There are two program below, please describe all the bugs you found and how you fixed them. Step 1: Follow the Demo To use GDB, you need to compile your program with a debugging (-g) flag gcc --std=c99 -g [some_program.c] -o [exe_name] Then, run gdb with the executable: gdb ./[exe_name] Step 2: Use GDB to resolve the segmentation fault Try to use GDB to debug the program buggy-list-sort.c. The program in buggy- list-sort.c specifically generates a short linked list containing random integers and tries to sort it using bubble sort. However, something in the code is broken, and the program crashes with a segmentation fault before the sorting is complete. If you were able to successfully debug the program, make sure to describe all of the bugs you found and how you fixed them in list_sort.txt. (3 pts) Step 3: Use GDB to resolve the logic error Try to use GDB to debug the program buggy-array-sort.c. This program generates a small array of random integers and tries to sort it using insertion sort. However, something in the program is broken, and the array is not correctly sorted. If you were able to successfully debug the program, make sure to describe all of the bugs you found and how you fixed them in array_sort.txt. Here is the buggy-list-sort.c /* * This program should sort a linked by increasing value using bubble sort, * but it's currently broken. Use GDB and Valgrind to help debug it. */ #include #include /* * This link structure is used below to implement a simple singly-linked list. */ struct link { int val; struct link* next; }; /* * This function swaps the values of two integers, using their memory addresses * (i.e. using pointers to those integers). */ void swap(int* a, int* b) { int tmp = *a; *a = *b; *b = tmp; } /* * This function implements bubble sort: * * https://en.wikipedia.org/wiki/Bubble_sort * * Here, we specifically sort a linked list of integers by increasing value * (i.e. the smallest value should be at the front of the array after it's * sorted). */ void bubble_sort(struct link* head) { int swapped = 0; do { swapped = 0; struct link* curr = head; while (curr != NULL) { if (curr->val > curr->next->val) { swap(&curr->val, &curr->next->val); swapped = 1; } curr = curr->next; } } while (swapped); } int main(int argc, char const *argv[]) { /* * Generate a linkd list containing 16 random values between 0 and 100. */ struct link* head = NULL; for (int i = 0; i < 16; i++) { struct link* newlink = malloc(sizeof(struct link)); newlink->val = rand() % 100; newlink->next = head; head = newlink; } /* * Print the entire list. */ printf("The unsorted list values are:\n"); for (struct link* curr = head; curr != NULL; curr = curr->next) { printf(" %d", curr->val); } printf("\n\n"); /* * Sort the list, then print it again. */ printf("The sorted list values are:\n"); bubble_sort(head); for (struct link* curr = head; curr != NULL; curr = curr->next) { printf(" %d", curr->val); } printf("\n"); return 0; } Here is the program buggy-array-sort.c * * This program should sort an array by decreasing value using insertion sort, * but it's currently broken. Use GDB and Valgrind to help debug it. */ #include #include /* * This function swaps the values of two integers, using their memory addresses * (i.e. using pointers to those integers). */ void swap(int* a, int* b) { int tmp = *a; *a = *b; *b = tmp; } /* * This function implements insertion sort: * * https://en.wikipedia.org/wiki/Insertion_sort * * Here, we specifically sort an array of integers by decreasing value (i.e. * the largest value should be at the front of the array after it's sorted). */ void insertion_sort(int* array, int n) { for (int i = 1; i < n; i++) { for (int j = i; j >= 0; j--) { if (array[j-1] >= array[j]) { break; } swap(&array[j-1], &array[j]); } } } int main(int argc, char const *argv[]) { /* * Generate an array containing 16 random values between 0 and 100. */ int n = 16; int* array = malloc(n * sizeof(int)); for (int i = 0; i < n; i++) { array[i] = rand() % 100; } /* * Print the unsorted array. */ printf("The unsorted array values are:\n"); for (int i = 0; i < n; i++) { printf(" %d", array[i]); } printf("\n\n"); /* * Sort the array and print it again. */ insertion_sort(array, n); printf("The sorted array values are:\n"); for (int i = 0; i < n; i++) { printf(" %d", array[i]); } printf("\n"); return 0; }
There are two program below, please describe all the bugs you found and how you fixed them.
Step 1: Follow the Demo
To use GDB, you need to compile your program with a debugging (-g) flag gcc --std=c99 -g [some_program.c] -o [exe_name]
Then, run gdb with the executable:
gdb ./[exe_name]
Step 2: Use GDB to resolve the segmentation fault
Try to use GDB to debug the program buggy-list-sort.c. The program in buggy- list-sort.c specifically generates a short linked list containing random integers and
tries to sort it using bubble sort. However, something in the code is broken, and the program crashes with a segmentation fault before the sorting is complete. If you were able to successfully debug the program, make sure to describe all of the bugs you found and how you fixed them in list_sort.txt.
(3 pts) Step 3: Use GDB to resolve the logic error
Try to use GDB to debug the program buggy-array-sort.c. This program generates a small array of random integers and tries to sort it using insertion
sort. However, something in the program is broken, and the array is not correctly sorted. If you were able to successfully debug the program, make sure to describe all of the bugs you found and how you fixed them in array_sort.txt.
Here is the buggy-list-sort.c
/*
* This program should sort a linked by increasing value using bubble sort,
* but it's currently broken. Use GDB and Valgrind to help debug it.
*/
#include <stdio.h>
#include <stdlib.h>
/*
* This link structure is used below to implement a simple singly-linked list.
*/
struct link {
int val;
struct link* next;
};
/*
* This function swaps the values of two integers, using their memory addresses
* (i.e. using pointers to those integers).
*/
void swap(int* a, int* b) {
int tmp = *a;
*a = *b;
*b = tmp;
}
/*
* This function implements bubble sort:
*
* https://en.wikipedia.org/wiki/Bubble_sort
*
* Here, we specifically sort a linked list of integers by increasing value
* (i.e. the smallest value should be at the front of the array after it's
* sorted).
*/
void bubble_sort(struct link* head) {
int swapped = 0;
do {
swapped = 0;
struct link* curr = head;
while (curr != NULL) {
if (curr->val > curr->next->val) {
swap(&curr->val, &curr->next->val);
swapped = 1;
}
curr = curr->next;
}
} while (swapped);
}
int main(int argc, char const *argv[]) {
/*
* Generate a linkd list containing 16 random values between 0 and 100.
*/
struct link* head = NULL;
for (int i = 0; i < 16; i++) {
struct link* newlink = malloc(sizeof(struct link));
newlink->val = rand() % 100;
newlink->next = head;
head = newlink;
}
/*
* Print the entire list.
*/
printf("The unsorted list values are:\n");
for (struct link* curr = head; curr != NULL; curr = curr->next) {
printf(" %d", curr->val);
}
printf("\n\n");
/*
* Sort the list, then print it again.
*/
printf("The sorted list values are:\n");
bubble_sort(head);
for (struct link* curr = head; curr != NULL; curr = curr->next) {
printf(" %d", curr->val);
}
printf("\n");
return 0;
}
Here is the program buggy-array-sort.c
*
* This program should sort an array by decreasing value using insertion sort,
* but it's currently broken. Use GDB and Valgrind to help debug it.
*/
#include <stdio.h>
#include <stdlib.h>
/*
* This function swaps the values of two integers, using their memory addresses
* (i.e. using pointers to those integers).
*/
void swap(int* a, int* b) {
int tmp = *a;
*a = *b;
*b = tmp;
}
/*
* This function implements insertion sort:
*
* https://en.wikipedia.org/wiki/Insertion_sort
*
* Here, we specifically sort an array of integers by decreasing value (i.e.
* the largest value should be at the front of the array after it's sorted).
*/
void insertion_sort(int* array, int n) {
for (int i = 1; i < n; i++) {
for (int j = i; j >= 0; j--) {
if (array[j-1] >= array[j]) {
break;
}
swap(&array[j-1], &array[j]);
}
}
}
int main(int argc, char const *argv[]) {
/*
* Generate an array containing 16 random values between 0 and 100.
*/
int n = 16;
int* array = malloc(n * sizeof(int));
for (int i = 0; i < n; i++) {
array[i] = rand() % 100;
}
/*
* Print the unsorted array.
*/
printf("The unsorted array values are:\n");
for (int i = 0; i < n; i++) {
printf(" %d", array[i]);
}
printf("\n\n");
/*
* Sort the array and print it again.
*/
insertion_sort(array, n);
printf("The sorted array values are:\n");
for (int i = 0; i < n; i++) {
printf(" %d", array[i]);
}
printf("\n");
return 0;
}

Step by step
Solved in 4 steps with 4 images

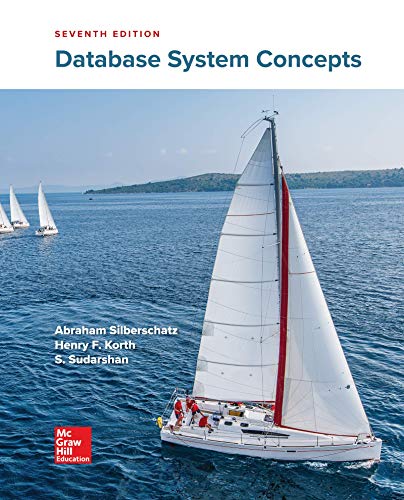
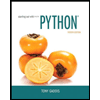
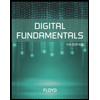
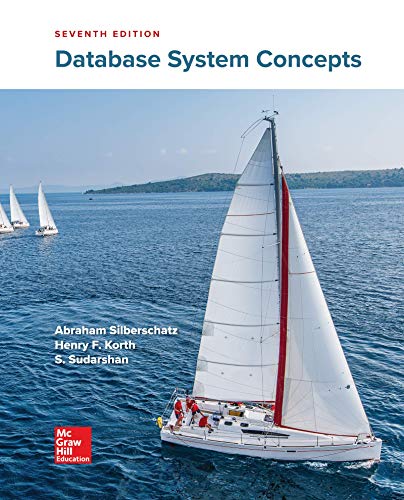
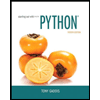
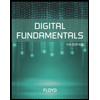
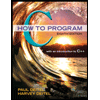
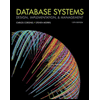
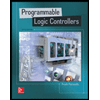