This code is in c. The question I have is that I have to find a min and maxes in arrays A and B. Based on source code and complied response below how can I fix my logical errors. Feel free to edit put notes about the source code. #include #include #include #define N 10 int main(void) { int i,j,k,n, max, min; float a[N], b[N],sum; char key_hit; do { printf("Please input how many values are inside array a (from 2 to 10):"); scanf("%i", &n); }while (n<2 || n>10); printf("\n\nPlease enter array a values"); for(i=1; i<=n; i+=1) { printf("\narray a value %i. : ",(i)); scanf("%f", &a[i]); } do { printf("Please input how many values are inside array b (from 2 to 10):"); scanf("%i", &k); }while (k<2 || k>10); printf("\n\nPlease enter array b values"); for(j=1; j<=k; j+=1) { printf("\narray b value %i. : ",(j)); scanf("%f", &b[j]); } printf("\n\nIs there anything choice you would like to do?"); printf("\nThe numbers related to the choices are listed below:"); printf("\n1. Max and min values of a."); printf("\n2. Max and min values of b."); printf("\n3. Dot product of a and b."); printf("\n4. Review a specific value in the set."); printf("\n5. End the program."); printf("\nPlease enter your choice (the number): "); scanf(" %c", &key_hit); key_hit == '0'; if(key_hit == '1') { min = a[0]; max = a[0]; for(i=0; i<=n; i++) { if(a[i] < min) { min = a[i+1]; } if(a[i] > max) { max = a[i]; } } printf("\nThe max value entry in a is: %i", max); printf("\nThe min value entry in a is: %i", min); } if(key_hit == '2') { min = b[0]; max = b[0]; for(j=0; j<=k; j++) { if(b[j] < min) { min = b[j]; } if(b[j] > max) { max = b[j]; } } printf("\nThe max value entry in b is: %i", max); printf("\nThe min value entry in b is: %i", min); } return 0; } Please input how many values are inside array a (from 2 to 10):5 Please enter array a values array a value 1. : 1 array a value 2. : 2 array a value 3. : 3 array a value 4. : 4 array a value 5. : 5 Please input how many values are inside array b (from 2 to 10):5 Please enter array b values array b value 1. : 1 array b value 2. : 2 array b value 3. : 3 array b value 4. : 4 array b value 5. : 5 Is there anything choice you would like to do? The numbers related to the choices are listed below: 1. Max and min values of a. 2. Max and min values of b. 3. Dot product of a and b. 4. Review a specific value in the set. 5. End the program. Please enter your choice (the number): 2 The max value entry in b is: 800052 The min value entry in b is: 1
This code is in c. The question I have is that I have to find a min and maxes in arrays A and B. Based on source code and complied response below how can I fix my logical errors. Feel free to edit put notes about the source code.
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#define N 10
int main(void)
{
int i,j,k,n, max, min;
float a[N], b[N],sum;
char key_hit;
do
{
printf("Please input how many values are inside array a (from 2 to 10):");
scanf("%i", &n);
}while (n<2 || n>10);
printf("\n\nPlease enter array a values");
for(i=1; i<=n; i+=1)
{
printf("\narray a value %i. : ",(i));
scanf("%f", &a[i]);
}
do
{
printf("Please input how many values are inside array b (from 2 to 10):");
scanf("%i", &k);
}while (k<2 || k>10);
printf("\n\nPlease enter array b values");
for(j=1; j<=k; j+=1)
{
printf("\narray b value %i. : ",(j));
scanf("%f", &b[j]);
}
printf("\n\nIs there anything choice you would like to do?");
printf("\nThe numbers related to the choices are listed below:");
printf("\n1. Max and min values of a.");
printf("\n2. Max and min values of b.");
printf("\n3. Dot product of a and b.");
printf("\n4. Review a specific value in the set.");
printf("\n5. End the program.");
printf("\nPlease enter your choice (the number): ");
scanf(" %c", &key_hit);
key_hit == '0';
if(key_hit == '1')
{
min = a[0];
max = a[0];
for(i=0; i<=n; i++)
{
if(a[i] < min)
{
min = a[i+1];
}
if(a[i] > max)
{
max = a[i];
}
}
printf("\nThe max value entry in a is: %i", max);
printf("\nThe min value entry in a is: %i", min);
}
if(key_hit == '2')
{
min = b[0];
max = b[0];
for(j=0; j<=k; j++)
{
if(b[j] < min)
{
min = b[j];
}
if(b[j] > max)
{
max = b[j];
}
}
printf("\nThe max value entry in b is: %i", max);
printf("\nThe min value entry in b is: %i", min);
}
return 0;
}
Please input how many values are inside array a (from 2 to 10):5
Please enter array a values
array a value 1. : 1
array a value 2. : 2
array a value 3. : 3
array a value 4. : 4
array a value 5. : 5
Please input how many values are inside array b (from 2 to 10):5
Please enter array b values
array b value 1. : 1
array b value 2. : 2
array b value 3. : 3
array b value 4. : 4
array b value 5. : 5
Is there anything choice you would like to do?
The numbers related to the choices are listed below:
1. Max and min values of a.
2. Max and min values of b.
3. Dot product of a and b.
4. Review a specific value in the set.
5. End the program.
Please enter your choice (the number): 2
The max value entry in b is: 800052
The min value entry in b is: 1

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

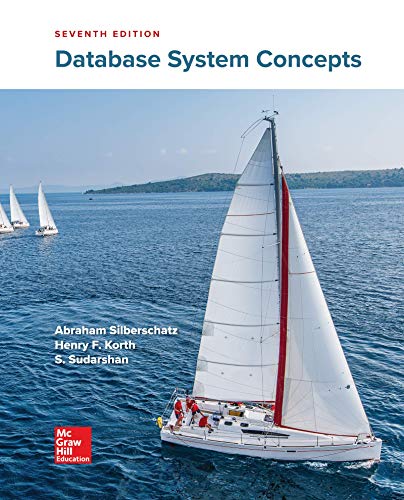
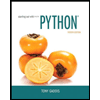
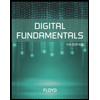
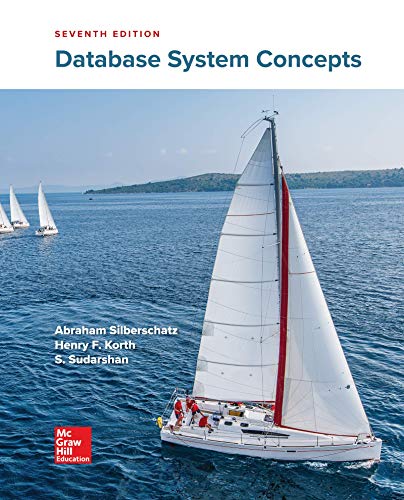
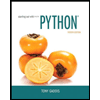
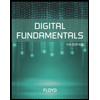
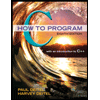
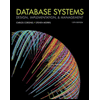
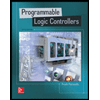