The Caesar Cipher: Privacy in communication has been an issue ever since the dawn of time. To prevent people from stealing information, we can "Encrypt" and "Decrypt" it in several ways. To encrypt something means that the message gets translated into a secret code that only the people involved can understand, decrypt is the opposite procedure. One of the oldest methods of encryption is called the Caesar Cipher Named after the dictator Julius Caesar). It consists of assigning every letter a number value and writing the word as a sequence of numbers. Consider the Caesar cipher where each character gets assigned its value in the ASCII code. a 97 b C d c с f 98 99 100 101 102 g h i 103 j k 1 104 105 106 107 108 109 11 110 0 P q r S 111 112 113 114 115 116 t 117 V 118 W 119 120 121 2122 u X y The problem is to create a C++ program that receives an encrypted message, translates it, then receives an answer from the u English and encrypts it. To do this and get full marks you are to create 5 functions, both the function prototypes and the main unction are provided. A function that reads the input array. . A function that translates the input and prints the translated message. . . A function that reads a string and returns it. hi there A function that receives a string of letters and encrypts it, returning an array. . A function that prints the answer array. Input: The first number N it receives is the length of the received code, then it receives a sequence of Nintegers. Afte translated message it should read your answer to be translated. Example: Input 5 104 101 108 108 111 1 Output M hello Assume it always receives a valid input this is N>1 all numbers in the original code are betwee 104 105 32 116 104 101 114 101
The Caesar Cipher: Privacy in communication has been an issue ever since the dawn of time. To prevent people from stealing information, we can "Encrypt" and "Decrypt" it in several ways. To encrypt something means that the message gets translated into a secret code that only the people involved can understand, decrypt is the opposite procedure. One of the oldest methods of encryption is called the Caesar Cipher Named after the dictator Julius Caesar). It consists of assigning every letter a number value and writing the word as a sequence of numbers. Consider the Caesar cipher where each character gets assigned its value in the ASCII code. a 97 b C d c с f 98 99 100 101 102 g h i 103 j k 1 104 105 106 107 108 109 11 110 0 P q r S 111 112 113 114 115 116 t 117 V 118 W 119 120 121 2122 u X y The problem is to create a C++ program that receives an encrypted message, translates it, then receives an answer from the u English and encrypts it. To do this and get full marks you are to create 5 functions, both the function prototypes and the main unction are provided. A function that reads the input array. . A function that translates the input and prints the translated message. . . A function that reads a string and returns it. hi there A function that receives a string of letters and encrypts it, returning an array. . A function that prints the answer array. Input: The first number N it receives is the length of the received code, then it receives a sequence of Nintegers. Afte translated message it should read your answer to be translated. Example: Input 5 104 101 108 108 111 1 Output M hello Assume it always receives a valid input this is N>1 all numbers in the original code are betwee 104 105 32 116 104 101 114 101
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++ language

Transcribed Image Text:The Caesar Cipher:
Privacy in communication has been an issue ever since the dawn of time. To prevent people from stealing information, we can
"Encrypt" and "Decrypt" it in several ways. To encrypt something means that the message gets translated into a secret code that
only the people involved can understand, decrypt is the opposite procedure.
One of the oldest methods of encryption is called the Caesar Cipher Named after the dictator Julius Caesar). It consists of
assigning every letter a number value and writing the word as a sequence of numbers.
Consider the Caesar cipher where each character gets assigned its value in the ASCII code.
a
b
C
d
C
с
f
97
98
99
100
101
102
g
h
i
103
j
k
1
104
105
106
107
108
109
11 110
0 111
Р 112
q 113
Г 114
115
t 116
S
u 117
V
W
x
y
118
119
120
121
2122
The problem is to create a C++ program that receives an encrypted message, translates it, then receives an answer from the u
English and encrypts it. To do this and get full marks you are to create 5 functions, both the function prototypes and the main
unction are provided.
A function that reads the input array.
.
A function that translates the input and prints the translated message.
.
.
A function that reads a string and returns it.
.
A function that receives a string of letters and encrypts it, returning an array.
.
A function that prints the answer array.
Input:
The first number N it receives is the length of the received code, then it receives a sequence of Nintegers. Afte
translated message it should read your answer to be translated.
Example:
hi there
Input
5
104 101 108 108 111 1
Output
hello
Assume it always receives a valid input this is N>1 all numbers in the original code are betwee
104 105 32 116 104 101 114 101
![Input
5
104 101 108 108 111
should read your answer to be translated
gth of the received code, then it receives a sequence
hi there
1
104 105 32 116 104 101 114 101
Assume it always receives a valid input, this is, N 2 1, all numbers in the original code are between 0 and 26 and all the characters in
the response are either a space or lowercase letters.
#include <iostream>
#include <string>
using namespace std;
Output
int main() {
//function prototypes
void read_array (int input [], int size);
void translate_to_english (int input, int size);
hello
string read_answer ();
void encrypt (string answer, int encrypted_ans []);
void print_array(int array], int size);
//define and read the received array
int array_size;
cin >> array_size;
int A[ array_size];
read_array(A, array_size );
//decode the received message
translate_to_english( A, array_size);
cin.ignore (100,'\n');
answer = read_answer();
//define and read the answer from the user
string answer;
//clears the buffer
//encode the answer into an array of numbers
int answer array [answer.length()];
encrypt( answer, answer_array);
print_array( answer_array, answer.length() );
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fea26996e-a928-4e4d-b4fd-9751c0983a4f%2F88d30507-9237-4470-bd84-b4c957a30821%2Fp93bw_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Input
5
104 101 108 108 111
should read your answer to be translated
gth of the received code, then it receives a sequence
hi there
1
104 105 32 116 104 101 114 101
Assume it always receives a valid input, this is, N 2 1, all numbers in the original code are between 0 and 26 and all the characters in
the response are either a space or lowercase letters.
#include <iostream>
#include <string>
using namespace std;
Output
int main() {
//function prototypes
void read_array (int input [], int size);
void translate_to_english (int input, int size);
hello
string read_answer ();
void encrypt (string answer, int encrypted_ans []);
void print_array(int array], int size);
//define and read the received array
int array_size;
cin >> array_size;
int A[ array_size];
read_array(A, array_size );
//decode the received message
translate_to_english( A, array_size);
cin.ignore (100,'\n');
answer = read_answer();
//define and read the answer from the user
string answer;
//clears the buffer
//encode the answer into an array of numbers
int answer array [answer.length()];
encrypt( answer, answer_array);
print_array( answer_array, answer.length() );
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
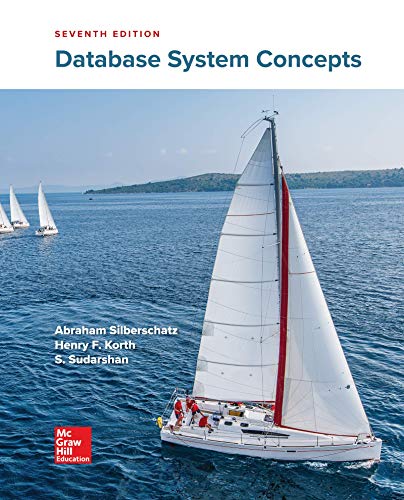
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
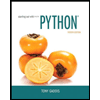
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
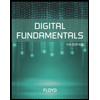
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
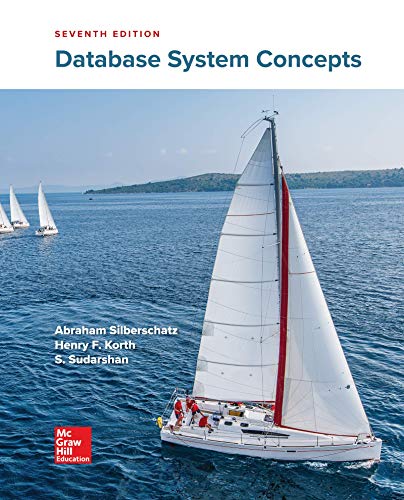
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
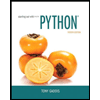
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
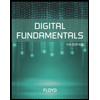
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
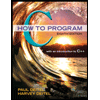
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
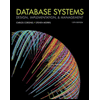
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
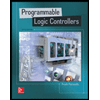
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education