solve this question using java You must define a class named MediaRental that implements the MediaRentalInt interface functionality (index A). You must define classes that support the functionality specified by the interface: . Define a class named MediaRental. Feel free to add any instance variables you understand are needed or any private methods. Do not add any public methods (beyond the ones specified in the MediaRentalInt interface). . The media rental system keeps track of customers and media (movies ,music albums and games). A customer has a name, address as string , a plan and two lists. One list represent the media the customer is interested in receiving and the second one represents the media already received (rented) by the customer. There are two plans a customer can have: UNLIMITED and LIMITED. UNLIMITED allows a customer to receive as many media as they want; LIMITED restricts the media to a default value of 2 (this value can be change via a media rental class method). A movie has a title, a number of copies available and a rating (e.g., "HR"). An album has a title, number of copies available, an artist and the songs that are part of the album. A game has title,number of copies,and the weight (in grams) is also stored. . You must define and use at least four classes (not including MediaRental) as part of your design. At least two of those classes must be in a superclass/subclass relationship (Inheritance Requirement). The other two can be defined as you wish. Feel free to define as many classes as you want. These classes must support the functionality of the system otherwise you will not receive any credit. . One of your classes must define an equals method that has as parameter an Object parameter. . The database for your system needs to be represented using two ArrayList objects. One ArrayList will represent the customers present in the database; the second will represent the media (movies, albums , and games). . Regarding the searchMedia method: the songs parameter represents a substring (fragment) or the full list of songs associated with the album. If the full list is provided you can assume commas will be part of the string. Hint: you may want to consider using the indexOf method of the String class. 7. Your program should store the results in a file between executions of the program, so that when the program is run again it will start up with the same inventory contents as when it last terminated. . free to use Collections.sort to sort your data. . Write test driver. . Handle expectations where it’s needed. .Not all the details associated with the project can be fully specified in this description. The sooner you start working on the project the sooner you will be able to address any doubts you may have. Index A in the second picture.(d3) index B: Index B: Public Driver: Method Detail public void testAddingCustomers() public void testAddingMedia() public void testingAddingToCart() public void testingRemovingFromCart() public void testProcessingRequestsOne() public void testProcessingRequestsTwo() public void testReturnMedia() public void testSearchMedia()
solve this question using java
You must define a class named MediaRental that implements the MediaRentalInt interface functionality
(index A). You must define classes that support the functionality specified by the interface:
. Define a class named MediaRental. Feel free to add any instance variables you understand are needed or any private methods. Do not add any public methods (beyond the ones specified in the MediaRentalInt interface).
. The media rental system keeps track of customers and media (movies ,music albums and games). A customer
has a name, address as string , a plan and two lists. One list represent the media the customer is interested in
receiving and the second one represents the media already received (rented) by the customer. There are two
plans a customer can have: UNLIMITED and LIMITED. UNLIMITED allows a customer to receive as many media
as they want; LIMITED restricts the media to a default value of 2 (this value can be change via a media rental
class method).
A movie has a title, a number of copies available and a rating (e.g., "HR"). An album has a title, number of
copies available, an artist and the songs that are part of the album. A game has title,number of copies,and the
weight (in grams) is also stored.
. You must define and use at least four classes (not including MediaRental) as part of your design. At least two of
those classes must be in a superclass/subclass relationship (Inheritance Requirement). The other two can be
defined as you wish. Feel free to define as many classes as you want. These classes must support the
functionality of the system otherwise you will not receive any credit.
. One of your classes must define an equals method that has as parameter an Object parameter.
. The
the customers present in the database; the second will represent the media (movies, albums , and games).
. Regarding the searchMedia method: the songs parameter represents a substring (fragment) or the full list of
songs associated with the album. If the full list is provided you can assume commas will be part of the string.
Hint: you may want to consider using the indexOf method of the String class.
7. Your program should store the results in a file between executions of the program, so
that when the program is run again it will start up with the same inventory contents as
when it last terminated.
. free to use Collections.sort to sort your data.
. Write test driver.
. Handle expectations where it’s needed.
.Not all the details associated with the project can be fully specified in this description. The sooner you start
working on the project the sooner you will be able to address any doubts you may have.
Index A
in the second picture.(d3)
index B:
Index B:
Public Driver:
Method Detail
public void testAddingCustomers()
public void testAddingMedia()
public void testingAddingToCart()
public void testingRemovingFromCart()
public void testProcessingRequestsOne()
public void testProcessingRequestsTwo()
public void testReturnMedia()
public void testSearchMedia()



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

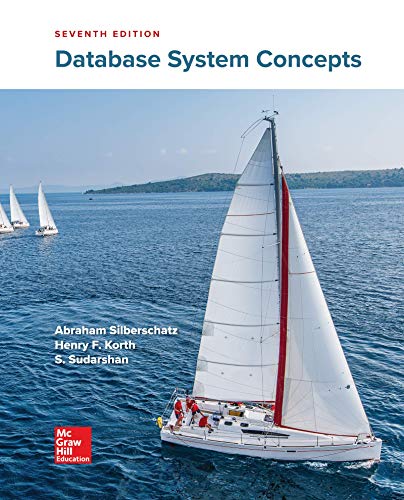
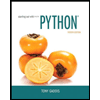
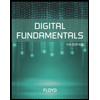
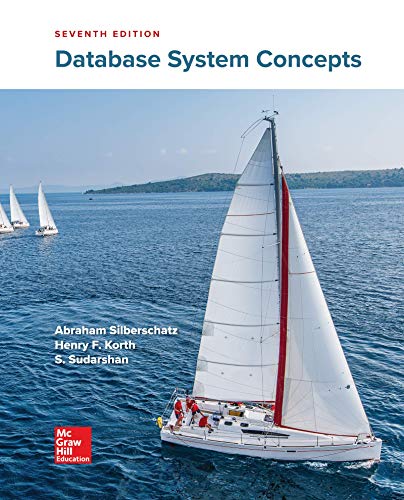
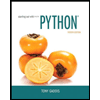
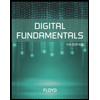
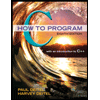
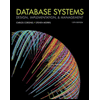
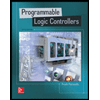