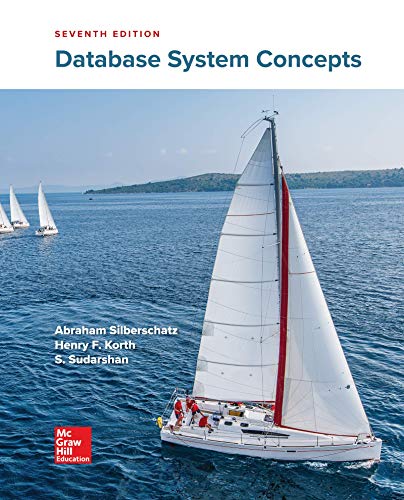
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
This code is correct in reversing a 32-bit integer when looking at the binary form of the integer. But it uses 54 operations instead of 45. We are strictly limited to using only !, +, ^, &, <<, >>, |, ~. We cannot use constants that are anything other than 0x0 to 0xFF. We also cannot use loops or conditionals, in other words "straight line" code. I am unsure where I can simplify this code in order to get it into the required maximum number of operations (45).
Will leave good review!
int bitReverse(int x) {
int result, mask1, mask2, mask4, mask8, mask16, min;
min = 1 << 31;
mask16 = ((0xff << 8) | 0xff);
mask8 = (mask16 << 8) ^ mask16;
mask4 = (mask8 << 4) ^ mask8;
mask2 = (mask4 << 2) ^ mask4;
mask1 = (mask2 << 1) ^ mask2;
mask1 = ~mask1;
mask2 = ~mask2;
mask4 = ~mask4;
mask8 = ~mask8;
mask16 = ~mask16;
result = ((x << 16) & mask16) | (((x & mask16) >> 16) & (~mask16));
result = ((result << 8) & mask8) | (((result & mask8) >> 8) & (~(min >> 7)));
result = ((result << 4) & mask4) | (((result & mask4) >> 4) & (~(min >> 3)));
result = ((result << 2) & mask2) | (((result & mask2) >> 2) & (~(min >> 1)));
result = ((result << 1) & mask1) | (((result & mask1) >> 1) & (~min));
return result;
}
int result, mask1, mask2, mask4, mask8, mask16, min;
min = 1 << 31;
mask16 = ((0xff << 8) | 0xff);
mask8 = (mask16 << 8) ^ mask16;
mask4 = (mask8 << 4) ^ mask8;
mask2 = (mask4 << 2) ^ mask4;
mask1 = (mask2 << 1) ^ mask2;
mask1 = ~mask1;
mask2 = ~mask2;
mask4 = ~mask4;
mask8 = ~mask8;
mask16 = ~mask16;
result = ((result << 8) & mask8) | (((result & mask8) >> 8) & (~(min >> 7)));
result = ((result << 4) & mask4) | (((result & mask4) >> 4) & (~(min >> 3)));
result = ((result << 2) & mask2) | (((result & mask2) >> 2) & (~(min >> 1)));
result = ((result << 1) & mask1) | (((result & mask1) >> 1) & (~min));
return result;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- As the algorithm performs division of numbers with high precision, it is very common to see really big numbers after the period (for example: 1200.2300001), which is not desirable because of several reasons: may cause confusion to some users when they see such big numbers; uses more memory to store a bigger number; it just does not make sense to display currency number in this format. For this reason, you are going to implement a utility function to format any number into the appropriate currency format, using 2 decimal places. For example: The number 1200.2300001 would be became: 1200.23arrow_forwardIn this lab, you will write a MIPS program to convert Fahrenheit temperature to Celsius. A basic conversion program was taught in the class. You should utilize that code from the lecture slides. Your program will take the Fahrenheit temperature as user input (single precision floating point). The temperature conversion code will be a leaf procedure that will be called from the main program after the user input. The procedure also will print the single precision floating value of the Celsius temperature. After the program returns to the main program, the user will be asked if they want another conversion. A character will be taken as input to indicate the user’s preference. If the input character is ‘y’, the program will continue from the beginning by taking another input and calling the conversion procedure. Any other input character will make the program exit. You may need to learn a few syscall parameters that deal with character and single precision floating point numbers. You will…arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments through the code, and test the given examples below. Thanks. Write a function crypto() that takes as an input a string s and returns an encryptedstring where encryption proceeds as follows: split the text up into blocks of two letterseach and swap each pair of letters (where spaces/punctuation, etc. is treated like letters).If the input string>>> crypto('Secret Message')'eSrcteM seaseg'>>> crypto('Secret Messages')'eSrcteM seasegs'arrow_forward
- Generate a list of 10 random numbers with values between 1 and 20, and submit the first 10 numbers as an answer to this question. (Use any random number generator). For the purpose of using them in the next questions, call these numbers A, B, C, D, . . . J Convert Aπ/B into degrees. Find the result ofa. Degrees = (A × B × C) + 180 mod 180; this is the remainder after dividing (A × B × C) + 180 by 180 b. Convert Degrees into radians, and put the answer in π notation. Calculate the measurements of the acute angles of a right triangle whose sides measure A, B, C units. Build a matrix, M, of random numbers as follows: A B C D E F G H I What is MT? Find the inverse of M, showing the steps. What is the result of M * M−1? Let V = [A B C] and W = [ D E F]. In the textbook, the dot product V • W involves an angle, alpha (α). How do you calculate alpha for this example? Let V = [A B C]. Find the cross product V • V. What can you conclude from this…arrow_forwardThe twins Kartik and Kunaal want to play word search puzzles with their class. They want to befaster than everyone else in their class, so they decided to write a C code to help them find wordswith a click of a button. Little did they know, everyone in class decided to do this question. You are required to implement search2D function. void search2D(char word[], int wordSize, const int Size, char grid[Size][Size]); search2D receives the word to look for char word[], the size of the word int wordSize, sizeof the grid const int Size and the puzzle grid char grid[Size][Size]. The word size can bebetween 2 and 23. You are NOT required to check for the validity of the word size.search2D should look for the first letter in the word row by row. If it finds the first letter of the wordin the puzzle, it should look for the remaining of the word in the 8 directions from the south and goesclockwise, i.e. south, south-west, west, north-west, north, north-east, east and lastly…arrow_forwardUse the cubicSpline module to write programs that interpolate between a given data point with a cubic spline. The program must be able to evaluate the interpolant for more than one x value. As a test, use the data points specified in the previous Example and calculate the interpolants at x = 1.5 and x = 4.5 (due to symmetry, these values must be the same) import numpy as np from cubicSpline import * xData = np.array([1,2,3,4,5], float) yData = np.array([0,1,0,1,0], float) k = curvatures (xData, yData) while True: try: x = eval(input("\nx ==> ")) except SyntaxError: break print("y=", evalSpline (xData, yData, k, x)) input ("Done. Press return to exit") Exercise Fix the Cubic Spline Program There is an error in the Cubic Spline program, fix it and evaluate the values of x 1/2 = 1.5 and x = 4.5, where the results of both values must be the same.arrow_forward
- So long as ptr is a reference to an integer, what happens if we add 4 to it?arrow_forwardCode C Can this be done as a flowchart please? Detailed and clear This week’s exercise is to implement an encoder. When completed, your program should be able to accept a short sentence from the user and display an encrypted version of it. Encryption is a complex subject but we can implement a very simple example as follows. For simplicity, let us assume one case of letters throughout, say CAPITALS. We can use a substitution method to scramble a sentence and then use the reverse of this process to recover it. If we take two rows of the alphabet and shift the bottom row along a specified amount (our key), we can then read the letters in our sentence from the top row and use the substituted letter from the bottom row to form the encrypted sentence: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z X Y Z A B C D E F G H I J K L M N O P Q R S T U V W In the example above, the key is 3, hence the second row is shifted by three characters along. Take, for instance, the sentence “HELLO”.…arrow_forwardCan this python code be done without using the isdigit() function. Please try to change the following code in the picture so it doesn't use isdigit().arrow_forward
- Can you give me two ways to do itarrow_forwardi need it ASAP pleasearrow_forwardThis code has a delay in the loop, during which time the user inputs will be ignored. So, please modify it and implement a solution that would trigger the needed function when there is an input. // Pin numbers written on the board itself do not correspond to Aurdino pinnumbers.Constants are defined to make using this board easierconst uint8_t BTN_PIN = 2;const uint8_t LED_PIN = 13;//assigning button;s previous state and LedSate to LOWuint8_t buttonPrevState = LOW;uint8_t ledState = LOW;//defining a new functionvoid setup(){// Assigning the types of input parameterspinMode(BTN_PIN, INPUT_PULLUP);//Assigning the types of output parameterspinMode(LED_PIN, OUTPUT);//begin of process detailsSerial.begin(9600);}void loop(){//assigning button state to be read modeuint8_t buttonState = digitalRead(BTN_PIN); //To print the following in output windowSerial.print(buttonState);Serial.print(buttonPrevState);Serial.print(ledState);Serial.println(""); //check if the button state presently is same…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
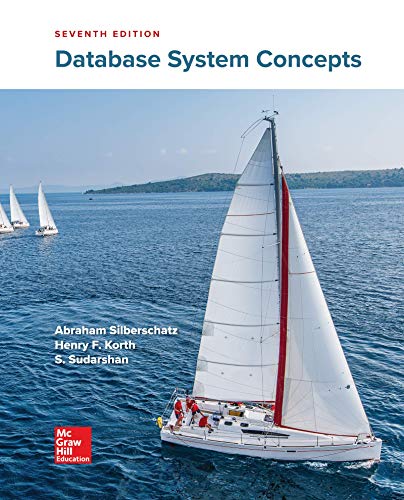
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
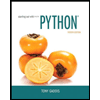
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
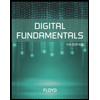
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
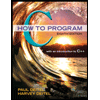
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
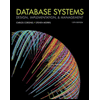
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
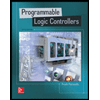
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education