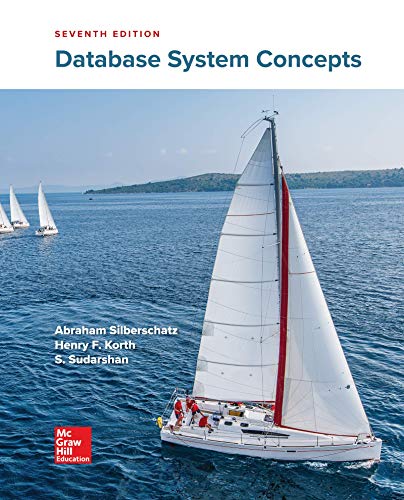
Concept explainers
The
1. Modify Program #1 and Provided code for Program #2:
- Remove the paycheck display part from the code of program 1 (so that you leave only netpay to pass it to program #2
- Modify the program 2 to accept the netpay from program 1 as the startBalance for investment
- set the number of years to 4 years
- set the interest rate at 6% (an integer percent)
3. The report is displayed in tabular form with a header (see output below)
4. Computations and outputs:
- For each year
▪ compute the interest and add it to the investment
▪ print a formatted row of results for that year
5. The total investment and interest earned are also displayed (format the output according the example below
Program 1:
def calcWeeklyWages(totalHours, hourlyWage):
# return the total weekly wages for a worked working totalHours,
# with a given regular hourlyWage. Include overtime for hours over 40
if totalHours <= 40:
totalWages = hourlyWage*totalHours
print("you had no overtime hours this week")
else:
overtime = totalHours - 40
totalWages = hourlyWage*40 + (1.5*hourlyWage)*overtime
print("your overtime hours this week are: ", overtime, "hours")
return totalWages
# main program (that you will need to modify)
hours_in_week1 = float(input('Enter hours worked in first: '))
wage_in_week1 = float(input('Enter dollars paid per hour: '))
week1_earned = calcWeeklyWages(hours_in_week1, wage_in_week1)
hours_in_week2 = float(input('Enter hours worked in second week: '))
wage_in_week2 = float(input('Enter dollars paid per hour in second week: '))
week2_earned = calcWeeklyWages(hours_in_week2, wage_in_week2)
# Modification below:
# 1. Modify the main program section by creating new user defined functions called weeklypay()
# your weeklypay() will prompt the user to enter hours for this week,
# set the wage to 15 and call calcWeeklyWages(totalHours, hourlyWage)
# then return the total pay for that week
# 2. To calculate the weekly pay for week 1 and week 2, call weeklypay() twice (for week 1 & week 2)
print('Wages_in_week1 for {hours_in_week1} hours at ${wage_in_week1:.2f} per hour are ${week1_earned:.2f}.'.format(**locals()))
print('Wages_in_week2 for {hours_in_week2} hours at ${wage_in_week2:.2f} per hour are ${week2_earned:.2f}.'.format(**locals()))
# 3. Add the wages from week 1 and week 2 into a variable called paycheck_amount
total_hours=hours_in_week1+hours_in_week2
total_earned=week1_earned+week2_earned
print('total pay earned for two weeks {total_hours} hours at wages_in_week1 ${wage_in_week1:.2f} and at wages_in_week2 ${wage_in_week2:.2f} per hour are ${total_earned:.2f}.'.format(**locals()))
# 4. Calculate the income tax amount that your employer withhold from your paycheck
# by multiplying paycehck amount * .18 (18 percent)
amount_of_taxes =total_earned*18/100
print('amount of taxes withheld based on 18% of the {total_earned:.2f}. is {amount_of_taxes:.2f}'.format(**locals()))
# 5. Calculate the net pay by taking the income tax amount off the paycheck amount
# 6. Finally, display the earned pay (paycheck amount), tax amount and net pay amount
# based on the output example that you will see
print('earned pay is:',total_earned)
print('taxes withheld:',amount_of_taxes)
print('net pay is:',total_earned-amount_of_taxes);
Program 2: provided code
# Accept the inputs from the user
startBalance = float(input("Enter the investment amount: "))
years = int(input("Enter the number of years: "))
rate = int(input("Enter the rate as a %: "))
# Convert the rate to a decimal number
rate = rate / 100
# Initialize the accumulator for the interest
totalInterest = 0.0
# Display the header for the table
print("%4s%18s%10s%16s" % \
("Year", "Starting balance",
"Interest", "Ending balance"))
# Compute and display the results for each year
for year in range(1, years + 1):
interest = startBalance * rate
endBalance = startBalance + interest
print("%4d%18.2f%10.2f%16.2f" % \
(year, startBalance, interest, endBalance))
startBalance = endBalance
totalInterest += interest
# Display the totals for the period
print("Ending balance: $%0.2f" % endBalance)
print("Total interest earned: $%0.2f" % totalInterest)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In a C program, when is a parameter initialized? Group of answer choices Parameters are initialized automatically when a function is called Parameters are initialized when the program starts Parameters are initialized when the main routine completes Parameters are initialized when the main routine startsarrow_forwardProblem B Musical Key ConversionThe chromatic scale is a 12-note scale in music in which all notes are evenly spaced: that is, the ratio of the frequency between any two consecutive notes is constant. The notes are typically labeled in the following sequence: A, A#, B, C, C#, D, D#, E, F, F#, G, G# After G#, the labels loop back and start over with A (one octave higher). To convert between musical keys, you can shift all notes in a piece of music a constant number of steps along the scale above. For example, the sequence of notes E, E, F, G, G, F, E, D, C, C, D, E, E, D, D can be converted to another musical key by shifting everything up three steps: E, E, F, G, G, F, E, D, C, C, D, E, E, D, D G, G, G#, A#, A#, G#, G, F, D#, D#, F, G, G, F, F Notice that G was converted to A#, since going three steps up required us to loop off of the top of the scale back to the bottom: G -> G# -> A -> A#. Technically we should note that this would be A# of the next octave up, but we’ll…arrow_forwardSummary In this lab, you complete a C++ program with the provided data files. The program calculates the amount of tax withheld from an employee’s weekly salary, the tax deduction to which the employee is entitled for each dependent, and the employee’s take- home pay. The program output includes state tax withheld, federal tax withheld, dependent tax deductions, salary, and take-home pay. Instructions Ensure the source code file named Payroll.cpp is open in the code editor. Variables have been declared and initialized for you as needed, and the input and output statements have been written. Read the code carefully before you proceed to the next step. Write the C++ code needed to perform the following: Calculate state withholding tax (stateTax) at 6.5 percent Calculate federal withholding tax (federalTax) at 28.0 percent. Calculate dependent deductions (dependentDeduction) at 2.5 percent of the employee’s salary for each dependent. Calculate total withholding (totalWithholding)…arrow_forward
- Exercise 3: Perform in C++ Write a program to simulate an experiment rolling two six sided "fair" dice. Allow the user to enter the number of rolls of the dice to simulate. What percentage of the time does the sum of the dots of the dice equal eight in the simulation?arrow_forwardUsing c++ programming, plz complete the coding shown below.arrow_forwardI need help writing a C++ program. I need a function name celsius that accepts a Fahrenheit temperature as an argument and returns the temp in Celsius. I need the function as a loop that displays a table of every fifth Fahrenheit temperature from 0 through 100 and its celsusis equivalent.arrow_forward
- Subject: programming language : c++ Question : Jane has opened a new fitness center with charges of 2500 per month so the cost to become a member of a fitness center is as follow :1.For senior citizens discount is 30 %2. For young one the discount is 15 %3. For adult the discount is 20% Write a menu driven program (using structure c++) thata. Add a new memberb. Display the general information on about the fitness center and its chargesc. Determine the cost of a new membershipd. At any time show the total money made.Use appropriate parameters to pass information on and out of a function.arrow_forwardInstructions C++ 8-17 A company hired 10 temporary workers who are paid hourly and you are given a data file that contains the last name of the employees, the number of hours each employee worked in a week, and the hourly pay rate of each employee. You are asked to write a program that computes each employee’s weekly pay and the average salary of all employees. The program then outputs the weekly pay of each employee, the average weekly pay, and the names of all the employees whose pay is greater than or equal to the average pay. If the number of hours worked in a week is more than 40, then the pay rate for the hours over 40 is 1.5 times the regular hourly rate. Use two parallel arrays: a one-dimensional array to store the names of all the employees (Name) a two-dimensional array of 10 rows and 3 columns to store the number of hours an employee worked in a week (Hrs Worked), the hourly pay rate (Pay Rate), and the weekly pay (Salary). Your program must contain at least the following…arrow_forwardExercise #2 Frequency Analysis: One of the oldest approaches to breaking a code is to perform a frequency count of letters. Write a C++ program to perform a frequency count by reading the text from a file (code.txt). Your program should output how many A's are there in the text, how many B's are there, and so on. Note that the program will not make any distinction between uppercase and lowercase letters. It will output the results to the screen and in a text file (count.txt) Sample output (for code.txt): A: 3 B: 3 C: 23 D: 13 E: 41 F: 4 G: 9 H: 15 1:44 J: 1 K: 0 L: 7 M: 9 N: 33 O: 16 P: 4 Q:0 R: 22 S: 24 T: 27 U: 8 V: 5 W: 1 X: 0 Y: 4 Z: 0arrow_forward
- The parameters passed to function are .called formal parameters True O Fales Oarrow_forwardI am creating a c++ snakes and ladders game (no visuals). I want the game to have up to 5 or 6 players. For the snakes/ladders I am going to create a function which needs the dice roll and adds it to the score which will then decide if the player has landed on a snake or a ladder, is there any way I can get all players scores to be stored in the “score” variable in the switch statements without using multiple switch statements? So I basically want a switch statement which can accommodate all 1-6 players . E.g p1 initially at 1 rolls a 4. So their new score is 1+4=5, then the switch function checks for a ladder or snake. Then player 2 who's initially a 2 rolls a 5, so their new score is 2+5=7 then THE SAME switch function checks for a snake or ladder... And so on for all other players.arrow_forwardUsing a Sentinel Value to Control a while Loop in C++ In this lab, you write a while loop that uses a sentinel value to control a loop in a C++ program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the source code file named MovieGuide.cpp is open in your code editor. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop. The output statements within the loop have already been written for you. Ensure you include the calculations to compute the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
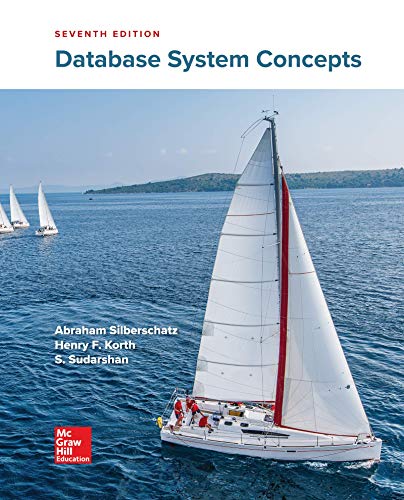
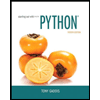
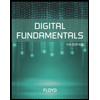
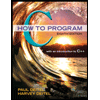
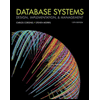
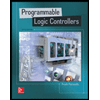