the array and test for a match. You also need to set a flag if there is a match, and then test the flag variable to determine if you should print the "Not a city in Michigan" message. Comments in the code tell you where to write your statements. Instructions f Ⓒ 1. Study the prewritten code to make sure you understand it. 2. Write a loop statement that examines the names of cities stored in the array. 3. Write code that tests for a match. 4. Write code that, when appropriate, prints the message: "Not a city in Michigan.". 5. Execute the program using the following as input: Chicago Brooklyn Watervliet Acme Grading When you have completed your program, click the Submit button to record your score. 8 public class MichiganCities 9 { 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 public static void main(String args[]) throws Exception { E ▬▬ // Declare variables. String inCity; // name of city to look up in array. // Initialized array of cities in Michigan. String cities In Michigan[] = ["Acme", "Albion", "Detroit", "Watervliet", "Colom "Richland", "Glenn","Midland", "Brooklyn"}; boolean foundIt = false; // Flag variable. // Loop control variable. int x; // Get user input. Scanner input = new Scanner(System.in); System.out.println("Enter the name of the city: "); inCity = input.nextLine(); // Write your loop here. // Write your test statement here to see if there is // a match. Set the flag to true if city is found. } // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed. System.exit(0); 40 } // End of main() method. 41} // End of MichiganCities class. 1 W ± Q Search 16
the array and test for a match. You also need to set a flag if there is a match, and then test the flag variable to determine if you should print the "Not a city in Michigan" message. Comments in the code tell you where to write your statements. Instructions f Ⓒ 1. Study the prewritten code to make sure you understand it. 2. Write a loop statement that examines the names of cities stored in the array. 3. Write code that tests for a match. 4. Write code that, when appropriate, prints the message: "Not a city in Michigan.". 5. Execute the program using the following as input: Chicago Brooklyn Watervliet Acme Grading When you have completed your program, click the Submit button to record your score. 8 public class MichiganCities 9 { 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 public static void main(String args[]) throws Exception { E ▬▬ // Declare variables. String inCity; // name of city to look up in array. // Initialized array of cities in Michigan. String cities In Michigan[] = ["Acme", "Albion", "Detroit", "Watervliet", "Colom "Richland", "Glenn","Midland", "Brooklyn"}; boolean foundIt = false; // Flag variable. // Loop control variable. int x; // Get user input. Scanner input = new Scanner(System.in); System.out.println("Enter the name of the city: "); inCity = input.nextLine(); // Write your loop here. // Write your test statement here to see if there is // a match. Set the flag to true if city is found. } // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed. System.exit(0); 40 } // End of main() method. 41} // End of MichiganCities class. 1 W ± Q Search 16
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![اماء
the array and test for a match. You also need to set
a flag if there is a match, and then test the flag
variable to determine if you should print the "Not a
city in Michigan" message. Comments in the code
tell you where to write your statements.
Instructions
go
Ⓒ
1. Study the prewritten code to make sure you
understand it.
2. Write a loop statement that examines the names
of cities stored in the array.
3. Write code that tests for a match.
4. Write code that, when appropriate, prints the
message: "Not a city in Michigan.".
5. Execute the program using the following as
input:
Chicago
Brooklyn
Watervliet
Acme
Grading
When you have completed your program, click the
Submit button to record your score.
8 public class MichiganCities
9 {
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
1
public static void main(String args[]) throws Exception
{
EE
// Declare variables.
String inCity;
// name of city to look up in array.
// Initialized array of cities in Michigan.
String cities InMichigan[] = ["Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Sagina
"Richland", "Glenn","Midland", "Brooklyn";
boolean foundIt = false; // Flag variable.
int x;
// Loop control variable.
// Get user input.
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the city: ");
inCity = input.nextLine();
// Write your loop here.
// Write your test statement here to see if there is
// a match. Set the flag to true if city is found.
}
39
40 } // End of main() method.
41} // End of MichiganCities class.
// Test to see if city was not found to determine if
// "Not a city in Michigan" message should be printed.
System.exit(0);
Q Search
16](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd37f1519-9bca-4c3f-95f7-bdfad11980b2%2F23bfa8d9-b1db-4c3b-8a6d-819e873d433d%2Flq3exsk_processed.jpeg&w=3840&q=75)
Transcribed Image Text:اماء
the array and test for a match. You also need to set
a flag if there is a match, and then test the flag
variable to determine if you should print the "Not a
city in Michigan" message. Comments in the code
tell you where to write your statements.
Instructions
go
Ⓒ
1. Study the prewritten code to make sure you
understand it.
2. Write a loop statement that examines the names
of cities stored in the array.
3. Write code that tests for a match.
4. Write code that, when appropriate, prints the
message: "Not a city in Michigan.".
5. Execute the program using the following as
input:
Chicago
Brooklyn
Watervliet
Acme
Grading
When you have completed your program, click the
Submit button to record your score.
8 public class MichiganCities
9 {
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
1
public static void main(String args[]) throws Exception
{
EE
// Declare variables.
String inCity;
// name of city to look up in array.
// Initialized array of cities in Michigan.
String cities InMichigan[] = ["Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Sagina
"Richland", "Glenn","Midland", "Brooklyn";
boolean foundIt = false; // Flag variable.
int x;
// Loop control variable.
// Get user input.
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the city: ");
inCity = input.nextLine();
// Write your loop here.
// Write your test statement here to see if there is
// a match. Set the flag to true if city is found.
}
39
40 } // End of main() method.
41} // End of MichiganCities class.
// Test to see if city was not found to determine if
// "Not a city in Michigan" message should be printed.
System.exit(0);
Q Search
16
![L06.2-Searching an Array for an Exact Match in Java
B|
YE
</>
Searching an Array for an Exact
Match
The data file provided for this lab includes the input
statements and the necessary variable declarations.
You need to use a loop to examine all the items in
the array and test for a match. You also need to set
a flag if there is a match, and then test the flag
variable to determine if you should print the "Not a
city in Michigan" message. Comments in the code
tell you where to write your statements.
f
Ⓒ
✪
Instructions
1. Study the prewritten code to make sure you
understand it.
2. Write a loop statement that examines the names
of cities stored in the array.
3. Write code that tests for a match.
4. Write code that, when appropriate, prints the
message: "Not a city in Michigan.".
5. Execute the program using the following as
input:
Chicago
Brooklyn
Watervliet
Acme
Grading
MichiganCities.java
1 // MichiganCities.java
2 // Input: Interactive.
3 // Output: Error message or nothing.
4
5
24
25
6 import java.util.Scanner;
7
8 public class MichiganCities
9 {
10 public static void main(String args[]) throws Exception
11
{
12
13
14
15
16
17
18
19
20
21
22
23
26
27
28
29
30
31
32
33
34
35
36
FH
+
// Declare variables.
String inCity;
// Initialized array of cities in Michigan.
String citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw",
"Richland", "Glenn","Midland", "Brooklyn"};
boolean foundIt = false; // Flag variable.
// Loop control variable.
int x;
}
This program prints a message for invalid cities in Michigan.
// Get user input.
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the city: ");
inCity input.nextLine();
// Write your loop here.
// Write your test statement here to see if there is
// a match. Set the flag to true if city is found.
£
Q Search
// name of city to look up in array.
// Test to see if city was not found to determine if
// "Not a city in Michigan" message should be printed.
System.exit(0);
16
Q Sear
dx
12
10/1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd37f1519-9bca-4c3f-95f7-bdfad11980b2%2F23bfa8d9-b1db-4c3b-8a6d-819e873d433d%2Fe5jmx1_processed.jpeg&w=3840&q=75)
Transcribed Image Text:L06.2-Searching an Array for an Exact Match in Java
B|
YE
</>
Searching an Array for an Exact
Match
The data file provided for this lab includes the input
statements and the necessary variable declarations.
You need to use a loop to examine all the items in
the array and test for a match. You also need to set
a flag if there is a match, and then test the flag
variable to determine if you should print the "Not a
city in Michigan" message. Comments in the code
tell you where to write your statements.
f
Ⓒ
✪
Instructions
1. Study the prewritten code to make sure you
understand it.
2. Write a loop statement that examines the names
of cities stored in the array.
3. Write code that tests for a match.
4. Write code that, when appropriate, prints the
message: "Not a city in Michigan.".
5. Execute the program using the following as
input:
Chicago
Brooklyn
Watervliet
Acme
Grading
MichiganCities.java
1 // MichiganCities.java
2 // Input: Interactive.
3 // Output: Error message or nothing.
4
5
24
25
6 import java.util.Scanner;
7
8 public class MichiganCities
9 {
10 public static void main(String args[]) throws Exception
11
{
12
13
14
15
16
17
18
19
20
21
22
23
26
27
28
29
30
31
32
33
34
35
36
FH
+
// Declare variables.
String inCity;
// Initialized array of cities in Michigan.
String citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw",
"Richland", "Glenn","Midland", "Brooklyn"};
boolean foundIt = false; // Flag variable.
// Loop control variable.
int x;
}
This program prints a message for invalid cities in Michigan.
// Get user input.
Scanner input = new Scanner(System.in);
System.out.println("Enter the name of the city: ");
inCity input.nextLine();
// Write your loop here.
// Write your test statement here to see if there is
// a match. Set the flag to true if city is found.
£
Q Search
// name of city to look up in array.
// Test to see if city was not found to determine if
// "Not a city in Michigan" message should be printed.
System.exit(0);
16
Q Sear
dx
12
10/1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
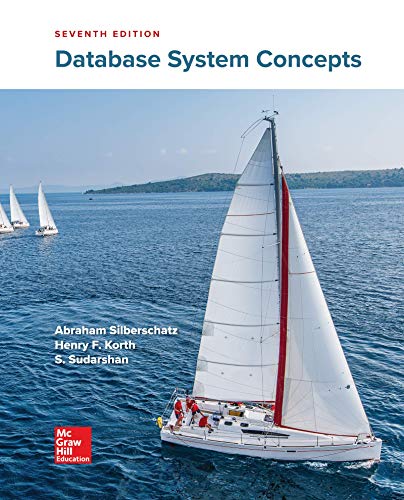
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
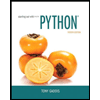
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
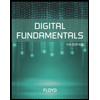
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
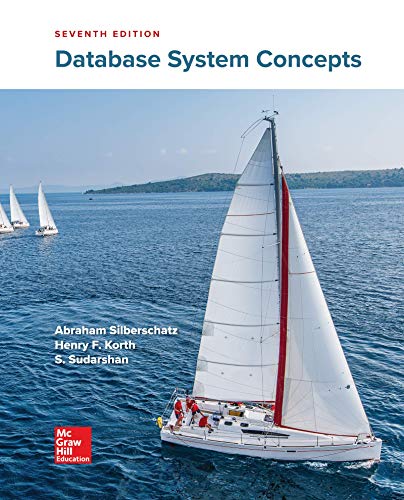
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
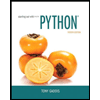
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
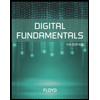
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
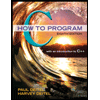
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
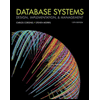
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
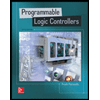
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education