Task 1: • Declare the first function as follow: 1. Return type: int. 2. Function name: bintodec (Binary to Decimal). 3. Parameter List: a string. (char *str). • Declare the second function as follow: o Return type: void. o Function name: dectobin (Decimal to Binary). o Parameter List: an int(named x), a string. (char *bin) Task 2: Define bintodec(char *str): Task 3: Each element of str is a character ('0' or '1'). Declare an int named x and initialize it as zero. To convert str to a decimal number, you can start from left of str to right. For example, to convert "00101100" to a decimal number, start at "00101100". Decrement the ASCII code of '0' to find if the number is 0 or 1. Add it to twice of x. Hint: x= (str[i] - '0') + x * 2; Continue for the next character up to the end of str. Retune x Define dectobin (int x, char *bin). In a loop, assign 0 to all 7 elements of bin. Assign '\0' to bin[7]. Repeat the following statements as far as x is not 0. The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the reminder to a character and assign the character to the appropriate element of bin. Divide x by 2 and continue the loop. • No need to return any value.
Task 1: • Declare the first function as follow: 1. Return type: int. 2. Function name: bintodec (Binary to Decimal). 3. Parameter List: a string. (char *str). • Declare the second function as follow: o Return type: void. o Function name: dectobin (Decimal to Binary). o Parameter List: an int(named x), a string. (char *bin) Task 2: Define bintodec(char *str): Task 3: Each element of str is a character ('0' or '1'). Declare an int named x and initialize it as zero. To convert str to a decimal number, you can start from left of str to right. For example, to convert "00101100" to a decimal number, start at "00101100". Decrement the ASCII code of '0' to find if the number is 0 or 1. Add it to twice of x. Hint: x= (str[i] - '0') + x * 2; Continue for the next character up to the end of str. Retune x Define dectobin (int x, char *bin). In a loop, assign 0 to all 7 elements of bin. Assign '\0' to bin[7]. Repeat the following statements as far as x is not 0. The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the reminder to a character and assign the character to the appropriate element of bin. Divide x by 2 and continue the loop. • No need to return any value.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Hello. Please answer the attached C
*If correctly fulfill and answer all of the tasks correctly, I will give you a thumbs up. Thanks.
![### Task 1:
- **Declare the first function as follows:**
1. **Return type:** int.
2. **Function name:** `bintodec` (Binary to Decimal).
3. **Parameter List:** a string. (`char *str`).
- **Declare the second function as follows:**
- **Return type:** void.
- **Function name:** `dectobin` (Decimal to Binary).
- **Parameter List:** an int (named `x`), a string. (`char *bin`).
### Task 2:
**Define `bintodec` (char *str):**
- Each element of `str` is a character ('0' or '1').
- Declare an int named `x` and initialize it as zero.
- To convert `str` to a decimal number, you can start from left of `str` to right. For example, to convert "00101100" to a decimal number, start at "00101100".
- Decrement the ASCII code of ‘0’ to find if the number is 0 or 1.
- Add it to twice of `x`. **Hint:** `x = (str[i] - '0') + x * 2`.
- Continue for the next character up to the end of `str`.
- Return `x`.
### Task 3:
**Define `dectobin` (int x, char *bin):**
- In a loop, assign 0 to all 7 elements of `bin`.
- Assign `'\0'` to `bin[7]`.
- Repeat the following statements as far as `x` is not 0.
- The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the remainder to a character and assign the character to the appropriate element of `bin`.
- Divide `x` by 2 and continue the loop.
- No need to return any value.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1223c4c6-4ebb-4911-bc0d-edb37c26385f%2F7f7045b3-2bae-4094-8136-464f9018528d%2F5q5twho_processed.png&w=3840&q=75)
Transcribed Image Text:### Task 1:
- **Declare the first function as follows:**
1. **Return type:** int.
2. **Function name:** `bintodec` (Binary to Decimal).
3. **Parameter List:** a string. (`char *str`).
- **Declare the second function as follows:**
- **Return type:** void.
- **Function name:** `dectobin` (Decimal to Binary).
- **Parameter List:** an int (named `x`), a string. (`char *bin`).
### Task 2:
**Define `bintodec` (char *str):**
- Each element of `str` is a character ('0' or '1').
- Declare an int named `x` and initialize it as zero.
- To convert `str` to a decimal number, you can start from left of `str` to right. For example, to convert "00101100" to a decimal number, start at "00101100".
- Decrement the ASCII code of ‘0’ to find if the number is 0 or 1.
- Add it to twice of `x`. **Hint:** `x = (str[i] - '0') + x * 2`.
- Continue for the next character up to the end of `str`.
- Return `x`.
### Task 3:
**Define `dectobin` (int x, char *bin):**
- In a loop, assign 0 to all 7 elements of `bin`.
- Assign `'\0'` to `bin[7]`.
- Repeat the following statements as far as `x` is not 0.
- The remainder of any number by two is either 0 or one. Add the ASCII code of zero to convert the remainder to a character and assign the character to the appropriate element of `bin`.
- Divide `x` by 2 and continue the loop.
- No need to return any value.
![### Task 4:
**Objective:**
Check the functions by the following main() function. Change `num` and `binaryNum` to check your code.
**Code:**
```c
int main()
{
int num = 12;
char binaryNum[] = "00101100";
char binaryRep[8];
int decRep;
int i, j;
dectobin(num, binaryRep);
decRep = bintodec(binaryNum);
printf(" Binary representation of %d is %s.\n", num, binaryRep);
printf(" Decimal representation of %s is %d.\n", binaryNum, decRep);
return 0;
}
```
**Explanation:**
- The `main()` function is initialized with two variables: `num` and `binaryNum`.
- `num` is an integer with a value of 12.
- `binaryNum` is a string containing the binary equivalent of a number.
- The function converts the decimal number `num` to its binary representation and stores it in `binaryRep`.
- It also converts the binary number `binaryNum` to its decimal equivalent and stores it in `decRep`.
- The results for both conversions are printed to the console.
**Testing the Code:**
**1. Decimal to Binary Conversion Tests:**
1. When `num = 12`, the output should be `0000 1100`.
2. When `num = 67`, the output should be `0100 0011`.
3. When `num = 32`, the output should be `0100 0000`.
**2. Binary to Decimal Conversion Tests:**
1. When `char binaryNum[] = "00101100"`, the output is 44.
2. When `char binaryNum[] = "01000011"`, the output should be 67.
3. When `char binaryNum[] = "01000000"`, the output should be 32.
**Graphical Explanation:**
- There are no graphs or diagrams in this text.
This example demonstrates how to test the correctness of custom functions `dectobin()` and `bintodec()` for converting between decimal and binary representations in C. By changing the values of `num` and `binaryNum`, you can verify the accuracy of these functions.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1223c4c6-4ebb-4911-bc0d-edb37c26385f%2F7f7045b3-2bae-4094-8136-464f9018528d%2Ftam8r1c_processed.png&w=3840&q=75)
Transcribed Image Text:### Task 4:
**Objective:**
Check the functions by the following main() function. Change `num` and `binaryNum` to check your code.
**Code:**
```c
int main()
{
int num = 12;
char binaryNum[] = "00101100";
char binaryRep[8];
int decRep;
int i, j;
dectobin(num, binaryRep);
decRep = bintodec(binaryNum);
printf(" Binary representation of %d is %s.\n", num, binaryRep);
printf(" Decimal representation of %s is %d.\n", binaryNum, decRep);
return 0;
}
```
**Explanation:**
- The `main()` function is initialized with two variables: `num` and `binaryNum`.
- `num` is an integer with a value of 12.
- `binaryNum` is a string containing the binary equivalent of a number.
- The function converts the decimal number `num` to its binary representation and stores it in `binaryRep`.
- It also converts the binary number `binaryNum` to its decimal equivalent and stores it in `decRep`.
- The results for both conversions are printed to the console.
**Testing the Code:**
**1. Decimal to Binary Conversion Tests:**
1. When `num = 12`, the output should be `0000 1100`.
2. When `num = 67`, the output should be `0100 0011`.
3. When `num = 32`, the output should be `0100 0000`.
**2. Binary to Decimal Conversion Tests:**
1. When `char binaryNum[] = "00101100"`, the output is 44.
2. When `char binaryNum[] = "01000011"`, the output should be 67.
3. When `char binaryNum[] = "01000000"`, the output should be 32.
**Graphical Explanation:**
- There are no graphs or diagrams in this text.
This example demonstrates how to test the correctness of custom functions `dectobin()` and `bintodec()` for converting between decimal and binary representations in C. By changing the values of `num` and `binaryNum`, you can verify the accuracy of these functions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
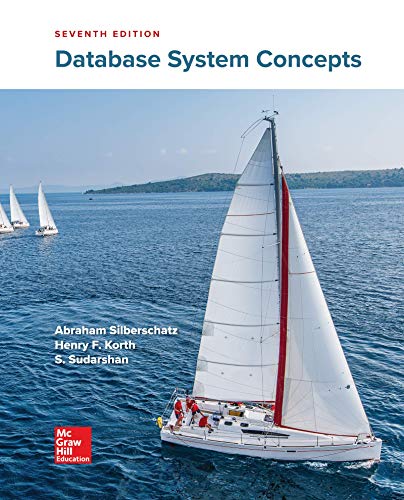
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
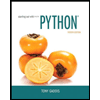
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
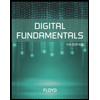
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
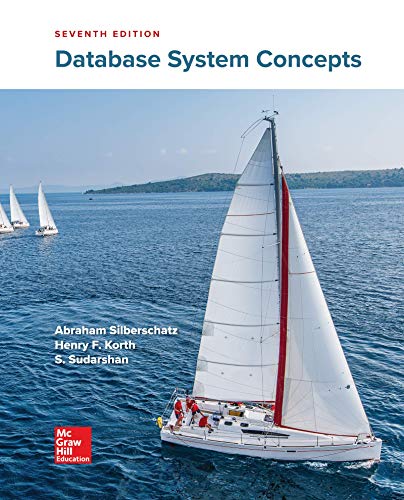
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
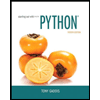
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
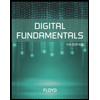
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
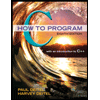
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
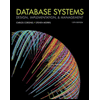
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
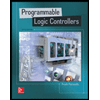
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education