The ROT-13 algorithm encrypts a string one character at a time by adding 13 to the value of the internal representation of the character. We want our encryption scheme to cover the entire printable ASCII range. After examining the ASCII character set and their internal representations, we see that characters 0 through 32 and character 127 are control characters. Technically, characters 32 (space) and 127 (DEL) are printable, but we don’t want to include them in our encryption routine. Therefore, we want our encrypted string to only contain characters in the range of 33 through 126. As an example: Plaintext: Norwich Ciphertext: [|!&vpu The internal representation of ‘N’ is 78. 78 plus 13 is 91, which is the character ‘[‘. The internal representation of ‘o’ is 111. 111 plus 13 is 124, which is the character ‘|’. The internal representation of ‘r’ is 114. 114 plus 13 is 127. Since 126 is our maximum value for our desired printable range, we must use modular arithmetic. Therefore, we get (114+13)%127=0. The character for 0 on the ASCII chart is NULL, which is still not within our desired range. So we have to shift any values less than 33. Therefore, ‘r’ encrypts to ‘!’ which is value 33. Character ‘s’ would be encrypted as ‘"’, which is value 34. The image below helps explain the encryption process. Write a C++ program that prompts the user to enter a password. The program then calls an encryption function that returns a string as the encrypted password. Print the encrypted password to the screen. Note that the printing of the encrypted password should occur inside of main() and not inside of your encryption function. Show your source code and a screenshot of your program output.
- The ROT-13
algorithm encrypts a string one character at a time by adding 13 to the value of the internal representation of the character. We want our encryption scheme to cover the entire printable ASCII range. After examining the ASCII character set and their internal representations, we see that characters 0 through 32 and character 127 are control characters. Technically, characters 32 (space) and 127 (DEL) are printable, but we don’t want to include them in our encryption routine. Therefore, we want our encrypted string to only contain characters in the range of 33 through 126. As an example:
Plaintext: Norwich
Ciphertext: [|!&vpu
The internal representation of ‘N’ is 78. 78 plus 13 is 91, which is the character ‘[‘. The internal representation of ‘o’ is 111. 111 plus 13 is 124, which is the character ‘|’. The internal representation of ‘r’ is 114. 114 plus 13 is 127. Since 126 is our maximum value for our desired printable range, we must use modular arithmetic. Therefore, we get (114+13)%127=0. The character for 0 on the ASCII chart is NULL, which is still not within our desired range. So we have to shift any values less than 33. Therefore, ‘r’ encrypts to ‘!’ which is value 33. Character ‘s’ would be encrypted as ‘"’, which is value 34. The image below helps explain the encryption process.
Write a C++ program that prompts the user to enter a password. The program then calls an encryption function that returns a string as the encrypted password. Print the encrypted password to the screen. Note that the printing of the encrypted password should occur inside of main() and not inside of your encryption function. Show your source code and a screenshot of your program output.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

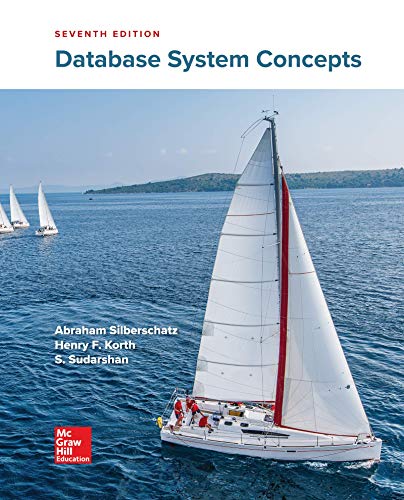
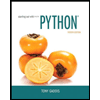
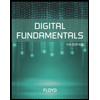
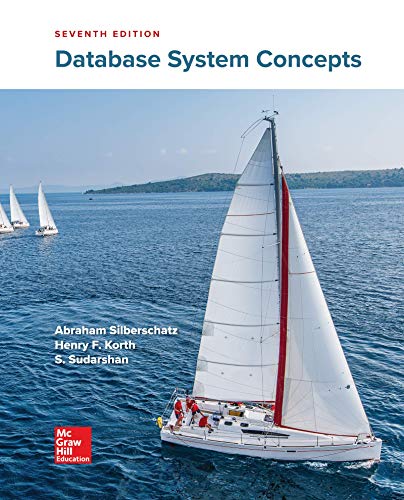
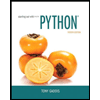
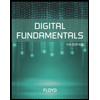
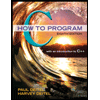
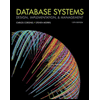
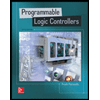