The following program is designed to input two numbers and output their sum. It asks the user if he/she would like to run the program. If the answer is Y or y, it prompts the user to enter two numbers. After adding the numbers and displaying the results, it again asks the user if he/she would like to add more numbers. However, the program fails to do so. Correct the program so that it works properly. #include #include using namespace std; int main() { char response; double num1; double num2; cout << "This program adds two numbers." << endl; cout << "Would you like to run the program: (Y/y) "; cin >> response; cout << endl; cout << fixed << showpoint << setprecision(2); while (response == 'Y' && response == 'y') { cout << "Enter two numbers: "; cin >> num1 >> num2; cout << endl; cout << num1 << " + " << num2 << " = " << (num1 – num2) << endl; cout << "Would you like to add again: (Y/y) "; cin >> response; cout << endl; } return 0; }
The following
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
char response;
double num1;
double num2;
cout << "This program adds two numbers." << endl;
cout << "Would you like to run the program: (Y/y) ";
cin >> response;
cout << endl;
cout << fixed << showpoint << setprecision(2);
while (response == 'Y' && response == 'y')
{
cout << "Enter two numbers: ";
cin >> num1 >> num2;
cout << endl;
cout << num1 << " + " << num2 << " = " << (num1 – num2)
<< endl;
cout << "Would you like to add again: (Y/y) ";
cin >> response;
cout << endl;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Write a for loop to reads 100 marks of students. The program must then find the sum and average mark of the 100 students
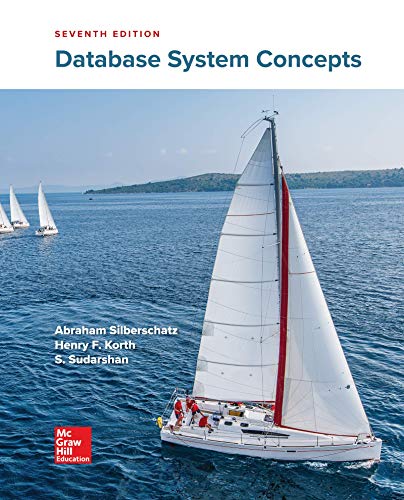
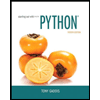
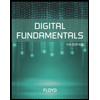
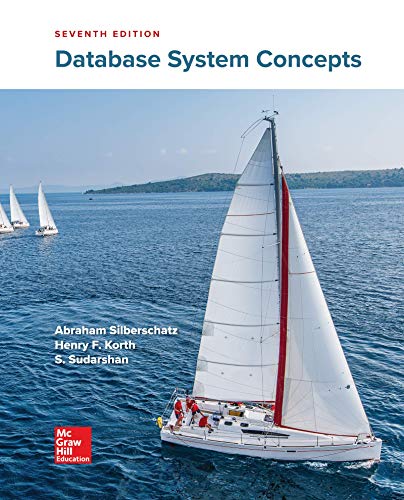
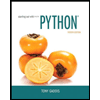
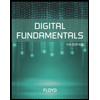
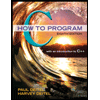
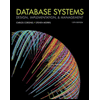
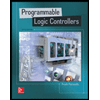