Test it by: Add numbers from 13 to 23 in the queue. Print the length of the queue Check if the queue is empty Show the item at the front of the queue Remove the first item in the queue Add 100 to the queue Remove all items in the queue Check if the queue is empty
Test it by: Add numbers from 13 to 23 in the queue. Print the length of the queue Check if the queue is empty Show the item at the front of the queue Remove the first item in the queue Add 100 to the queue Remove all items in the queue Check if the queue is empty
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python help.
"""
File: linkedqueue.py
Author: Ken Lambert
"""
from node import Node
from abstractcollection import AbstractCollection
class LinkedQueue(AbstractCollection):
"""A link-based queue implementation."""
# Constructor
def __init__(self, sourceCollection = None):
"""Sets the initial state of self, which includes the
contents of sourceCollection, if it's present."""
self.front = self.rear = None
AbstractCollection.__init__(self, sourceCollection)
# Accessor methods
def __iter__(self):
"""Supports iteration over a view of self."""
pass
def peek(self):
"""
Returns the item at the front of the queue.
Precondition: the queue is not empty.
Raises: KeyError if the stack is empty."""
if self.isEmpty():
raise KeyError("The queue is empty.")
return self.front.data
# Mutator methods
def clear(self):
"""Makes self become empty."""
pass
def add(self, item):
"""Adds item to the rear of the queue."""
newNode = Node(item, None)
if self.isEmpty():
self.front = newNode
else:
self.rear.next = newNode
self.rear = newNode
self.size += 1
def pop(self):
"""
Removes and returns the item at the front of the queue.
Precondition: the queue is not empty.
Raises: KeyError if the queue is empty.
Postcondition: the front item is removed from the queue."""
if self.isEmpty():
raise KeyError("The queue is empty.")
oldItem = self.front.data
self.front = self.front.next
if self.front is None:
self.rear = None
Write a LikedQueue (code at bottom)
Test it by:
- Add numbers from 13 to 23 in the queue.
- Print the length of the queue
- Check if the queue is empty
- Show the item at the front of the queue
- Remove the first item in the queue
- Add 100 to the queue
- Remove all items in the queue
- Check if the queue is empty
"""
File: linkedqueue.py
Author: Ken Lambert
"""
from node import Node
from abstractcollection import AbstractCollection
class LinkedQueue(AbstractCollection):
"""A link-based queue implementation."""
# Constructor
def __init__(self, sourceCollection = None):
"""Sets the initial state of self, which includes the
contents of sourceCollection, if it's present."""
self.front = self.rear = None
AbstractCollection.__init__(self, sourceCollection)
# Accessor methods
def __iter__(self):
"""Supports iteration over a view of self."""
pass
def peek(self):
"""
Returns the item at the front of the queue.
Precondition: the queue is not empty.
Raises: KeyError if the stack is empty."""
if self.isEmpty():
raise KeyError("The queue is empty.")
return self.front.data
# Mutator methods
def clear(self):
"""Makes self become empty."""
pass
def add(self, item):
"""Adds item to the rear of the queue."""
newNode = Node(item, None)
if self.isEmpty():
self.front = newNode
else:
self.rear.next = newNode
self.rear = newNode
self.size += 1
def pop(self):
"""
Removes and returns the item at the front of the queue.
Precondition: the queue is not empty.
Raises: KeyError if the queue is empty.
Postcondition: the front item is removed from the queue."""
if self.isEmpty():
raise KeyError("The queue is empty.")
oldItem = self.front.data
self.front = self.front.next
if self.front is None:
self.rear = None
self.size -= 1
return oldItem
"
return oldItem
"
"""
File: node.py
Node classes for one-way linked structures and two-way
linked structures.
"""
class Node(object):
def __init__(self, data, next = None):
"""Instantiates a Node with default next of None"""
self.data = data
self.next = next
class TwoWayNode(Node):
def __init__(self, data, previous = None, next = None):
Node.__init__(self, data, next)
self.previous = previous
# Just an empty link
node1 = None
# A node containing data and an empty link
node2 = Node("A", None)
# A node containing data and a link to node2
node3 = Node("B", node2)
File: node.py
Node classes for one-way linked structures and two-way
linked structures.
"""
class Node(object):
def __init__(self, data, next = None):
"""Instantiates a Node with default next of None"""
self.data = data
self.next = next
class TwoWayNode(Node):
def __init__(self, data, previous = None, next = None):
Node.__init__(self, data, next)
self.previous = previous
# Just an empty link
node1 = None
# A node containing data and an empty link
node2 = Node("A", None)
# A node containing data and a link to node2
node3 = Node("B", node2)
"
"
"
"""
File: abstractcollection.py
Author: Ken Lambert
"""
class AbstractCollection(object):
"""An abstract collection implementation."""
# Constructor
def __init__(self, sourceCollection = None):
"""Sets the initial state of self, which includes the
contents of sourceCollection, if it's present."""
self.size = 0
if sourceCollection:
for item in sourceCollection:
self.add(item)
# Accessor methods
def isEmpty(self):
"""Returns True if len(self) == 0, or False otherwise."""
return len(self) == 0
def __len__(self):
"""Returns the number of items in self."""
return self.size
def __str__(self):
"""Returns the string representation of self."""
return "[" + ", ".join(map(str, self)) + "]"
def __add__(self, other):
"""Returns a new bag containing the contents
of self and other."""
result = type(self)(self)
for item in other:
result.add(item)
return result
def __eq__(self, other):
"""Returns True if self equals other,
or False otherwise."""
if self is other: return True
if type(self) != type(other) or \
len(self) != len(other):
return False
otherIter = iter(other)
for item in self:
if item != next(otherIter):
return False
return True
def count(self, item):
"""Returns the number of instances of item in self."""
total = 0
for nextItem in self:
if nextItem == item:
total += 1
return total
File: abstractcollection.py
Author: Ken Lambert
"""
class AbstractCollection(object):
"""An abstract collection implementation."""
# Constructor
def __init__(self, sourceCollection = None):
"""Sets the initial state of self, which includes the
contents of sourceCollection, if it's present."""
self.size = 0
if sourceCollection:
for item in sourceCollection:
self.add(item)
# Accessor methods
def isEmpty(self):
"""Returns True if len(self) == 0, or False otherwise."""
return len(self) == 0
def __len__(self):
"""Returns the number of items in self."""
return self.size
def __str__(self):
"""Returns the string representation of self."""
return "[" + ", ".join(map(str, self)) + "]"
def __add__(self, other):
"""Returns a new bag containing the contents
of self and other."""
result = type(self)(self)
for item in other:
result.add(item)
return result
def __eq__(self, other):
"""Returns True if self equals other,
or False otherwise."""
if self is other: return True
if type(self) != type(other) or \
len(self) != len(other):
return False
otherIter = iter(other)
for item in self:
if item != next(otherIter):
return False
return True
def count(self, item):
"""Returns the number of instances of item in self."""
total = 0
for nextItem in self:
if nextItem == item:
total += 1
return total
"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
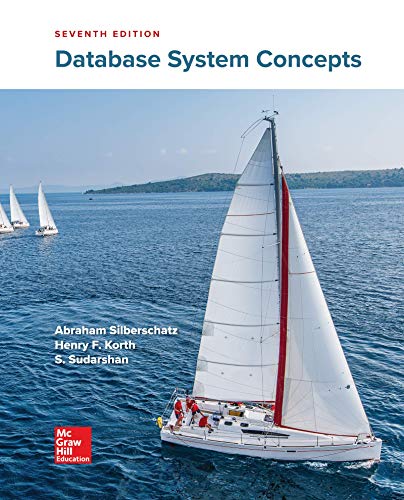
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
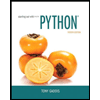
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
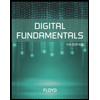
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
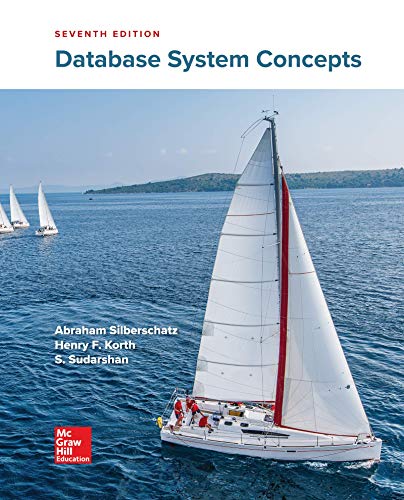
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
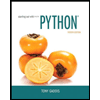
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
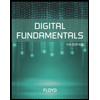
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
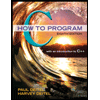
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
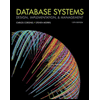
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
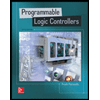
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education