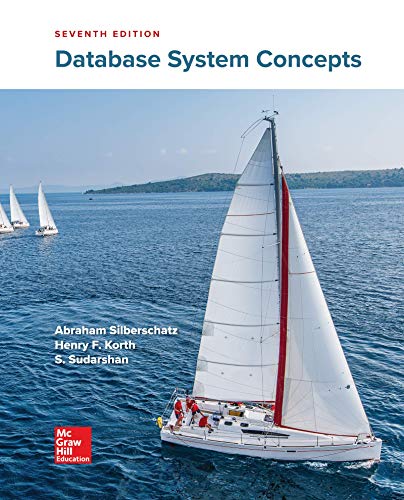
Java Program:
There are errors in the lexer and shank file. Please fix those errors and there must be no error in any of the code at all. Below is the lexer, shank, and token files. The shank file is the main method. There is a rubric attached as well.
Lexer.java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import mypack.Token.TokenType;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int SYMBOL_STATE = 4;
private static final int ERROR_STATE = 5;
private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<String, TokenType>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<Character, TokenType>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=', TokenType.EQUALS);
put(':', TokenType.COLON);
put(';', TokenType.SEMICOLON);
put('(', TokenType.LEFT_PAREN);
put(')', TokenType.RIGHT_PAREN);
put('{', TokenType.LEFT_BRACE);
put('}', TokenType.RIGHT_BRACE);
put('<', TokenType.LESS_THAN);
put('>', TokenType.GREATER_THAN);
}};
public Lexer(String input) {
Lexer.input = input;
index = 0;
currentChar = input.charAt(index);
}
private void nextChar() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
private void skipWhiteSpace() {
while (Character.isWhitespace(currentChar)) {
nextChar();
}
}
private int getIndentLevel() {
int level = 0;
int i = index;
char c = input.charAt(i);
while (c == ' ' || c == '\t') {
if (c == '\t') {
level += 1;
} else if (c == ' ') {
level += 1;
}
i++;
if (i >= input.length()) {
break;
}
Shank.java
package mypack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line);
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
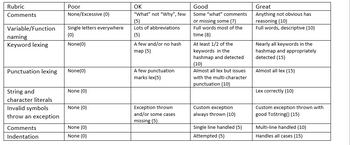

- In the HashMap declaration for the keywords, you can replace the anonymous inner class with a static block.
- In the getIndentLevel method, the variable
level
andi
are never used after being incremented, so there is an infinite loop. - In the same method, you should increment
level
by a specific number of spaces instead of just incrementing by one for each space or tab character. - In the same method, you should break out of the loop when the current character is not a space or tab, not when
i >= input.length()
.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

There are still errors in the lexer and shank files. Please fix those errors and make sure there are no errors in any of the files at all. Attached is an image of the error on the lexer file. There is still an error in the creation of the Lexer object in the main method.
Lexer.java
package mypack;
import java.util.HashMap;
import mypack.Token.TokenType;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int SYMBOL_STATE = 4;
private static final int ERROR_STATE = 5;
private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=', TokenType.EQUALS);
put(':', TokenType.COLON);
put(';', TokenType.SEMICOLON);
put('(', TokenType.LEFT_PAREN);
put(')', TokenType.RIGHT_PAREN);
put('{', TokenType.LEFT_BRACE);
put('}', TokenType.RIGHT_BRACE);
put('<', TokenType.LESS_THAN);
put('>', TokenType.GREATER_THAN);
}};
public Lexer(String input) {
Lexer.input = input;
index = 0;
currentChar = input.charAt(index);
}
private void nextChar() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
private void skipWhiteSpace() {
while (Character.isWhitespace(currentChar)) {
nextChar();
}
}
private int getIndentLevel() {
int level = 0;
int i = index;
char c = input.charAt(i);
while (c == ' ' || c == '\t') {
if (c == '\t') {
level += 1;
} else if (c == ' ') {
level += 1;
}
i++;
if (i >= input.length()) {
break;
}
}
return level;
}
}
Shank.java
package mypack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line);
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
![private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
31
private static final char EOF= (char) -1;
32
33 /**The methods below will allow the user to throw an exception and set up the input, index, current
34 *character, line number, indent level, and the last indent level of the lexer file (lines 37-42)
27
28
29
30
35 */
367 38 39 40 44 2 B4U 45 46 F 48 49
41
42
430
44
47
500
51
52
53
54
55
56
57
58
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put ("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=' TokenType. EQUALS);
put(':', TokenType.COLON);
put(';
TokenType.SEMICOLON);
nut('(' Token Tyne LEFT PAREN).
Problems @Javadoc Declaration Console X
<terminated > Shank (1) [Java Application] C:\Users\subri\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_17.0.1.v2021111
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The method lex (String) is undefined for the type Lexer
at mypack.Shank.main(Shank.java:35)](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/906594c1-1100-49dd-9001-9cca0316a52d/olyszi6a_thumbnail.png)
There are still errors in the lexer and shank files. Please fix those errors and make sure there are no errors in any of the files at all. Attached is an image of the error on the lexer file. There is still an error in the creation of the Lexer object in the main method.
Lexer.java
package mypack;
import java.util.HashMap;
import mypack.Token.TokenType;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int SYMBOL_STATE = 4;
private static final int ERROR_STATE = 5;
private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=', TokenType.EQUALS);
put(':', TokenType.COLON);
put(';', TokenType.SEMICOLON);
put('(', TokenType.LEFT_PAREN);
put(')', TokenType.RIGHT_PAREN);
put('{', TokenType.LEFT_BRACE);
put('}', TokenType.RIGHT_BRACE);
put('<', TokenType.LESS_THAN);
put('>', TokenType.GREATER_THAN);
}};
public Lexer(String input) {
Lexer.input = input;
index = 0;
currentChar = input.charAt(index);
}
private void nextChar() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
private void skipWhiteSpace() {
while (Character.isWhitespace(currentChar)) {
nextChar();
}
}
private int getIndentLevel() {
int level = 0;
int i = index;
char c = input.charAt(i);
while (c == ' ' || c == '\t') {
if (c == '\t') {
level += 1;
} else if (c == ' ') {
level += 1;
}
i++;
if (i >= input.length()) {
break;
}
}
return level;
}
}
Shank.java
package mypack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
public class Shank {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Error: Exactly one argument is required.");
System.exit(0);
}
String filename = args[0];
try {
List<String> lines = Files.readAllLines(Paths.get(filename));
for (String line : lines) {
try {
Lexer lexer = new Lexer(line);
List<Token> tokens = lexer.lex(line);
for (Token token : tokens) {
System.out.println(token);
}
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
} catch (IOException e) {
System.out.println("Error: Could not read file '" + filename + "'.");
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
![private static final int STRING_STATE = 6;
private static final int CHAR_STATE = 7;
private static final int COMMENT_STATE = 8;
31
private static final char EOF= (char) -1;
32
33 /**The methods below will allow the user to throw an exception and set up the input, index, current
34 *character, line number, indent level, and the last indent level of the lexer file (lines 37-42)
27
28
29
30
35 */
367 38 39 40 44 2 B4U 45 46 F 48 49
41
42
430
44
47
500
51
52
53
54
55
56
57
58
private static String input;
private static int index;
private static char currentChar;
private static int lineNumber = 1;
private static int indentLevel = 0;
private static int lastIndentLevel = 0;
private static HashMap<String, TokenType> keywords = new HashMap<>() {{
put("while", TokenType.WHILE);
put("if", TokenType.IF);
put ("else", TokenType.ELSE);
put("print", TokenType.PRINT);
}};
private static HashMap<Character, TokenType> symbols = new HashMap<>() {{
put('+', TokenType.PLUS);
put('-', TokenType.MINUS);
put('*', TokenType.MULTIPLY);
put('/', TokenType.DIVIDE);
put('=' TokenType. EQUALS);
put(':', TokenType.COLON);
put(';
TokenType.SEMICOLON);
nut('(' Token Tyne LEFT PAREN).
Problems @Javadoc Declaration Console X
<terminated > Shank (1) [Java Application] C:\Users\subri\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_17.0.1.v2021111
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The method lex (String) is undefined for the type Lexer
at mypack.Shank.main(Shank.java:35)](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/906594c1-1100-49dd-9001-9cca0316a52d/olyszi6a_thumbnail.png)
- Please answer the question in the screenshot. The language used here is in Java.arrow_forwardJava Program ASAP Please modify Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.HashMap;import java.util.Map;import java.util.Scanner;public class MorseCodeConverter { public static void main(String[] args) { Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text =…arrow_forwardJava Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also I need one one program and please correct and change the program so that the numbers are in the correct places as shown in the correct test case. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.ArrayList;import java.util.Arrays;import java.util.InputMismatchException;import java.util.Scanner;public class FileSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); String fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { ArrayList<String> lines = readFile(fileName); if (lines.isEmpty()) { System.out.println("File " +…arrow_forward
- You will also have to write Student Tests that test the following in the provided file.i. Adding element(s).ii. Get elements at index – (1) with a valid index and (2) with an invalid indexiii. Test equality of two StringArrayLists (of size 0, 2)iv. Test if a StringArrayList contains a String (test both true and false cases)v.Test removing a String from a StringArrayList.arrow_forwardSuppose names is an ABList containing 10 elements. The call names.add(0, "George") results in: A. an exception being thrown. B. a 10-element list with "George" as the first element. C. an 11-element list with "George" as the first element. D. a single element list containing "George". E. None of these is correct.arrow_forwardGiven that an ArrayList of Strings named friendList has already been created and names have already been inserted, write the statement necessary to change the name "Tyler", stored at index 8, to "Bud". This is needed in Javaarrow_forward
- 4. Now examine the ArrayList methods in the above table, a) Which method retrieves elements from the ArrayList? b) Which method replaces the value of an element that already exists in the ArrayList? c) Which two methods initializes the value of an element? d) How do the two methods in (c) differ? Which method(s) would be appropriate in the above Java program (after we convert it to work with ArrayLists)?arrow_forwardFirst, implement a python class called Student. This class should store 2 variables, name and grade. Later, implement a class called Classroom. The constructor of this class should input the list of students in this classrom. This class should also contain 3 methods, add_student, remove_student and calculate_stats. As the names suggest, add_student method should input a Student object and add that to its' already existing student list, remove_student must input a full name(string) and delete the student with the provided name(you can assume no 2 students have the same full name). Finally, calculate_stats method must calculate and print the following statistics of the grades: 1. The name of the student with highest grade and the grade itself. 2. The name of the student with lowest grade and the grade itself. 3. The average gradearrow_forwardInstruction: To test the Linked List class, create a new Java class with the main method, generate Linked List using Integer and check whether all methods do what they’re supposed to do. A sample Java class with main method is provided below including output generated. If you encounter errors, note them and try to correct the codes. Post the changes in your code, if any. Additional Instruction: Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html package com.linkedlist; public class linkedListTester { public static void main(String[] args) { ListI<Integer> list = new LinkedList<Integer>(); int n=10; for(int i=0;i<n;i++) { list.addFirst(i); } for(int…arrow_forward
- New JAVA code can only be added between lines 9 and 10, as seen in image.arrow_forwardI ran the code and got an error. I even created a main.java file to run the test cases. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.arrow_forwardpackage Q2;import java.io.FileInputStream;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Question2 {public static void main(String[] args) throws FileNotFoundException {/*** Part a* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.*/ArrayList<String> NameList;/*** Part b* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description*/FileInputStream inputStream = null;Scanner scnr = new Scanner(inputStream); //Do not modify this line of code/*** Part c* Using a loop and the Scanner object provided, read the names from Names.txt* and store them in NameList created in Part a.*//*** Part d* Reorder the names in the ArrayList so that they appear in reverse alphabetical order.*/// System.out.println("NameList after correct ordering: "…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
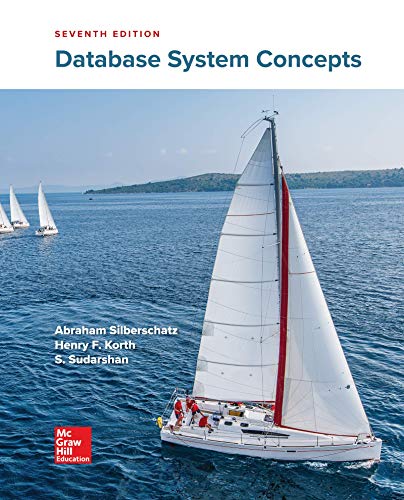
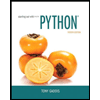
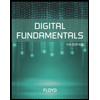
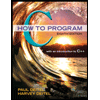
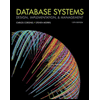
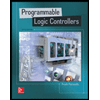