PYTHON Import the green channel of the following image URL (make sure your code works for this URL): https://raw.githubusercontent.com/dpploy/chen-3170/master/notebooks/images/nerve-cells.jpg as a matrix A intended for linear algebra operations and show the matrix as an image as below (make sure the data is normalized). Show the complete data type specification of the matrix including maximum, minimum, average, and standard deviation values.
PYTHON
Import the green channel of the following image URL (make sure your code works for this URL):
https://raw.githubusercontent.com/dpploy/chen-3170/master/notebooks/images/nerve-cells.jpg
as a matrix A intended for linear algebra operations and show the matrix as an image as below (make sure the data is normalized). Show the complete data type specification of the matrix including maximum, minimum, average, and standard deviation values.


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Compute the LU factorization of the symmetric part of A(100*100)and verify that your factorization is correct; explain. The symmetric part of a matrix sym(A) is defined as
sym(A) =( A+ A^T)/2
Make your best visual demonstration (art contest) that it (the sym(A)
matrix) is indeed symmetric.
The LU factorization program code is :
def lu_factorization(A):
2 """
3 Performs LU factorization of a matrix A without pivoting.
4
5 Args:
6 A: A square matrix.
7
8 Returns:
9 A tuple (L, U) where L is the lower triangular matrix and U is the upper triangular matrix.
10 """
11 n = len(A)
12 L = [[0.0] * n for _ in range(n)]
13 U = [[0.0] * n for _ in range(n)]
14
15 for k in range(n - 1):
16 for i in range(k + 1, n):
17
18 mik = A[i][k] / A[k][k]
19 L[i][k] = mik
20
21 for j in range(k + 1, n):
22 A[i][j] = A[i][j] - mik * A[k][j]
23
24 U[k][i] = mik
25
26 for i in range(n):
27 L[i][i] = 1.0
28
29 return L, U
30
31A = [[1.0, 2.0, 3.0],
32 [4.0, 5.0, 6.0],
33 [7.0, 8.0, 9.0]]
34
35L, U = lu_factorization(A)
36
37print("L:")
38for row in L:
39 print(row)
40
41print("U:")
42for row in U:
43 print(row)
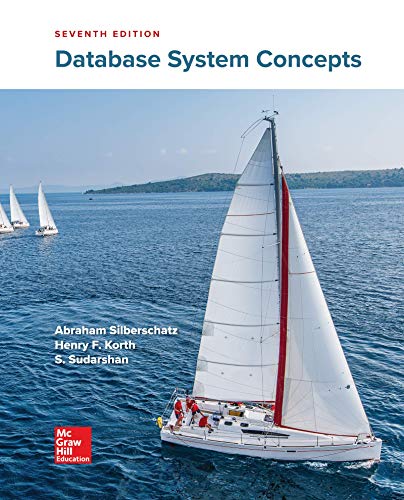
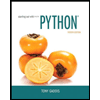
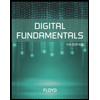
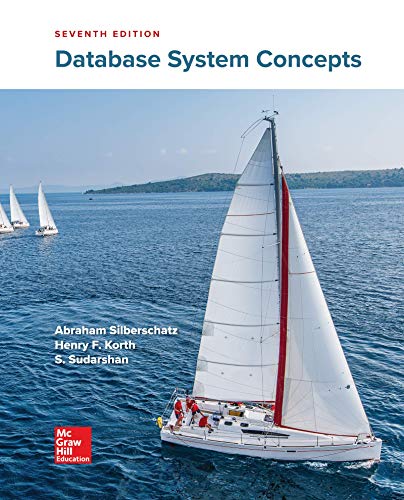
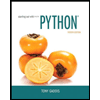
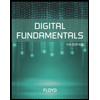
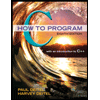
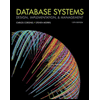
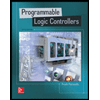