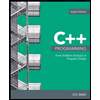
The Code must be in Java
Write a program to calculate the following for each integer from 0 to 35:
- Print the current integer and one set of calculated values per line.
- Calculate the following for each value from 0 to 35:
- The square of the integer
- The cube of the integer
- The Fibonacci Number which occupies that integer’s position in the Fibonacci number sequence
Given that the first few numbers in the Fibonacci sequence are 1, 1, 2, 3, 5, 8, 13 and 21, for the integer 6 the output would be “6 36 216 8”. Print a header or title line for the table to show what each of the columns represents (abbreviate “square” to “sqr” for simplicity.) The table headers and numeric output should line up nicely.
You must write the Fibonacci series part of the assignment with an ArrayList. The point of this exercise is to practice with ArrayLists. Everything you need to know about ArrayLists to do this was in the lecture and slides. The other parts are simple multiplication operations. You shouldn’t use ArrayLists for them-just for the Fibonacci Sequence. Create and fill the ArrayList first and then use a loop to generate the output.
Once you have the program running properly, add the necessary code to it to send the output to a file named “Lab10.out.txt” on the computer.
If you were to put the output of this program in Notepad, it may not appear to line up properly even though it lines up nicely in the Eclipse console. This warning is not telling you to submit the output.
Do not create a Class to hold all the data. You are only using most of it once, so create the ArrayList of Fibonacci numbers and then just calculate the other values as you print them

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- (Numerical) Using the srand() and rand() C++ library functions, fill an array of 1000 floating-point numbers with random numbers that have been scaled to the range 1 to 100. Then determine and display the number of random numbers having values between 1 and 50 and the number having values greater than 50. What do you expect the output counts to be?arrow_forward(Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. Each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs, increment the counter variable that’s keeping count of the 1s that occur; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.arrow_forwardThe program in the Programming Example: Fibonacci Number does not check whether the first number entered by the user is less than or equal to the second number and whether both the numbers are non-negative. Also, the program does not check whether the user entered a valid value for the position of the desired number in the Fibonacci sequence. Rewrite that program so that it checks for these things.arrow_forward
- (Statistical) In many statistical analysis programs, data values considerably outside the range of the majority of values are simply dropped from consideration. Using this information, write a C++ program that accepts up to 10 floating-point values from a user and determines and displays the average and standard deviation of the input values. All values more than four standard deviations away from the computed average are to be displayed and dropped from any further calculation, and a new average and standard deviation should be computed and displayed.arrow_forwardWhen you borrow money to buy a house, a car, or for some other purpose, you repay the loan by making periodic payments over a certain period of time. Of course, the lending company will charge interest on the loan. Every periodic payment consists of the interest on the loan and the payment toward the principal amount. To be specific, suppose that you borrow $1,000 at an interest rate of 7.2% per year and the payments are monthly. Suppose that your monthly payment is $25. Now, the interest is 7.2% per year and the payments are monthly, so the interest rate per month is 7.2/12 = 0.6%. The first months interest on $1,000 is 1000 0.006 = 6. Because the payment is $25 and the interest for the first month is $6, the payment toward the principal amount is 25 6 = 19. This means after making the first payment, the loan amount is 1,000 19 = 981. For the second payment, the interest is calculated on $981. So the interest for the second month is 981 0.006 = 5.886, that is, approximately $5.89. This implies that the payment toward the principal is 25 5.89 = 19.11 and the remaining balance after the second payment is 981 19.11 = 961.89. This process is repeated until the loan is paid. Write a program that accepts as input the loan amount, the interest rate per year, and the monthly payment. (Enter the interest rate as a percentage. For example, if the interest rate is 7.2% per year, then enter 7.2.) The program then outputs the number of months it would take to repay the loan. (Note that if the monthly payment is less than the first months interest, then after each payment, the loan amount will increase. In this case, the program must warn the borrower that the monthly payment is too low, and with this monthly payment, the loan amount could not be repaid.)arrow_forward(Numerical) Write and test a function that returns the position of the largest and smallest values in an array of double-precision numbers.arrow_forward
- 4. During each summer, John and Jessica grow vegetables in their backyard and buy seeds and fertilizer from a local nursery. The nursery carries different types of vegetable fertilizers in various bag sizes. When buying a particular fertilizer, they want to know the price of the fertilizer per pound and the cost of fertilizing per square foot. The following program prompts the user to enter the size of the fertilizer bag, in pounds, the cost of the bag, and the area, in square feet, that can be covered by the bag. The program should output the desired result. However, the program contains logic errors. Find and correct the logic errors so that the program works properly. // Logic errors. #include #include using namespace std; int main() { double costs double area; double bagsize; cout > bagsize; cout > cost; cout > area; cout << endl; cout << "The cost of the fertilizer per pound is: $" << bagsize / cost << endl; cout << "The cost of fertilizing per square foot is: $" << area / cost << endl; return 0; }arrow_forward(Data processing) Your professor has asked you to write a C++ program that determines grades at the end of the semester. For each student, identified by an integer number between 1 and 60, four exam grades must be kept, and two final grade averages must be computed. The first grade average is simply the average of all four grades. The second grade average is computed by weighting the four grades as follows: The first grade gets a weight of 0.2, the second grade gets a weight of 0.3, the third grade gets a weight of 0.3, and the fourth grade gets a weight of 0.2. That is, the final grade is computed as follows: 0.2grade1+0.3grade2+0.3grade3+0.2grade4 Using this information, construct a 60-by-7 two-dimensional array, in which the first column is used for the student number, the next four columns for the grades, and the last two columns for the computed final grades. The program’s output should be a display of the data in the completed array. For testing purposes, the professor has provided the following data:arrow_forwardWhen you perform arithmetic operations with operands of different types, such as adding an int and a float, ____________. C# chooses a unifying type for the result you must choose a unifying type for the result you must provide a cast you receive an error messagearrow_forward
- (Computation) A magic square is a square of numbers with N rows and N columns, in which each integer value from 1 to (N * N) appears exactly once, and the sum of each column, each row, and each diagonal is the same value. For example, Figure 7.21 shows a magic square in which N=3, and the sum of the rows, columns, and diagonals is 15. Write a program that constructs and displays a magic square for a given odd number N. This is the algorithm:arrow_forwardChoose the best data type for each of the following, so that no memory storage is wasted. Give an example of a typical value that would be held by the variable, and explain why you chose the type you did. the number of years of school you have completed your final grade in this class the population of China the number of passengers on an airline flight one players score in a Scrabble game the number of Electoral College votes received by a U.S. presidential candidate the number of days with below freezing temperatures in a winter in Miami, Florida one teams score in a Major League Baseball gamearrow_forward(Numerical) Given a one-dimensional array of integer numbers, write and test a function that displays the array elements in reverse order.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
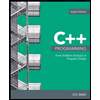
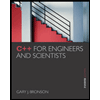
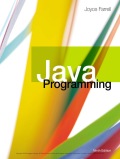
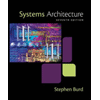
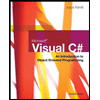