The greatest common divisor of two positive integers, A and B, is the largest number that can be evenly divided into both of them. Euclid's algorithm can be used to find the greatest common divisor (GCD) of two positive integers. You can use this algorithm in the following manner: 1. Compute the remainder of dividing the larger number by the smaller number. 2. Replace the larger number with the smaller number and the smaller number with the remainder. 3. Repeat this process
The greatest common divisor of two positive integers, A and B, is the largest number that can be evenly divided into both of them. Euclid's algorithm can be used to find the greatest common divisor (GCD) of two positive integers. You can use this algorithm in the following manner: 1. Compute the remainder of dividing the larger number by the smaller number. 2. Replace the larger number with the smaller number and the smaller number with the remainder. 3. Repeat this process
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The greatest common divisor of two positive integers, A and B, is the largest number that can be evenly divided into both of them. Euclid's algorithm can be used to find the greatest common divisor (GCD) of two positive integers. You can use this algorithm in the following manner:
1. Compute the remainder of dividing the larger number by the smaller number.
2. Replace the larger number with the smaller number and the smaller number with the
remainder.
3. Repeat this process until the smaller number is zero.
The larger number at this point is the GCD of A and B. Write a program that lets the user enter two integers and then prints each step in the process of using the Euclidean algorithm to find their
GCD.
An example of the program input and output is shown below:
Enter the smaller number: 5
Enter the larger number: 15
The greatest common divisor is 5

Transcribed Image Text:mming Exercise 3.8
Instructions
gcd.py
The greatest common divisor of two positive integers, A and B, is the largest number that can
1 # Put your code here
evenly divided into both of them. Euclid's algorithm can be used to find the greatest common
divisor (GCD) of two positive integers. You can use this algorithm in the following manner:
1. Compute the remainder of dividing the larger number by the smaller number.
2. Replace the larger number with the smaller number and the smaller number with the
remainder.
3. Repeat this process until the smaller number is zero.
The larger number at this point is the GCD of A and B. Write a program that lets the user enter two
integers and then prints each step in the process of using the Euclidean algorithm to find their
GCD.
An example of the program input and output is shown below:
Enter the smaller number: 5
Enter the larger number: 15
The greatest common divisor is 5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
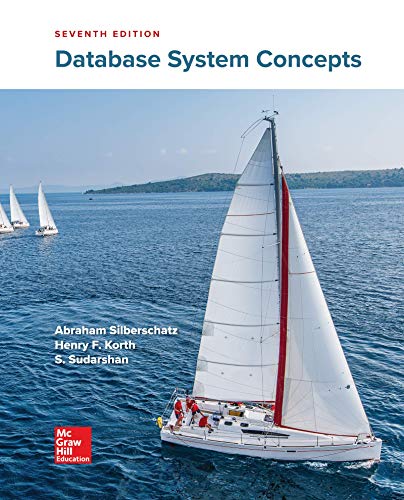
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
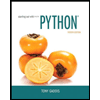
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
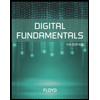
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
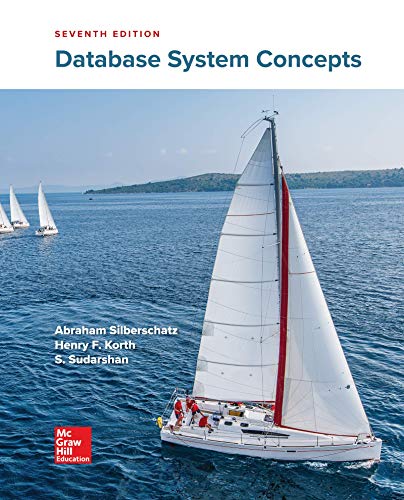
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
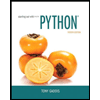
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
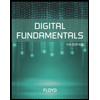
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
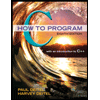
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
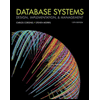
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
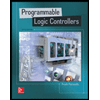
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education