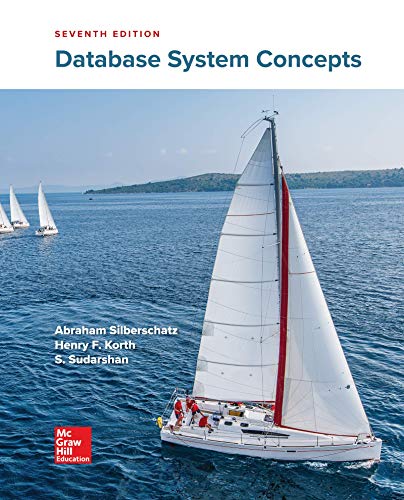
Suppose you have a list of key/value pairs (i.e., a nested list where each element of the list is a (key value)pair. For example, the list might look like this: '((France Paris) (France Nice) (Spain Madrid) (Poland Krakow) (Poland Warsaw)) (Notice that the keys need not be unique for this problem.)
Write a function named first-occurrence in Scheme that takes a key and a list then returns the value of the first item on the list with a matching key. Return '() if none of items on the list has a matching key.
You must use either fold or reduce to do the vast majority of the work. You may use the built-in foldmethod by adding the line (require 'list-lib), or you can use your own implementation.
The built-in fold function has parameters in this order: action partial-result lst.
You may assume that the list contains an element with the key to be updated.
For example,
- (first-occurrence 'Germany '((France Paris) (Germany Bonn) (Germany Berlin))) should return 'Bonn
- (first-occurrence 'Poland '((France Paris) (Germany Bonn) (Germany Berlin))) should return '()
- (first-occurrence 'Poland '()) should return '()
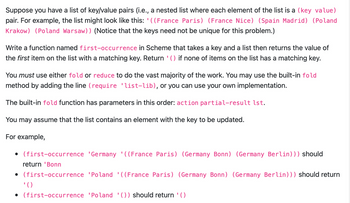

Step by stepSolved in 3 steps

- Program in Carrow_forwardin #lang schemearrow_forwardWrite a function definition named first_and_second that takes in sequence and returns the first and second value of that sequence as a list. This is in python Also assert, assert first_and_second([1, 2, 3, 4]) == [1, 2] assert first_and_second(["python", "is", "awesome"]) == ["python", "is"] assert first_and_second(["strawberry", "kiwi", "mango", "guava"]) == ["strawberry", "kiwi"]arrow_forward
- Write a function two in Scheme that takes a single parameter, a list of integers. The function tworeturns #t if the list of integers includes two equal integers. Otherwise, it returns #f. The list L isflat, i.e., it does not contain sublists.arrow_forwardI need the answer as soon as possiblearrow_forwardPractice recursion on lists Practice multiple base conditions Combine recursion call with and,or not operators Search for an element in a list using recursion Instructions In this lab, we will write a recursive function for searching a list of integers/strings. Write a recursive function to determine if a list contains an element. Name the function recursive_search(aList, value), where aList is a list and value if primitive type is the object we want to search for. Return a boolean, specifically True if and only if value is an element of aList else return False from the function Examples: recursive_search([1,2,3], 2) == True recursive_search([1,2,3], 4) == False recursive_search([ ], 4) == False recursive_search([ [ 1 ], 2 ], 1) == False # -----> (because the list contains [ 1 ] and 2, not 1) Hint: Think about what the base case is? When is it obvious that the element is not in the list? If we are not at the base case, how can you use the information about the first element of the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
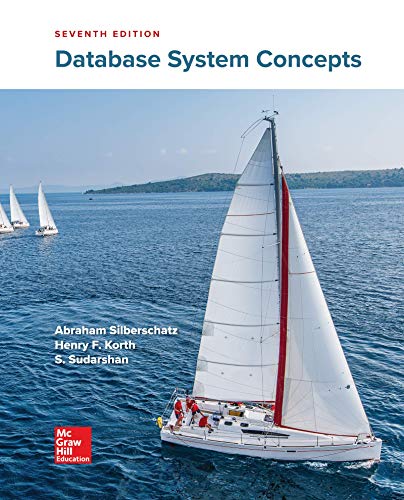
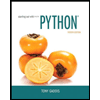
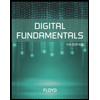
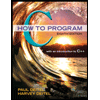
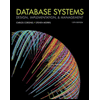
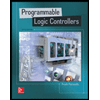