t oriented programming Make code in C++ Write a pure abstract class named Token that has the following virtual functions. public: virtual float PutNumber()=0; // pure virtual functions virtual char PutOperator()=
Subject Object oriented
Make code in C++
Write a pure abstract class named Token that has the following virtual functions.
public:
virtual float PutNumber()=0; // pure virtual functions
virtual char PutOperator()=0;
Derive a class named Operator from Token class that has following member variables and functions.
private:
char oper; // operators +, -, *, /
public:
Operator(char); // constructor sets value
char PutOperator(); // displays character operator
float PutNumber();// dummy function, does nothing
Derive a class named Number from Token class that has following member variables and functions.
private:
float fnum; // the number
public:
Number(float); // constructor sets value
float PutNumber(); // displays number
char PutOperator(); // dummy function, does nothing
Next Write a class named Stack that has the following member variables and functions.
Variables : int Top, Token *array[2];
Functions: A constructor to initialize the variables.
A Push function to push a Token object in to the stack.
A Pop function to return a Token object from the stack.
Write a main function that will declare pointer array of type Token having size 2 and initialize the array with the objects of type Operator and Number respectively. Then declare an object of Stack class and call a push function to push object of type Token holding Operator object on to the stack. Then call a pop function to return object of type Token and call PutOperator function to display the operator.
Similarly push object of type Token holding Number object on to the stack. Then call a pop function to return object of type Token and call PutNumber function to display the number.
Note: Do not change the names of functions and variables. Otherwise, not acceptabe. Do not define extra functions except for constructors.

Step by step
Solved in 2 steps

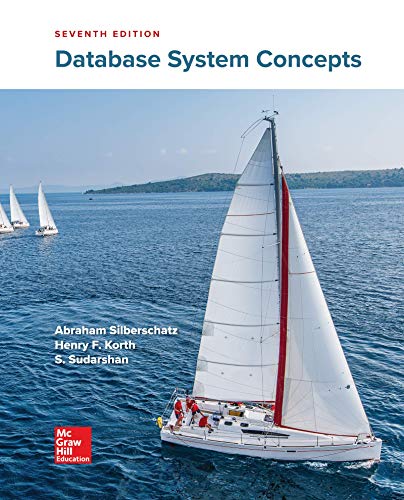
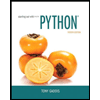
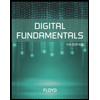
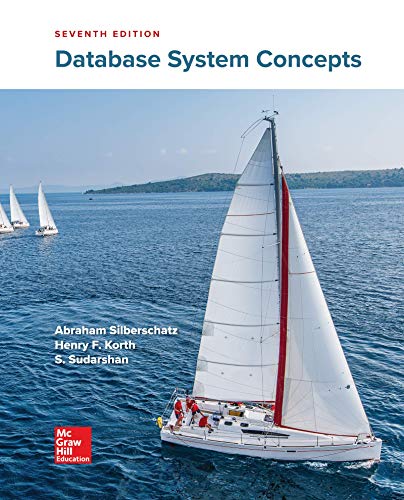
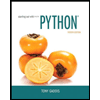
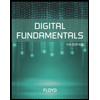
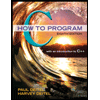
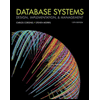
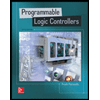