The following is a Java code for a Design Patterns: Strategy Pattern in object oriented design programming. Execute the code in Netbeans and provide screenshots to verify the code runs successfully, Draw a UML class diagram to model the program. File : Billing.java public interface Billing { public double getDiscount();} File : LoyaltyBilling.java public class LoyaltyBilling implements Billing{ @Override public double getDiscount() { return 20.00; } } File : NormalBilling.java public class NormalBilling implements Billing{ @Override public double getDiscount() { return 0; } } File : SeasonalBilling.java public class SeasonalBilling implements Billing{ @Override public double getDiscount() { return 10; } } File :SaleBilling.java public class SaleBilling implements Billing{ @Override public double getDiscount() { return 50; } } File : Product.java public class Product { private String name; private double price; public Product(String name, double price) { this.name = name; this.price = price; } public String getName() { return name; } public double getPrice() { return price; } public void setName(String name) { this.name = name; } public void setPrice(double price) { this.price = price; } } File: Sale.java import java.util.ArrayList; public class Sale { private Billing billing; private ArrayList<Product> products; public Sale(Billing billing, ArrayList<Product> products) { this.billing = billing; this.products = products; } public double getDiscountPercentage(){ return billing.getDiscount(); } public double calculateCost() { double total = 0; for(int i=0; i<products.size();i++){ total = total+products.get(i).getPrice(); } total = total - (getDiscountPercentage()/100)*total; return total; }} File: Shop.java import java.text.ParseException;import java.text.SimpleDateFormat;import java.util.ArrayList;import java.util.Date;import java.util.Scanner; public class Shop { Product[] shopProducts; Sale newSale; Scanner in = new Scanner(System.in); public void initilaizeProducts(){ shopProducts = new Product[10]; shopProducts[0] = new Product("Rice", 10); shopProducts[1] = new Product("Sugar", 8); shopProducts[2] = new Product("Pasta", 2); shopProducts[3] = new Product("Coffee", 3); shopProducts[4] = new Product("Tea", 3); shopProducts[5] = new Product("Banana", 10); shopProducts[6] = new Product("Onion", 8); shopProducts[7] = new Product("Cucumber", 2); shopProducts[8] = new Product("Carrot", 3); shopProducts[9] = new Product("Chilly", 3); } public void startBilling(){ System.out.print("Enter date:(dd/mm/yyyy): "); String date = in.next(); try { Date date1=new SimpleDateFormat("dd/MM/yyyy").parse(date); } catch (ParseException ex) { System.err.println("Error in date entry"); } ArrayList<Product> scannedProducts = new ArrayList<Product>(); System.out.println("PRODUCTS"); for(int i=0;i<shopProducts.length;i++){ System.out.println(" "+(i+1)+". "+shopProducts[i].getName()+" $"+shopProducts[i].getPrice()); } System.out.println(" "+(shopProducts.length+1)+". Exit"); int choice; do{ System.out.print("Enter choice: "); choice = in.nextInt(); if(choice<1 || choice>(shopProducts.length)) break; scannedProducts.add(shopProducts[choice-1]); }while(choice>0 && choice<(shopProducts.length+1)); System.out.println("Select billing"); System.out.println("1. Loyalty Billing"); System.out.println("2. Normal Billing"); System.out.println("3. Seasonal Billing"); System.out.println("4. Sale Billing"); choice = in.nextInt(); switch(choice){ case 1: newSale = new Sale(new LoyaltyBilling(), scannedProducts); break; case 2: newSale = new Sale(new NormalBilling(), scannedProducts); break; case 3: newSale = new Sale(new SeasonalBilling(), scannedProducts); break; case 4: newSale = new Sale(new SaleBilling(), scannedProducts); break; default: System.out.println("Invalid entry"); } System.out.printf("Total Cost: $%.2f",newSale.calculateCost()); } public static void main(String[] args) { Shop s = new Shop(); s.initilaizeProducts(); s.startBilling(); }}
The following is a Java code for a Design Patterns: Strategy Pattern in object oriented design programming. Execute the code in Netbeans and provide screenshots to verify the code runs successfully, Draw a UML class diagram to model the program. File : Billing.java public interface Billing { public double getDiscount();} File : LoyaltyBilling.java public class LoyaltyBilling implements Billing{ @Override public double getDiscount() { return 20.00; } } File : NormalBilling.java public class NormalBilling implements Billing{ @Override public double getDiscount() { return 0; } } File : SeasonalBilling.java public class SeasonalBilling implements Billing{ @Override public double getDiscount() { return 10; } } File :SaleBilling.java public class SaleBilling implements Billing{ @Override public double getDiscount() { return 50; } } File : Product.java public class Product { private String name; private double price; public Product(String name, double price) { this.name = name; this.price = price; } public String getName() { return name; } public double getPrice() { return price; } public void setName(String name) { this.name = name; } public void setPrice(double price) { this.price = price; } } File: Sale.java import java.util.ArrayList; public class Sale { private Billing billing; private ArrayList<Product> products; public Sale(Billing billing, ArrayList<Product> products) { this.billing = billing; this.products = products; } public double getDiscountPercentage(){ return billing.getDiscount(); } public double calculateCost() { double total = 0; for(int i=0; i<products.size();i++){ total = total+products.get(i).getPrice(); } total = total - (getDiscountPercentage()/100)*total; return total; }} File: Shop.java import java.text.ParseException;import java.text.SimpleDateFormat;import java.util.ArrayList;import java.util.Date;import java.util.Scanner; public class Shop { Product[] shopProducts; Sale newSale; Scanner in = new Scanner(System.in); public void initilaizeProducts(){ shopProducts = new Product[10]; shopProducts[0] = new Product("Rice", 10); shopProducts[1] = new Product("Sugar", 8); shopProducts[2] = new Product("Pasta", 2); shopProducts[3] = new Product("Coffee", 3); shopProducts[4] = new Product("Tea", 3); shopProducts[5] = new Product("Banana", 10); shopProducts[6] = new Product("Onion", 8); shopProducts[7] = new Product("Cucumber", 2); shopProducts[8] = new Product("Carrot", 3); shopProducts[9] = new Product("Chilly", 3); } public void startBilling(){ System.out.print("Enter date:(dd/mm/yyyy): "); String date = in.next(); try { Date date1=new SimpleDateFormat("dd/MM/yyyy").parse(date); } catch (ParseException ex) { System.err.println("Error in date entry"); } ArrayList<Product> scannedProducts = new ArrayList<Product>(); System.out.println("PRODUCTS"); for(int i=0;i<shopProducts.length;i++){ System.out.println(" "+(i+1)+". "+shopProducts[i].getName()+" $"+shopProducts[i].getPrice()); } System.out.println(" "+(shopProducts.length+1)+". Exit"); int choice; do{ System.out.print("Enter choice: "); choice = in.nextInt(); if(choice<1 || choice>(shopProducts.length)) break; scannedProducts.add(shopProducts[choice-1]); }while(choice>0 && choice<(shopProducts.length+1)); System.out.println("Select billing"); System.out.println("1. Loyalty Billing"); System.out.println("2. Normal Billing"); System.out.println("3. Seasonal Billing"); System.out.println("4. Sale Billing"); choice = in.nextInt(); switch(choice){ case 1: newSale = new Sale(new LoyaltyBilling(), scannedProducts); break; case 2: newSale = new Sale(new NormalBilling(), scannedProducts); break; case 3: newSale = new Sale(new SeasonalBilling(), scannedProducts); break; case 4: newSale = new Sale(new SaleBilling(), scannedProducts); break; default: System.out.println("Invalid entry"); } System.out.printf("Total Cost: $%.2f",newSale.calculateCost()); } public static void main(String[] args) { Shop s = new Shop(); s.initilaizeProducts(); s.startBilling(); }}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The following is a Java code for a Design Patterns: Strategy Pattern in object oriented design
Draw a UML class diagram to model the program.
File : Billing.java
public interface Billing {
public double getDiscount();
}
}
File : LoyaltyBilling.java
public class LoyaltyBilling implements Billing{
@Override
public double getDiscount() {
return 20.00;
}
@Override
public double getDiscount() {
return 20.00;
}
}
File : NormalBilling.java
public class NormalBilling implements Billing{
@Override
public double getDiscount() {
return 0;
}
public double getDiscount() {
return 0;
}
}
File : SeasonalBilling.java
public class SeasonalBilling implements Billing{
@Override
public double getDiscount() {
return 10;
}
public double getDiscount() {
return 10;
}
}
File :SaleBilling.java
public class SaleBilling implements Billing{
@Override
public double getDiscount() {
return 50;
}
public double getDiscount() {
return 50;
}
}
File : Product.java
public class Product {
private String name;
private double price;
private String name;
private double price;
public Product(String name, double price) {
this.name = name;
this.price = price;
}
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
return name;
}
public double getPrice() {
return price;
}
return price;
}
public void setName(String name) {
this.name = name;
}
this.name = name;
}
public void setPrice(double price) {
this.price = price;
}
}
this.price = price;
}
}
File: Sale.java
import java.util.ArrayList;
public class Sale {
private Billing billing;
private ArrayList<Product> products;
private ArrayList<Product> products;
public Sale(Billing billing, ArrayList<Product> products) {
this.billing = billing;
this.products = products;
}
public double getDiscountPercentage(){
return billing.getDiscount();
}
public double calculateCost() {
double total = 0;
for(int i=0; i<products.size();i++){
total = total+products.get(i).getPrice();
}
total = total - (getDiscountPercentage()/100)*total;
return total;
}
}
this.billing = billing;
this.products = products;
}
public double getDiscountPercentage(){
return billing.getDiscount();
}
public double calculateCost() {
double total = 0;
for(int i=0; i<products.size();i++){
total = total+products.get(i).getPrice();
}
total = total - (getDiscountPercentage()/100)*total;
return total;
}
}
File: Shop.java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Scanner;
public class Shop {
Product[] shopProducts;
Sale newSale;
Scanner in = new Scanner(System.in);
public void initilaizeProducts(){
shopProducts = new Product[10];
shopProducts[0] = new Product("Rice", 10);
shopProducts[1] = new Product("Sugar", 8);
shopProducts[2] = new Product("Pasta", 2);
shopProducts[3] = new Product("Coffee", 3);
shopProducts[4] = new Product("Tea", 3);
shopProducts[5] = new Product("Banana", 10);
shopProducts[6] = new Product("Onion", 8);
shopProducts[7] = new Product("Cucumber", 2);
shopProducts[8] = new Product("Carrot", 3);
shopProducts[9] = new Product("Chilly", 3);
}
public void startBilling(){
System.out.print("Enter date:(dd/mm/yyyy): ");
String date = in.next();
try {
Date date1=new SimpleDateFormat("dd/MM/yyyy").parse(date);
} catch (ParseException ex) {
System.err.println("Error in date entry");
}
ArrayList<Product> scannedProducts = new ArrayList<Product>();
System.out.println("PRODUCTS");
for(int i=0;i<shopProducts.length;i++){
System.out.println(" "+(i+1)+". "+shopProducts[i].getName()+" $"+shopProducts[i].getPrice());
}
System.out.println(" "+(shopProducts.length+1)+". Exit");
int choice;
do{
System.out.print("Enter choice: ");
choice = in.nextInt();
if(choice<1 || choice>(shopProducts.length))
break;
scannedProducts.add(shopProducts[choice-1]);
}while(choice>0 && choice<(shopProducts.length+1));
System.out.println("Select billing");
System.out.println("1. Loyalty Billing");
System.out.println("2. Normal Billing");
System.out.println("3. Seasonal Billing");
System.out.println("4. Sale Billing");
choice = in.nextInt();
switch(choice){
case 1:
newSale = new Sale(new LoyaltyBilling(), scannedProducts);
break;
case 2:
newSale = new Sale(new NormalBilling(), scannedProducts);
break;
case 3:
newSale = new Sale(new SeasonalBilling(), scannedProducts);
break;
case 4:
newSale = new Sale(new SaleBilling(), scannedProducts);
break;
default:
System.out.println("Invalid entry");
}
System.out.printf("Total Cost: $%.2f",newSale.calculateCost());
}
public static void main(String[] args) {
Shop s = new Shop();
s.initilaizeProducts();
s.startBilling();
}
}
Product[] shopProducts;
Sale newSale;
Scanner in = new Scanner(System.in);
public void initilaizeProducts(){
shopProducts = new Product[10];
shopProducts[0] = new Product("Rice", 10);
shopProducts[1] = new Product("Sugar", 8);
shopProducts[2] = new Product("Pasta", 2);
shopProducts[3] = new Product("Coffee", 3);
shopProducts[4] = new Product("Tea", 3);
shopProducts[5] = new Product("Banana", 10);
shopProducts[6] = new Product("Onion", 8);
shopProducts[7] = new Product("Cucumber", 2);
shopProducts[8] = new Product("Carrot", 3);
shopProducts[9] = new Product("Chilly", 3);
}
public void startBilling(){
System.out.print("Enter date:(dd/mm/yyyy): ");
String date = in.next();
try {
Date date1=new SimpleDateFormat("dd/MM/yyyy").parse(date);
} catch (ParseException ex) {
System.err.println("Error in date entry");
}
ArrayList<Product> scannedProducts = new ArrayList<Product>();
System.out.println("PRODUCTS");
for(int i=0;i<shopProducts.length;i++){
System.out.println(" "+(i+1)+". "+shopProducts[i].getName()+" $"+shopProducts[i].getPrice());
}
System.out.println(" "+(shopProducts.length+1)+". Exit");
int choice;
do{
System.out.print("Enter choice: ");
choice = in.nextInt();
if(choice<1 || choice>(shopProducts.length))
break;
scannedProducts.add(shopProducts[choice-1]);
}while(choice>0 && choice<(shopProducts.length+1));
System.out.println("Select billing");
System.out.println("1. Loyalty Billing");
System.out.println("2. Normal Billing");
System.out.println("3. Seasonal Billing");
System.out.println("4. Sale Billing");
choice = in.nextInt();
switch(choice){
case 1:
newSale = new Sale(new LoyaltyBilling(), scannedProducts);
break;
case 2:
newSale = new Sale(new NormalBilling(), scannedProducts);
break;
case 3:
newSale = new Sale(new SeasonalBilling(), scannedProducts);
break;
case 4:
newSale = new Sale(new SaleBilling(), scannedProducts);
break;
default:
System.out.println("Invalid entry");
}
System.out.printf("Total Cost: $%.2f",newSale.calculateCost());
}
public static void main(String[] args) {
Shop s = new Shop();
s.initilaizeProducts();
s.startBilling();
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 18 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
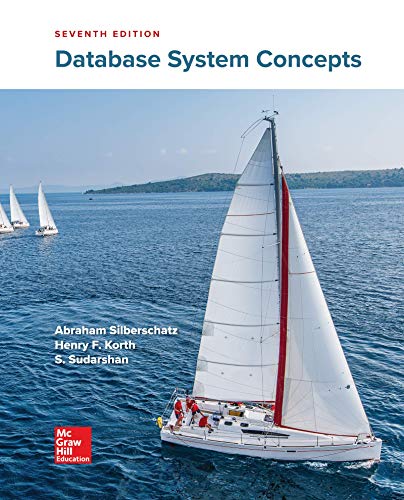
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
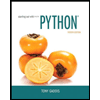
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
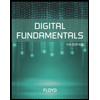
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
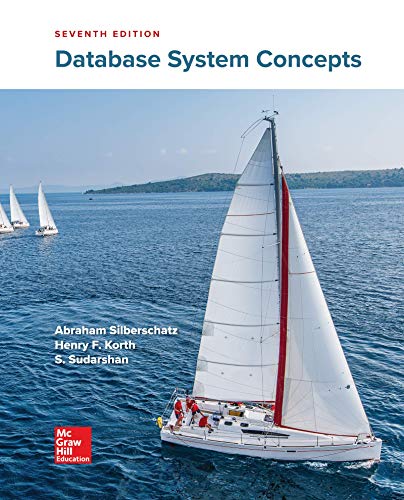
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
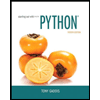
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
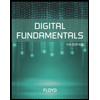
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
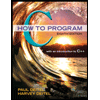
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
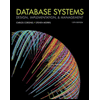
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
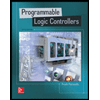
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education