Step 1 – Make the GPA calculations work Your job is to process the grade and units information when the user clicks the ADD or Reset buttons and to compute the GPA when the user clicks the GPA button. There are five (5) places in the given code for you to add your code. Each of these is commented with a brief description of your task followed by the phrase “Your code here (n of 5)….”. • The variable totalUnits stores the total credits of all classes taken. • The variable gradePoints stores the total grade points earned in those classes taken. For example, if you got a B in a 4 credit hour course, you earn 3.0 x 4 = 12.0 grade points. • The variable totalGPA stores the total GPA, which is simply the total grade points earned divided by the total units taken. For example, if you got an A in a 3 credit course, a C in a 5 credit course, and a B in a 4 credit course, you have (4.0 x 3) + (2.0 x 5) + (3.0 x 4) = 34.0 grade points and 3 + 5 + 4 = 12 total units, so the GPA is 34.0 / 12 = 2.833. Step 2 – Add exception handling You may notice that when you accidentally enter a non-number in the units field, it crashes your program. Instead of crashing add exception handling (using a try-catch block) to catch the problem. The error message tells you which statement causes the exception. Then you can wrap all the code related to that task (updating the points and units running totals) in a try-catch block. Use a JOptionPane.showMessageDialog(…) to report the error.
Step 1 – Make the GPA calculations work Your job is to process the grade and units information when the user clicks the ADD or Reset buttons and to compute the GPA when the user clicks the GPA button. There are five (5) places in the given code for you to add your code. Each of these is commented with a brief description of your task followed by the phrase “Your code here (n of 5)….”. • The variable totalUnits stores the total credits of all classes taken. • The variable gradePoints stores the total grade points earned in those classes taken. For example, if you got a B in a 4 credit hour course, you earn 3.0 x 4 = 12.0 grade points. • The variable totalGPA stores the total GPA, which is simply the total grade points earned divided by the total units taken. For example, if you got an A in a 3 credit course, a C in a 5 credit course, and a B in a 4 credit course, you have (4.0 x 3) + (2.0 x 5) + (3.0 x 4) = 34.0 grade points and 3 + 5 + 4 = 12 total units, so the GPA is 34.0 / 12 = 2.833. Step 2 – Add exception handling You may notice that when you accidentally enter a non-number in the units field, it crashes your program. Instead of crashing add exception handling (using a try-catch block) to catch the problem. The error message tells you which statement causes the exception. Then you can wrap all the code related to that task (updating the points and units running totals) in a try-catch block. Use a JOptionPane.showMessageDialog(…) to report the error.



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

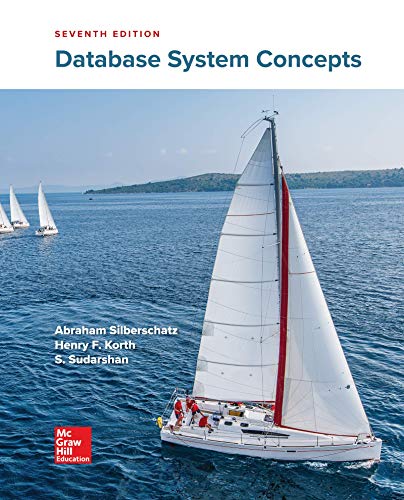
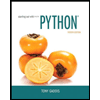
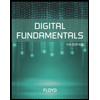
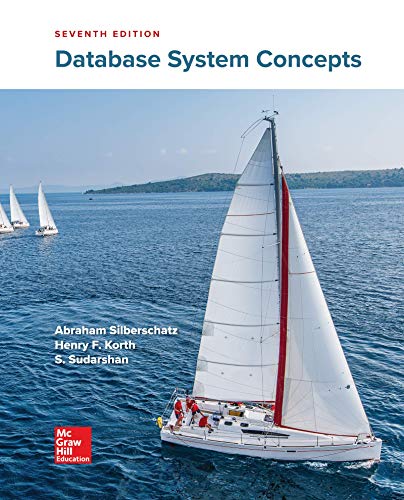
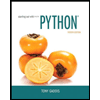
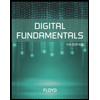
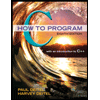
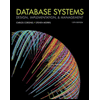
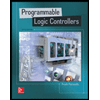