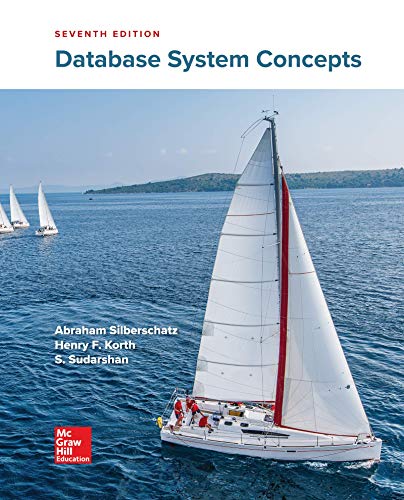
Using Lisp, implement a
For example, the call should be like:
Call: (mac '(3 3 l) '(0 0 r))
Output: ((3 3 L) (2 2 R) (3 2 L) (3 0 R) (3 1 L) (1 1 R) (2 2 L) (0 2 R) (0 3 L) (0 1 R) (1 1 L) (0 0 R))

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

(defmacro mac (start end)
`(dfs ,start ,end nil nil))
(defun dfs (current-state goal-state path &optional (solution-found nil))
(cond
((and solution-found (equal current-state goal-state))
(format t "Solution: ~A~%" (reverse path)))
((member current-state path)
nil)
(t
(let ((new-paths (generate-next-states current-state)))
(dolist (new-state new-paths)
(dfs new-state goal-state (cons current-state path) t))))))
(defun generate-next-states (state)
(let* ((m (car state))
(c (cadr state))
(b (caddr state))
(next-states '()))
(loop for m-move from 0 to m
do (loop for c-move from 0 to c
do (when (valid-move m-move c-move m c b)
(let* ((new-state (update-state state m-move c-move))
(missionaries-on-left (- m m-move))
(cannibals-on-left (- c c-move))
(boat-position (if (eq b 'l) 'r 'l)))
(push (list missionaries-on-left cannibals-on-left boat-position) next-states)))))
next-states))
(defun valid-move (m-move c-move m c b)
(and (<= 0 m-move m) (<= 0 c-move c)
(<= (+ m-move c-move) 2)
(or (and (eq b 'l) (>= (- m m-move) (- c c-move)))
(and (eq b 'r) (>= (- c c-move) (- m m-move))))))
(defun update-state (state m-move c-move)
(let* ((m (car state))
(c (cadr state))
(b (caddr state)))
(if (eq b 'l)
(list (- m m-move) (- c c-move) 'r)
(list (+ m m-move) (+ c c-move) 'l))))
;; Example usage:
(mac '(3 3 l) '(0 0 r))
This is the code provided that runs, but gives the incorrect output. Check the attached image to see what I get.
The solution should simply be:
((3 3 L) (2 2 R) (3 2 L) (3 0 R) (3 1 L) (1 1 R) (2 2 L) (0 2 R) (0 3 L) (0 1 R) (1 1 L) (0 0 R))
Please help me fix!
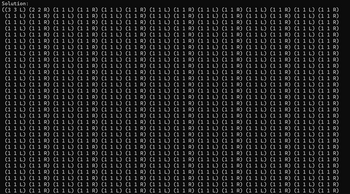
The code still does not seem to work for me. The error I get is: "
This code is still not working, can you fix it? I get the same error: "L is not a number".
I have tried to run the code, using the example usage as well and I get the error: "L is not a number".
I will note that I am using GNU Common Lisp to run the code. Do you know how I could fix that?
(defmacro mac (start end)
`(dfs ,start ,end nil nil))
(defun dfs (current-state goal-state path &optional (solution-found nil))
(cond
((and solution-found (equal current-state goal-state))
(format t "Solution: ~A~%" (reverse path)))
((member current-state path)
nil)
(t
(let ((new-paths (generate-next-states current-state)))
(dolist (new-state new-paths)
(dfs new-state goal-state (cons current-state path) t))))))
(defun generate-next-states (state)
(let* ((m (car state))
(c (cadr state))
(b (caddr state))
(next-states '()))
(loop for m-move from 0 to m
do (loop for c-move from 0 to c
do (when (valid-move m-move c-move m c b)
(let* ((new-state (update-state state m-move c-move))
(missionaries-on-left (- m m-move))
(cannibals-on-left (- c c-move))
(boat-position (if (eq b 'l) 'r 'l)))
(push (list missionaries-on-left cannibals-on-left boat-position) next-states)))))
next-states))
(defun valid-move (m-move c-move m c b)
(and (<= 0 m-move m) (<= 0 c-move c)
(<= (+ m-move c-move) 2)
(or (and (eq b 'l) (>= (- m m-move) (- c c-move)))
(and (eq b 'r) (>= (- c c-move) (- m m-move))))))
(defun update-state (state m-move c-move)
(let* ((m (car state))
(c (cadr state))
(b (caddr state)))
(if (eq b 'l)
(list (- m m-move) (- c c-move) 'r)
(list (+ m m-move) (+ c c-move) 'l))))
;; Example usage:
(mac '(3 3 l) '(0 0 r))
This is the code provided that runs, but gives the incorrect output. Check the attached image to see what I get.
The solution should simply be:
((3 3 L) (2 2 R) (3 2 L) (3 0 R) (3 1 L) (1 1 R) (2 2 L) (0 2 R) (0 3 L) (0 1 R) (1 1 L) (0 0 R))
Please help me fix!
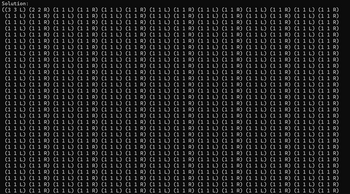
The code still does not seem to work for me. The error I get is: "
This code is still not working, can you fix it? I get the same error: "L is not a number".
I have tried to run the code, using the example usage as well and I get the error: "L is not a number".
I will note that I am using GNU Common Lisp to run the code. Do you know how I could fix that?
- In Kotlin, Write a recursive function called myZip, with an expression body. MyZip takes two Lists and returns a List of Pairs in which each value may be any type (I suggest your start by thinking out how to represent this data type). The pairs consist of corresponding elements in the two lists (the first element of the first list and the first element of the second list, etc). The base case should be that either (or both) of the original lists has length 1, so that, if the lists have different length, the zipping stops when the first list runs out of values.arrow_forwardWrite the code in python to define the function has_adjacent_repeats(mystr), which takes a string parameter and returns a boolean result. - If mystr has at least one instance of adjacent characters being equal, return True - Otherwise, return False Hint: You can iterate over the positive indices i for characters in mystr: 1, 2, 3, ...., len(mystr)-1 with a for loop. For each i, if the character at index i matches the character at index i - 1, return True. Otherwise, if no doubled character is found in the entire string, return False. For example: Test Result if not (has_adjacent_repeats("NOODLES") is True): print("error") if not (has_adjacent_repeats("Bananas") is False): print("shwoopsie") if not (has_adjacent_repeats("Hanoverr") is True): print("error")arrow_forwardWithout importing any Lib:arrow_forward
- In python, The function slice_end takes a string parameter, word, and a positive integer n; the function returns a slice of length n from the end of word. However, if word has length less than n, then the None object is returned instead. Hint: Notice that returning the empty string when n is zero might be a special case. You can use an if-elif-else decision structure and the slicing operator [ : ] to solve this problem. For example: Test Result word = "pineapple" n = 5 print(slice_end(word, n)) apple word = "too short" n = 20 print(slice_end(word, n)) None word = "llama" n = 0 print(slice_end(word, n)) word = "Saturn" n = 6 print(slice_end(word, n)) Saturnarrow_forwardWrite a function lis_rec(arr) that outputs the length of the longest increasing sequence and the actual longest increasing sequence. This function should use divide and conquer strategy to the solve the problem, by using an auxiliary function that is recursive. For example, one possibility is to define a recursive function, lis_rec(arr, i, prev), that takes the array arr, an index i, and the previous element index prev of LIS (which is part of the array arr before index i), and returns the length of the LIS that can be obtained by considering the subarray arr[i:]. Write a dynamic programming version ofthe function, lis_dp(arr), that outputs the length of the longest increasing sequence and the actual longest increasing sequence by using a table to store the results of subproblems in a bottom-up manner. Test the performance of the two functions on arrays of length n = 100, 500, 1000, 5000, 10000. Compare the running times and memory usage of the two functions.arrow_forwardImplement a function pairsThatSum that accepts two arguments: 1. a target number2. a list of numbers the function then returns a list of tuple s that contains all pairs of numbers from the list that sum to the given target number. Note: the pair can be two numbers with the same value, e.g. (2,2) but these must be different items (have different locations) in the list. See the last example below. each pair should be reported only once (don't include a pair and its reverse), and each pair's values should be listed in the order that they occur in the list. E.g., the first example records the pair (0,3) instead of the pair (3,0) , because 0 occurs in the list before 4 . the list of pairs should be ordered by the order of the first value in the list, e.g., (0,3) comes before (1,2) because 0 comes before 1 in the list. (note: if you take the right approach, you won't have to do anything extra for this requirement) Below is an example of the output from the code: >>>…arrow_forward
- Write a function using Java Function Name: winInRowParameters: board: 2D integer array, row: integer, piece: integerReturn: booleanAssume board is valid 2D int array, row is valid index in the board, piece is X==1/O==2Look at indicated row at given index in board. If that row has at least 3 consecutive entries withgiven type of piece (X/O) (3 in a row XXX, OOO), then return true, otherwise false.arrow_forwardIn c++, Thanks!!! Write the simplest program that will demonstrate iteration vs recursion using the following guidelines - Write two primary helper functions - one iterative (IsArrayPrimeIter) and one recursive (IsArrayPrimeRecur) - each of which Take an array and its size as input params and return a bool such that 'true' ==> array and all elements are prime, 'false' ==> at least one element in array is not prime, so array is not prime. Print out a message "Entering <function_name>" as the first statement of each function. Perform the code to test whether every element of the array is a Prime number. Print out a message "Leaving <function_name>" as the last statement before returning from the function. Remember - there will be nested loops for the iterative function and there can be no loops at all in the recursive function. You will need to define one other helper function (IsPrimeRecur) for the recursion which should also not contain any loops to make it a…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
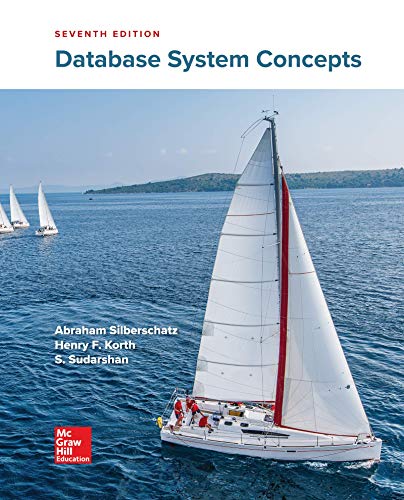
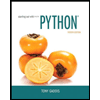
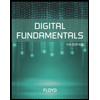
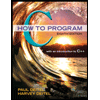
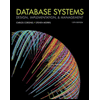
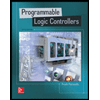