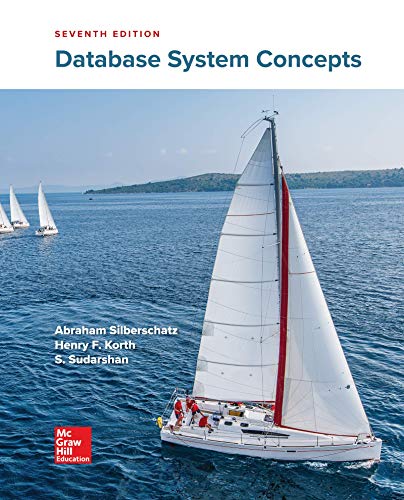
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
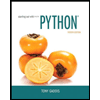
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
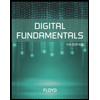
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
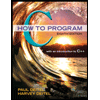
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
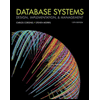
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
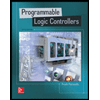
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education