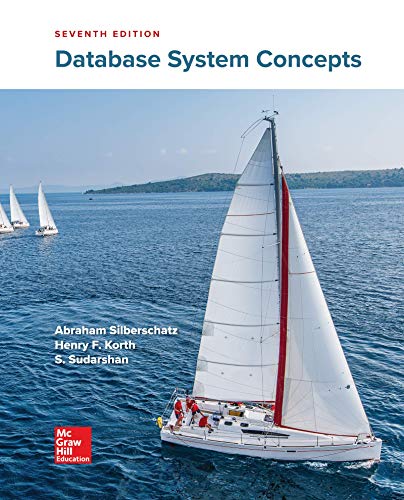
The Problem
The Mastermind game board game is a code breaking game with two players. One player (your program) becomes the codemaker, the other the codebreaker. The codemaker (your program) creates a 4 digit secret code which is randomly generated by the supplied code below and the codebreaker tries to guess the 4 digit pattern.
import random
secretCode =[]
for i in range (0,4):
n = random.randint(1,9)
secretCode.append(str(n))
print (secretCode) # take this out when you are playing the game for real because it is a secret
The secret code pattern generated above will consist of any of the digits 1-9 and can contain multiples of the same digit.
Your program should then tells the codebreaker which digits should be in their guess by displaying a sorted list of the digit characters contained in the secret code python list . Use the loop
for digit in sorted(secretCode):
#display each digit on the same line for the player to see; there could be duplicate digits in the secret
Prompt the codebreaker for a 4 digit pattern guess of valid digits listed (no spaces). Once entered, your program provides feedback by displaying a message stating how many values have been entered correctly (such as 2) but not which ones are in the correct position. To do this, you will have to use a for loop to loop through the list and check each position of the secret code to see if that particular digit character matches the same corresponding position in the string the user entered for their guess.
The player gets 5 guesses and if they do not get the secret code in 5 guesses, they lose. If they guess the secret code, display a congratulatory message.
Requirements:
Your program will play the guessing game as follows:
- Display a sorted list of possible digits for the code that were generated from the randomizing.
- Prompt the codebreaker for a guess.
- Count how many digits were in the correct place.
- End the game if:
- the guess is correct and print out a winner message
- it was the last guess (the fifth) and the guess is incorrect. Print out a loser message and the value of the secret code.
- If no end condition occurs, print out how many of the guess values are exactly right (correct number in the correct position). Example. The secret code is 1234 and the guess is 4132. The feedback would be 1 digit exactly correct.
- Continue the game until an end condition occurs.
Sample Interaction (you may use your own style as long as the required functionality is there)
Let’s play the Mystery code game!
Here are the digits that are valid guesses.
1668
Enter your guess
8166
You guessed 1 correctly
Enter your guess
6816
You guessed 0 correctly
Enter your guess
6861
You guessed 1 correctly
Enter your guess
1668
Congratulations, you won!
Let’s play the Mystery code game!
Here are the digits that are valid guesses.
5578
Enter your guess
7855
You guessed 1 correctly
Enter your guess
5785
You guessed 2 correctly
Enter your guess
5875
You guessed 1 correctly
Enter your guess
5578
You guessed 2 correctly
Enter your guess
5758
Sorry, you loose!

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Each year, the government releases a list of the 10,000 most common baby namesand their frequencies (the number of babies with that name). The only problem with this is that some names have multiple spellings. For example, "John" and ''.Jon" are essentially the same name but would be listed separately in the list. Given two lists, one of names/frequencies and the other of pairs of equivalent names, write an algorithm to print a new list of the true frequency of each name. Note that if John and Jon are synonyms, and Jon and Johnny are synonyms, then John andJohnny are synonyms. (It is both transitive and symmetric.) In the final list, any name can be usedas the "real" name.EXAMPLEInput:Names: John (15), Jon (12), Chris (13), Kris (4), Christopher (19)Synonyms: (Jon, John), (John, Johnny), (Chris, Kris), (Chris, Christopher)Output: John (27), Kris (36)arrow_forwardStart with any positive number n. If n is even divide it by 2, if n is odd multiply by 3 and add 1. Repeat until n becomes 1. The sequence that is generated is called a bumpy sequence. For example, the bumpy sequence that starts at n = 10 is: 10, 5, 16, 8, 4, 2, 1and the one that starts at 11 is11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1 Write a function named lengthBumpy that returns the length of the bumpy sequence generated by its integer argument. The signature of the function is int lengthBumpy(int n) Examples:lengthBumpy(10) returns 7, and lengthBumpy(11) returns 15. You may assume that the argument n is positive and that the sequence converges to 1 without arithmetic overflow.arrow_forwardSolve using JAVA only Given a square chess board of size N, solve the N queens problem. ONLY Print out the NUMBER of solutions for a given N. DO NOT print out or produce the actual solutions, the code shold ONLY PROVIDE the number of correct solutions. Solve for N between 2 and 8. Try your program with 9 and 10, produce those results if it returns in a reasonable amount of time. The N queens problem is to find every configuration of N queens distributed on an NxN square chess board such that all queens are safe from attack by each other.arrow_forward
- Using loops of any kind, lists, sets, def, etc NOT allowed Angela loves reading books. She recently started reading an AI generated series called “Harry Trotter”. Angela is collecting books from the series at her nearest bookstore. Since the series is AI generated, the publishers have produced an infinite collection of the books where each book is identified by a unique integer. The bookstore has exactly one copy of each book. Angela wants to buy the books in the range [l,r], where l ≤ r. As an example, the range [−3,3] means that Angela wants to buy the books − 3, − 2, − 1, 0, 1, 2, and 3. Dan also loves the series (or maybe annoying Angela – who knows, really), and he manages to sneak into the bookstore very early to buy all of the books in the range [d,u], where d ≤ u. When Angela later visits, sadly she will not find those books there anymore. For example, if Angela tries to buy books [−2,3] and Dan has bought books [0,2], Angela would only receive books − 2, − 1, and 3. The…arrow_forwardCan someone help me answer this question?arrow_forwardI need to write a Java ArrayList program called Search.java that counts the names of people that were interviewed from a standard input stream. The program reads people's names from the standard input stream and from time to time prints a sorted portion of the list of names. All the names in the input are given in the order in which they were found and interviewed. You can imagine a unique timestamp associated with each interview. The names are not necessarily unique (many of the people have the same name). Interspersed in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of names to be printed to the standard output stream. The list depends only on the names up to that point in the input stream and does include any people's names that appear in the input stream after the query. The list does not contain all the names of the previous interviews, but only a selection of them. A query provides the names of two people,…arrow_forward
- A palindrome is a string that reads the same forward and backward. For example,“deed” and “level” are palindromes. Write an algorithm in pseudocode that testswhether a string is a palindrome. Implement your algorithm as a static method inJava. The method should call itself recursively and determine if the string is apalindrome.Write a main method that reads a string from the user as input and pass it to thestatic method. You need to validate the string and make sure it contains only letters(no digits or special characters are allowed). The main method should displaywhether the string is a palindrome or not. a) Read the string from the user and validate it.b) Create a method that calls itself recursively and returns whether thestring is a palindrome or not.c) Include a test table with at least the following test cases:1. Invalid input. For example, string contains a number or specialcharacter.2. A valid string that is a palindrome.3. A valid string that is not a palindrome.arrow_forwardThis question is in java The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding. You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range. Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range. Ex List: 20 , 30 , 40 , 75 , 93Number of elements: 5Mean: 51.60Median: 40Range: 73 Grades.java is the main file import java.util.Scanner;public class grades { public static void main(String[] args) { int n; calculation c = new calculation(); int array[] = new int[20]; System.out.print("Enter number of grades that are to be entered: "); Scanner scan = new Scanner(System.in); n = scan.nextInt(); System.out.println("Enter the grades: "); for(int i=0; i<n; i++) { array[i] = scan.nextInt(); if(array[i] < 0) {…arrow_forwarddef findOccurrences(s, ch): lst = [] for i in range(0, len(s)): if a==s[i]: lst.append(i) return lst Use the code above instead of enumerate in the code posted below. n=int(input("Number of rounds of Hangman to be played:")) for i in range(0,n): word = input("welcome!") guesses = '' turns = int(input("Enter the number of failed attempts allowed:")) def hangman(word): secrete_word = "-" * len(word) print(" the secrete word " + secrete_word) user_input = input("Guess a letter: ") if user_input in word: occurences = findOccurrences(word, user_input) for index in occurences: secrete_word = secrete_word[:index] + user_input + secrete_word[index + 1:] print(secrete_word) else: user_input = input("Sorry that letter was not found, please try again: ") def findOccurrences(s, ch): return [i for i, letter in enumerate(s) if letter == ch] *** enumerate not discussed in…arrow_forward
- Use the ArrayList class Add and remove objects from an ArrayList Protect from index errors when removing Practice with input loop Details: This homework is for you to get practice adding and removing objects from an ArrayList. The Voter class was used to create instances of Voters which held their name and a voter identification number as instance variables, and the number of instances created as a static variable. This was the class diagram: The constructor takes a string, passed to the parameter n, which is the name of the voter and which should be assigned to the name instance variable. Every time a new voter is created, the static variable nVoters should be incremented. Also, every time a new voter is created, a new voterID should be constructed by concatenating the string “HI” with the value of nVoters and the length of the name. For example, if the second voter is named “Clark Kent”, then the voterID should be “HI210” because 2 is the value of nVoters and 10 is the number…arrow_forwardwe showed you the code#for two versions of binary_search: one using recursion, one#using loops. For this problem, use the recursive one.##In this problem, we want to implement a new version of#binary_search, called binary_search_year. binary_search_year#will take in two parameters: a list of instances of Date,#and a year as an integer. It will return True if any date#in the list occurred within that year, False if not.##For example, imagine if listOfDates had three instances of#date: one for January 1st 2016, one for January 1st 2017,#and one for January 1st 2018. Then:## binary_search_year(listOfDates, 2016) -> True# binary_search_year(listOfDates, 2015) -> False##You should not assume that the list is pre-sorted, but you#should know that the sort() method works on lists of dates.##Instances of the Date class have three attributes: year,#month, and day. You can access them directly, you don't#have to use getters (e.g. myDate.month will access the#month of myDate).##You may…arrow_forwardWrite a program that uses a recursive call to find the integer logb of a number. Where logb returns the integer log of a number in a designated base. For example, the integer base 10 log of 1234 is 3, and the integer base 2 log of 1234 is 10. This is a relatively easy calculation. You simply repeatedly divide the number by the base using integer division until the quotient is less than the base and count the number of completed divisions. 1234/10=123 (1) 123/10 12 (2) 12/10 = 1 (3) 1234/2= 617 (1) 617/2 = 308 (2) 308/2 = 154 (3) 154/2 = 77 (4) 77/2 = 38 (5) 38/2 = 19 (6) 19/2 = 9 (7) 9/2=4 (8) 4/2=2(9) 2/2 = 1 (10)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
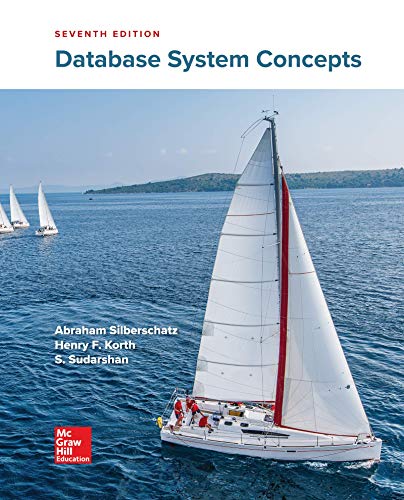
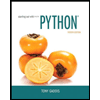
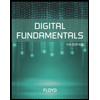
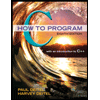
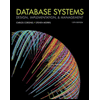
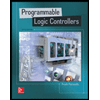