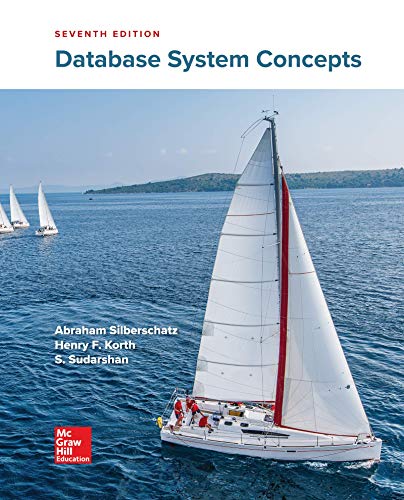
CSCI 3110 Project 2
Write a C++
The program plays “Hearts” with four players: computer 1, computer 2, computer 3, and the user. The winner of the game is the person who has the lowest number of points after the game is over. Points in the game are calculated as follows: each card of HEART suit has points: those with face value less than 10 is worth 5 points each, and the rest of the HEART cards worth 10 points each. In addition, the Queen of Spades is worth 100 points, and the club of Jack is worth -100 points.
Each player is dealt 13 cards at the beginning of the game. The cards held by each player should be sorted by suit. The person who holds 2 of CLUB must start the first hand by playing 2 of CLUB. The suit on the first card played on each hand is called the leading suit for that hand. Then, the remaining three players must each play a card of the same suit if they have one. If not, they can play a card of any other suit. For each hand, the player with the highest valued card of the leading suit must collect the points from that hand. Then, this player starts the next hand in a similar fashion with a new leading suit. Therefore, 13 different “hands” will be played. The game is over after all 13 hands have been played. The player who has the lowest points at the end of the game wins.
Your program simulates this game by showing the remaining cards of the user (in a table form) when it is his/her turn to play. The user is then asked to select a card to play. At each hand:
o no matter who starts the hand, the four players always play in clockwise rotation.
o display the cards played by each player,
o show points accumulated by each player, and
o indicate who will lead the next hand.
The strategy for card selection can be different between the user and the three computers.:
o For the user:
o if the user is to start a hand, he may select any of the remaining card
o if the user is to follow a card, e.g., one of the computer player starts the hand, the
user should select an appropriate card of the leading suit if he has cards of that suit, or select any card to play if he does not have cards of the leading suit.
o Your program needs to verify that the user’s choice is valid given the rule of the
game. If the choice is invalid, prompt the user to enter another choice.
o For each of the three computer players,
o if it is their turn to start a hand, they will always select to play the first of the
remaining cards,
o if they are to follow a card, they always select the first available card of the leading
suit if they have one, or select the first of the remaining cards to play if they do
not have cards of the leading suit.
Note:
o create a header file “mytype.h” that contains the definition of the CardStruct only
o include this file in CardClass.h and PlayerClass.h
You may develop the program in two stages as shown below.
Stage 1
Add the second class “PlayerClass”. The class is used to define the data and related operations
/functions of each player. The header file of the class is given below. You are to implement each
method according to the description given.
Write the client program to do the following only:
o Create a deck of cards in terms of an object of CardClass
o Create four players in terms of an array of 4 objects of PlayerClass.
o Shuffle the cards and deal cards out to the four players in clockwise rotation, one card at a time.
o Each player sorts their cards by suit
o The user displays his cards in table form
o The player who has 2 of Club declares the fact
Test to make sure this stage of the program works correctly before moving on to stage 2.
Stage 2
Complete the “hearts” game program by adding code in the client program with the logic for the
complete game.
Your program should display :
• On each round, which player leads the round, and card played by each player, and
• At the end of the game, the score for each player and who wins the overall game
![// The current score of the player is returned
GetScore() const;
int
// the points from the current round is added to the current player's score
// pre-condition: a point value is sent in from client
// post-condition: the player's score is increased by the points from the client program
void AddScore(int);
// Return the number of cards the player has
//pre-condition: none
//post-condition: the number of cards currently in the player's hand is returned
GetCount() const;
int
// sorts the cards by suit
// pre-condition: there are >=1 cards in hand
//post-condition: the cards are sorted by suit
void Sort();
// plays the card selected by user
// pre-condition: the card number selected by the user is supplied
// post-condition: the card corresponding to the user choice is played/returned the number
//
//
CardStruct PlaySelected Card (int choice);
// Checks to see if the card the user chooses is a valid choice, e.g., whether it matches the
// leading suit on that hand, if he has any.
// pre-condition: the player's choice and the leading suit are supplied
//post-condition: returns true if
of cards in player's hand is decremented by 1. If this card is in the
middle of one's hand, all cards following that card are shifted as a result
of removing the card
private:
//
//
// and returns false otherwise
bool
int
int
(1) user has cards of leading suit and the choice card is of that suit, or
(2) user does not have card of leading suit
CardStruct
#endif
IsValidChoice (suitType, int) const;
hand[MAX_PLAYER_CARDS]; // the set of cards held by the player
count; // keeps record of number of cards player has
score; //keeps score for the player](https://content.bartleby.com/qna-images/question/45088ba1-a1a3-4a96-b462-54f2c0a5a29e/a426e88b-31ba-4c49-9f3b-9fee3e7dbd47/ifndjp_thumbnail.png)
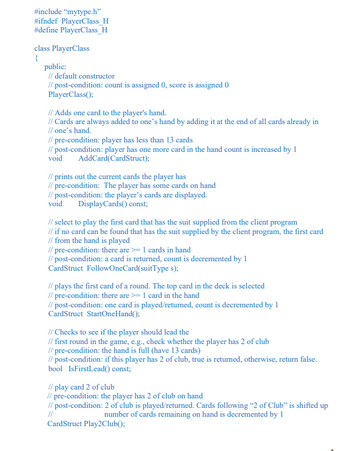

Stage 1
Adding the second class “PlayerClass”. The class is used to define the data and related operations /functions of each player. The header file of the class is given below. You are to implement each method according to the description given.
Write the client program to do the following only: o Create a deck of cards in terms of an object of CardClass o Create four players in terms of an array of 4 objects of PlayerClass. o Shuffle the cards and deal cards out to the four players in clockwise rotation, one card at a time o Each player sorts their cards by suit o The user displays his cards in table form o The player who has 2 of Club declares the fact
Test to make sure this stage of the program works correctly before moving on to stage 2.
Stage 2
Completing the “hearts” game program by adding code in the client program with the logic for the complete game.
Step by stepSolved in 2 steps with 3 images

- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
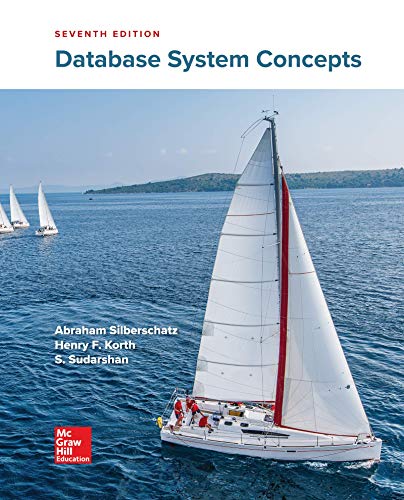
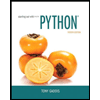
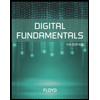
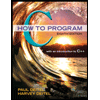
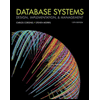
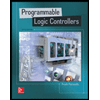