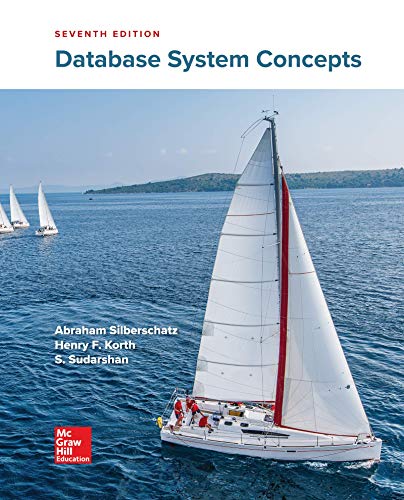
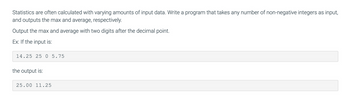

Algorithm:
1. Start
2. Create a function called calculate_statistics() that takes in a list of numbers as an argument.
3. Create variables called max_value and avg_value to store the maximum value and average value of the list.
4. Set max_value to the maximum value in the list.
5. Set avg_value to the sum of the list divided by the length of the list.
6. Return a tuple with both max_value and avg_value rounded to 2 decimal places.
7. Create a main() function.
8. Inside main(), take in user input and store it as a list of float values.
9. Call calculate_statistics() and pass in the list of float values as an argument.
10. Store the returned values in variables called max_value and avg_value.
11. Print the max_value and avg_value variables with two decimal places.
12. Stop
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- In many sports events, contestants are rated by judges with an average score being determined by discarding the highest and lowest scores and averaging the remaining scores. Write a program in which eight scores are entered, computing the average score for the contestants.arrow_forwardWrite a program that takes in an integer in the range 11-100 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Ex: If the input is: 93 the output is: 93 92 91 90 89 88 Ex: If the input is: 11 the output is: 11 Ex: If the input is: 9 or any value not between 11 and 100 (inclusive), the output is: Input must be 11-100 Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, ..., 99), as that approach would be cumbersome for larger ranges.arrow_forwardYou are working on a ticketing system. A ticket costs $10. The office is running a discount campaign: each group of 5 people is getting a discount, which is determined by the age of the youngest person in the group. You need to create a program that takes the ages of all 5 people as input and outputs the total price of the tickets. Sample Input: 55 28 15 38 63 Sample Output: 42.5 The youngest age is 15, so the group gets a 15% discount from the total price, which is $50 - 15% = $42.5 The given code is declaring an array of 5 elements and taking them from input using a loop.arrow_forward
- Statistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the average (using integer division) and max. A negative integer ends the input and is not included in the statistics. Ex: When the input is: 15 20 0 5 -1 the output is: 10 20 Assume that at least one non-negative integer is input.arrow_forwardStatistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the max and average. A negative integer ends the input and is not included in the statistics. Assume the input contains at least one non-negative integer. Output the average with two digits after the decimal point followed by a newline, which can be achieved as follows: System.out.printf("%.2f\n", average); Ex: When the input is: 15 20 0 3 -1 the output is: 20 9.50 365076.2342078.qx3zgy7 LAB 20.15.1: LAB: Varied amount of input data 0/ 10 АCTIVITY LabProgram.java Load default template... 1 import java.util.Scanner; 2 3 public class LabProgram { public static void main(String] args) { /* Type your code here. */ } 5 7 } 8arrow_forwarduse java programingarrow_forward
- 24. Write a program which asks the user for an integer number and prints out whether the input number is a multiple of 7. A. Example 1 i. Input: • ii. Output: B. Example 2 i. Input: Please enter number: 9 ii. Output: Number is not a multiple of seven 25. Write a program which asks the user for two integer numbers (say N1 and N2) and prints out whether the input number NI is a multiple of N2. A. Example 1 i. Input: • B. Example 2 ii. Output: • i. Input: A. Example Please enter number: 49 Number is a multiple of seven i. Input: ii. Output: Number 40 is not a multiple of 3 26. Write a program which asks the user for an integer number and prints out whether the input number is even or odd. Please enter first number: 49 Please enter second number: 7 Number 49 is a multiple of 7 Please enter first number: 40 Please enter second number: 3 • Please enter number: 42 i. Input ii. Output: Number is even 27. Write a program that asks the user for an integer number and prints a pattern. A. Example…arrow_forwardWrite a program that calculates the total grade for N classroom exercises as a percentage. The user should input the value for N followed by each of the N scores and totals. Calculate the overall percentage (sum of the total points earned divided by the total points possible) and output it as a percentage. Sample input and output is shown below. How many exercises to input? 3 Score received for exercise 1: 10 Total points possible for exercise 1: 10 Score received for exercise 2: 7 Total points possible for exercise 2: 12 Score received for exercise 3: 5 Total points possible for exercise 3: 8 Your total is 22 out of 30, or 73.33%.arrow_forwardWrite a program to calculate the net pay of an employee. Input the basic pay and calculate the net pay as follows: House rent is 45% of basic pay. Medical allowance is 2% of basic pay if basic pay is greater than Rs. 5000/-. It is 5% of basic pay if the basic pay is less than Rs. 5000/-. Conveyance allowance is Rs. 96/- if basic pay is less than Rs. 5000/-. It is Rs. 193/- if the basic pay is more than Rs. 5000/- Net pay is calculated by adding basic pay, medical allowance, conveyance allowance and house rant. using ooparrow_forward
- Statistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the max and average, respectively. Output the max and average with two digits after the decimal point. Ex: If the input is: 14.25 25 0 5.75 the output is: 25.00 11.25 461710 3116374.qx3zqy7 LAB ACTIVITY 6.7.1: LAB: Varied amount of input data 0/10arrow_forwardA palindromic number is a number that is the same when written forwards or backwards. The first few palindromic numbers are therefore are: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 11, 22, 33, 44, 55, 66, 77, 88, 99, 101, 111, 121, 323 Input a number from the user. Write a program that reverses the digits of a number. Use this program to find if the number is a palindrome. Print if the number is palindrome or not.arrow_forwardThe following program prints out several numbers. What is the first and what is the last number it prints? You should be able to answer the question without running the program. number = 2 numbers = [0, 1, 2, 3, 4] for number in numbers: print(number) print (number) The first number it prints is the last number it prints is Checkarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
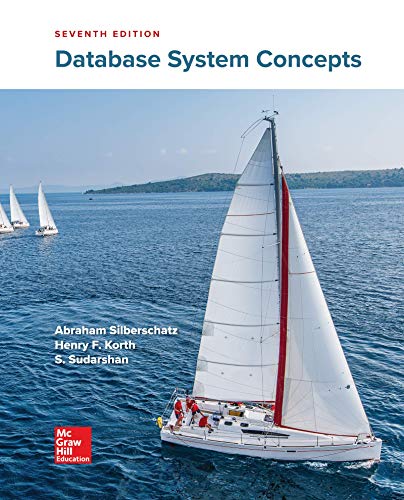
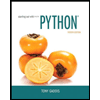
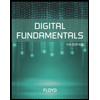
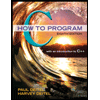
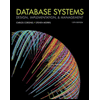
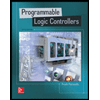