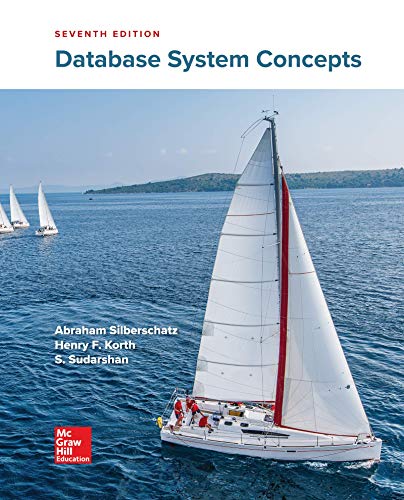
Suppose an array sorted in ascending order is rotated at some pivot unknown
to you beforehand. (i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).
Find the minimum element. The complexity must be O(logN)
You may assume no duplicate exists in the array.
"""
def find_min_rotate(array):
"""
Finds the minimum element in a sorted array that has been rotated.
"""
low = 0
high = len(array) - 1
while low < high:
mid = (low + high) // 2
if array[mid] > array[high]:
low = mid + 1
else:
high = mid
return array[low]
def find_min_rotate_recur(array, low, high):
"""
Finds the minimum element in a sorted array that has been rotated.
"""
mid = (low + high) // 2
if mid == low:.

Step by stepSolved in 5 steps with 2 images

- Suppose an array sorted in ascending order is rotated at some pivot unknownto you beforehand. (i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).Find the minimum element. The complexity must be O(logN)You may assume no duplicate exists in the array."""def find_min_rotate(array): """ Finds the minimum element in a sorted array that has been rotated. """ low = 0 high = len(array) - 1 while low < high: mid = (low + high) // 2 if array[mid] > array[high]: low = mid + 1 else: high = mid return array[low] def find_min_rotate_recur(array, low, high): """ Finds the minimum element in a sorted array that has been rotated. """ mid = (low + high) // 2 if mid == low:.arrow_forwardDESIGN YOUR OWN SETTING Task: Devise your own setting for storing and searching the data in an array of non-negative integers redundantly. You may just describe the setting without having to give an explicit algorithm to explain the process by which data is stored. You should explain how hardware failures can be detected in your method. Once you have described the setting, include the following to your answer: Write a pseudocode function to describe an algorithm where the stored data can be searched for a value key: if the data is found, its location in the original array should be returned; -1 should be returned if the data is not found; -2 should be returned if there is a data storage error Include a short commentary explaining why your pseudocode works Describe the worst-case and best-case inputs to your search algorithm Derive the worst-case and best-case running times for the search algorithm Derive the Theta notation for the worst-case and best-case running timesarrow_forwardProblem2: A square matrix can be represented by a two-dimensionalarray with N rows and N columns. You may assume a maximum size of 50 rows and 50 columns. 1. Write an algorithm MakeEmpty(n), which sets the first n rows and n columns to zero. 2. Write an algorithm Add(M1, M2, M3), which adds two matrices M1 and M2 together to produce matrix M3. 3. Write an algorithm Subtract(M1, M2, M3), which subtracts matrix M2 from matrix M1 to produce matrix M3. 4. Write an algorithm Copy(M1, M2), which copies matrix M1 into matrix M2.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
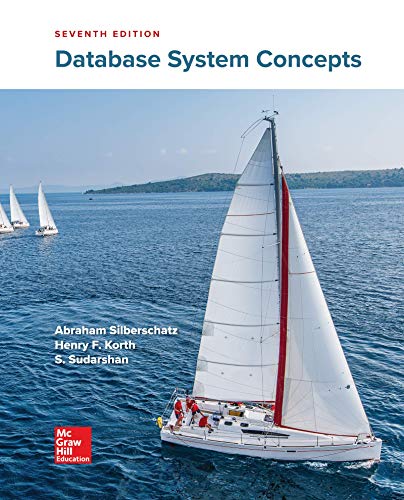
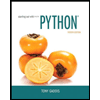
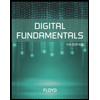
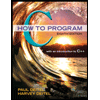
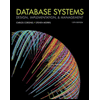
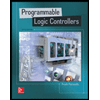